- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
- Subtract Two Numbers in Python
- Python Program to Check Whether a Number is Positive or Negative or zero
- Python program to Represent Negative Integers in binary format
- How to Get a Negation of a Boolean in Python
- Python program to concatenate two Integer values into one
- Shared Reference in Python
- Python | Accessing variable value from code scope
- Python program to create dynamically named variables from user input
- Python Program to Calculate the Area of a Triangle
- How to Take Multiple Inputs Using Loop in Python
- Perfect Number Program in Python
- Get Length of a List in Python Without Using Len()
- Program to Convert Fahrenheit into Celsius
- Append Tuple To A Set With Tuples
- Get User Input in Loop using Python
- Divide Two Integers to get Float in Python
- Python Program to Calculate the Area of a Trapezoid
- How to change any data type into a String in Python?
- Access the Index and Value using Python 'For' Loop

Intermediate Coding Problems in Python
Python, being a very dynamic and versatile programming language, is used in almost every field. From software development to machine learning, it covers them all. This article will focus on some interesting coding problems which can be used to sharpen our skills a bit more and at the same time, have fun solving this list of specially curated problems. Although this article will focus on solving these problems using Python, one can feel free to use any other language of their choice. So let’s head right into it!
Infinite Monkey Theorem
The theorem states that a monkey hitting keys at random on a typewriter keyboard for an infinite amount of time will almost surely type a given text, such as the complete works of William Shakespeare. Well, suppose we replace a monkey with a Python function. How long would it take for a Python function to generate just one sentence? The sentence we will go for is: “a computer science portal for geeks”. The way we will simulate this is to write a function that generates a string that is 35 characters long by choosing random letters from the 26 letters in the alphabet plus space. We will write another function that will score each generated string by comparing the randomly generated string to the goal. A third function will repeatedly call generate and score, then if 100% of the letters are correct we are done. If the letters are not correct then we will generate a whole new string. To make it easier to follow, our program should keep track of the best string generated so far.
Example:
Here, we wrote three functions. One will generate a random string using the 26 characters of the alphabet and space. The second function will then score the generated string by comparing each letter of it with the goalString. The third function will repeatedly call the first two functions until the task is completed. It will also take note of the best string generated so far by comparing their scores. The one with the highest score will be the best one. Finally, we run this program in our IDE and see it work.
The substring dilemma
This is a really interesting program as it generates some really funny outputs. It is also a healthy practice problem for beginners who want to understand the “string” type more clearly. Let’s look into the problem. Given a string, find a substring based on the following conditions:
- The substring must be the longest one of all the possible substring in the given string.
- There must not be any repeating characters in the substring.
- If there is more than one substring satisfying the above two conditions, then print the substring which occurs first.
- If there is no substring satisfying all the aforementioned conditions then print -1.
Although there can be many methods of approach to this problem, we will look at the most basic one.
Here, we write a single function that will carry out the entire task. First it will initialize variables called substring and testList to store the substring and a list of possible outputs respectively. Then it will loop over the entire string provided and break every time it finds a repetition and appends that word to testList. Finally, the longest word out of the possible outputs is returned.
Output:
A low-level implementation of the classic game “Mastermind”. We need to write a program that generates a four-digit random code and the user needs to guess the code in 10 tries or less. If any digit out of the guessed four-digit code is wrong, the computer should print out “B”. If the digit is correct but at the wrong place, the computer should print “Y”. If both the digit and position is correct, the computer should print “R”. Example:
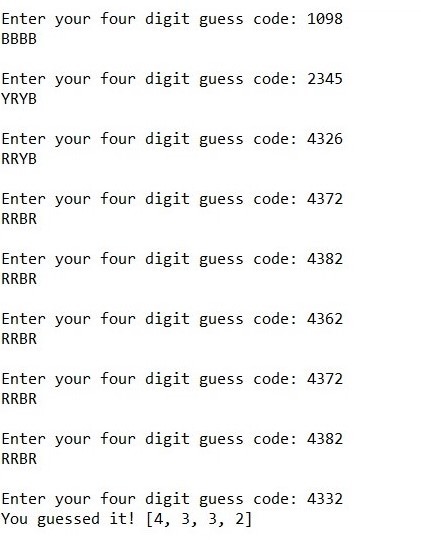
First, we write a function to generate a random four-digit code using Python’s random module. Next, we define a function that asks for user input. Finally, we write a function that compares the generated code to the guessed code and gives appropriate results.
