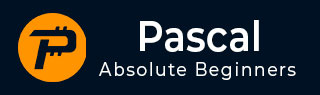
- Pascal Tutorial
- Pascal - Home
- Pascal - Overview
- Pascal - Environment Setup
- Pascal - Program Structure
- Pascal - Basic Syntax
- Pascal - Data Types
- Pascal - Variable Types
- Pascal - Constants

Pascal - Operators
- Pascal - Decision Making
- Pascal - Loops
- Pascal - Functions
- Pascal - Procedures
- Pascal - Variable Scope
- Pascal - Strings
- Pascal - Booleans
- Pascal - Arrays
- Pascal - Pointers
- Pascal - Records
- Pascal - Variants
- Pascal - Sets
- Pascal - File Handling
- Pascal - Memory
- Pascal - Units
- Pascal - Date & Time
- Pascal - Objects
- Pascal - Classes
- Pascal Useful Resources
- Pascal - Quick Guide
- Pascal - Useful Resources
- Pascal - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
An operator is a symbol that tells the compiler to perform specific mathematical or logical manipulations. Pascal allows the following types of operators −
- Arithmetic operators
- Relational operators
- Boolean operators
- Bit operators
- Set operators
- String operators
Let us discuss the arithmetic, relational, Boolean and bit operators one by one. We will discuss the set operators and string operations later.
Arithmetic Operators
Following table shows all the arithmetic operators supported by Pascal. Assume variable A holds 10 and variable B holds 20, then −
Show Examples
Relational Operators
Following table shows all the relational operators supported by Pascal. Assume variable A holds 10 and variable B holds 20, then −
Boolean Operators
Following table shows all the Boolean operators supported by Pascal language. All these operators work on Boolean operands and produce Boolean results. Assume variable A holds true and variable B holds false, then −
Bit Operators
Bitwise operators work on bits and perform bit-by-bit operation. All these operators work on integer operands and produces integer results. The truth table for bitwise and (&), bitwise or (|), and bitwise not (~) are as follows −
Assume if A = 60; and B = 13; now in binary format they will be as follows −
A = 0011 1100
B = 0000 1101
-----------------
A&B = 0000 1100
A^B = 0011 0001
~A = 1100 0011
The Bitwise operators supported by Pascal are listed in the following table. Assume variable A holds 60 and variable B holds 13, then:
Please note that different implementations of Pascal differ in bitwise operators. Free Pascal, the compiler we used here, however, supports the following bitwise operators −
Operators Precedence in Pascal
Operator precedence determines the grouping of terms in an expression. This affects how an expression is evaluated. Certain operators have higher precedence than others; for example, the multiplication operator has higher precedence than the addition operator.
For example x = 7 + 3 * 2; here, x is assigned 13, not 20 because operator * has higher precedence than +, so it first gets multiplied with 3*2 and then adds into 7.
Here, operators with the highest precedence appear at the top of the table, those with the lowest appear at the bottom. Within an expression, higher precedence operators will be evaluated first.
Data Type Assignments and Compatibility
Table 4-1 data type assignment type of variable/parameter type of assignment-compatible expression real , single , shortreal real , single , shortreal , double , longreal , any integer type double , longreal real , single , shortreal , double , longreal , any integer type integer , integer16 , integer32 integer , integer16 , integer32 boolean boolean char char enumerated same enumerated type subrange base type of the subrange record record of the same type array array with the same type set set with compatible base type pointer pointer to an identical type, univ_ptr, string assignments, fixed- and variable-length strings, table 4-2 fixed- and variable-length string assignments type of string type of assignment-compatible expression array of char varying string, constant string, and array of char if the arrays have the same length varying varying string, constant string, array of char , and char, null strings, table 4-3 null string assignments assignment description varying := ''; the compiler assigns the null string to the variable-length string. the length of the variable-length string equals zero. array of char := ''; the compiler assigns a string of blanks to the character array. the length of the resulting string is the number of elements in the source character array. char := ''; it is illegal to assign a null string to a char variable. use chr(0) instead. string concatenation in a string concatenation expression such as: s := 'hello' + '' + s; '' is treated as the additive identity (as nothing)., string constants, arithmetic operators, table 4-4 operator operation operands result + addition integer or real integer or real - subtraction integer or real integer or real * multiplication integer or real integer or real / division integer or real real div truncated division integer integer mod modulo integer integer arithmetic operators, the mod operator, bit operators, table 4-5 bit operators operator operation operands result ~ bitwise not integer integer & bitwise and integer integer | bitwise or integer integer bitwise or (same as | ) integer integer, boolean operators, table 4-6 boolean operators operator operation operands result and conjunction boolean boolean and then similar to boolean and boolean boolean not negation boolean boolean or disjunction boolean boolean or else similar to boolean or boolean boolean, the and then operator.
Note - You cannot insert comments between the and and the then operators.
The or else Operator
Note - You cannot insert comments between the or and the else operators.
Set Operators
Table 4-7 set operators operator operation operands result + set union any set type same as operands - set difference any set type same as operands * set intersection any set type same as operands in member of a specified set 2nd arg:any set type 1st arg:base type of 2nd