Direction Catastrophe
A very simple problem with many different solutions, but the main aim is to solve it in the most efficient way. A man was given directions to go from point A to point B. The directions were: “SOUTH”, “NORTH”, “WEST”, “EAST”. Clearly “NORTH” and “SOUTH” are opposite, “WEST” and “EAST” too. Going to one direction and coming back in the opposite direction is a waste of time and energy. So, we need to help the man by writing a program that will eliminate the useless steps and will contain only the necessary directions. For example: The directions [“NORTH”, “SOUTH”, “SOUTH”, “EAST”, “WEST”, “NORTH”, “WEST”] should be reduced to [“WEST”]. This is because going “NORTH” and then immediately “SOUTH” means coming back to the same place. So we cancel them and we have [“SOUTH”, “EAST”, “WEST”, “NORTH”, “WEST”]. Next, we go “SOUTH”, take “EAST” and then immediately take “WEST”, which again means coming back to the same point. Hence we cancel “EAST” and “WEST” to giving us [“SOUTH”, “NORTH”, “WEST”]. It’s clear that “SOUTH” and “NORTH” are opposites hence canceled and finally we are left with [“WEST”].
In the above solution, we create a dictionary of opposites to help us determine if a given direction is opposite to the other. Next, we initialize a variable called finalDirections which will be our output. If the direction which is in the givenDirections is opposite of the last element in finalDirections, we pop it out of finalDirections otherwise we append it to finalDirections.
Comparing arrays
This problem helps one to understand the key concepts of an array(list) in Python. Two arrays are said to be the same if they contain the same elements and in the same order. However, in this problem, we will compare two arrays to see if they are same, but with a slight twist. Here, two arrays are the same if the elements of one array are squares of elements of other arrays and regardless of the order. Consider two arrays a and b .
a = [121, 144, 19, 161, 19, 144, 19, 11] b = [121, 14641, 20736, 361, 25921, 361, 20736, 361]
Here b can be written as:
b = [11*11, 121*121, 144*144, 19*19, 161*161, 19*19, 144*144, 19*19]
which is a square of every element of a . Hence, they are same. If a or b are None, our program should written False

Please Login to comment...
Similar reads.
- python-basics
- Python Programs
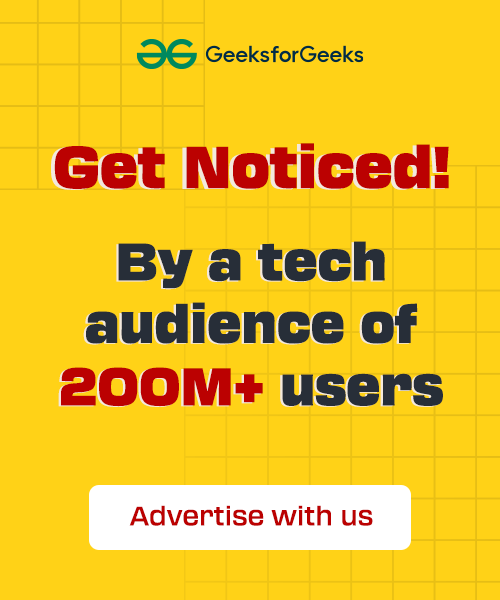
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- How it works
- Homework answers
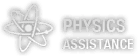
Answer to Question #332118 in Python for sandhya
Dice Score:
two friends are playing a dice game, the game is played with five six-sided dice. scores for each type of throws are mentioned below.
three 1's = 1000 points
three 6's = 600
three 5's = 500
three 4's = 400
three 3's = 300
three 2's = 200
one 1 = 100
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !
Leave a comment
Ask your question, related questions.
- 1. Number of moves:you are given a nxn square chessboard with one bishop and k number of obstacles plac
- 2. Ash is now an expert in python function topic. So, he decides to teach others what he knows by makin
- 3. def countdown(n): if n <= 0: print('Blastoff!') else: print
- 4. Create a program that asks the user to input 5 numbers. The numbers should be stored in a list, afte
- 5. Variables, python operators,data types,string slicing, range of string using list, python conditiona
- 6. Create a program the user will input 5 grades (choose any subjects and input any grades). Determine
- 7. Write a function in this file called nine_lines that uses the function three_lines (provided below)
- Programming
- Engineering
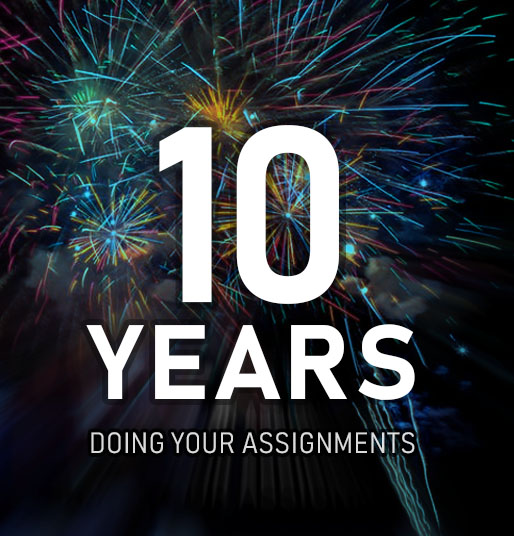

IMAGES
VIDEO
COMMENTS
ALL Answered. Question #350996. Python. Create a method named check_angles. The sum of a triangle's three angles should return True if the sum is equal to 180, and False otherwise. The method should print whether the angles belong to a triangle or not. 11.1 Write methods to verify if the triangle is an acute triangle or obtuse triangle.
The Assignment Problem is a special type of Linear Programming Problem based on the following assumptions: However, solving this task for increasing number of jobs and/or resources calls for…
With our help, you can master Python programming and tackle any assignment with confidence. In conclusion, mastering Python programming requires dedication, practice, and expert guidance.
For this problem, you'll need to parse a log file with a specified format and generate a report: Log Parser ( logparse.py) Accepts a filename on the command line. The file is a Linux-like log file from a system you are debugging. Mixed in among the various statements are messages indicating the state of the device.
These free exercises are nothing but Python assignments for the practice where you need to solve different programs and challenges. All exercises are tested on Python 3. Each exercise has 10-20 Questions. The solution is provided for every question. These Python programming exercises are suitable for all Python developers.
Are you facing a daunting Python assignment and searching for someone to help you out? Look no further! At ProgrammingHomeworkHelp.com, we specialize in offering top-notch assistance with Python…
We need to write a program that generates a four-digit random code and the user needs to guess the code in 10 tries or less. If any digit out of the guessed four-digit code is wrong, the computer should print out "B". If the digit is correct but at the wrong place, the computer should print "Y".
The Hungarian method is a combinatorial optimization algorithm that solves the assignment problem in polynomial time and which anticipated later primal-dual methods. It was developed and published ...
Python. Question #332118. Dice Score: two friends are playing a dice game, the game is played with five six-sided dice. scores for each type of throws are mentioned below. three 1's = 1000 points. three 6's = 600. three 5's = 500. three 4's = 400. three 3's = 300.
This video tutorial illustrates how you can solve the Assignment Problem (AP) using the Hungarian Method in Python
Read writing from Python Assignment and Solutions on Medium. Every day, Python Assignment and Solutions and thousands of other voices read, write, and share important stories on Medium. ... #find the sum of all digits in given number ''' Understanding problem #1591 =1+5+9+1 =16 ''' #Python code num=1591 add=0 while(num>0): #learnskill-online ...
Python programming assignments are designed to test the programming skills of students to solve a specific problem. Often times, Python assignments are very challenging and are hard to solve. If you are a student and want to solve your Python assignments easily, here are 7 tips to ace python assignment.
These experts possess extensive knowledge and practical insights into Python programming, offering personalized advice and strategies to help you overcome challenges and accelerate your learning ...
medium problem 1 python assignment expert Based on the search results, it seems that there is a question related to a medium-level problem in Python for an assignment. Unfortunately, the specific details of the problem are not provided in the search results.
Read stories about Python Assignment Experts on Medium. Discover smart, unique perspectives on Python Assignment Experts and the topics that matter most to you like Python Assignment Help, Python ...
The latest way to get help from an expert for your practice problems or python assignment is by hiring Homework Help Solutions. We are a team of experts in respective fields, brings solutions to the…
1. AimThe purpose of this assignment is to:• design and implement the solution to a program in the form of a medium sized Python program• practice the use of arithmetic computations, tests, repetitions, lists, and strings • use procedural programming.2. DescriptionYou will design and implement a program that prompts the user for ...
Statistics Assignment Help has been my academic savior! The intricate world of statistical analysis and hypothesis testing became more manageable with their expert guidance.
Python programming assignments are designed to test the programming skills of students to solve a specific problem. Often times, Python assignments are very hard to solve. If you are a student and want to solve your Python assignments easily, here are 7 tips to ace python assignment. Read the instructions carefully:
Images. Videos. News
Why HelpwithAssignment.com is the best for Python programming assignment help. Customized Solutions: We can't use the same type of solution for all the tasks. Our panel of Python assignment experts go thoroughly through the instructions given by your professor and delivers exactly what is being asked for. 24X7 Customer Care Support: It might ...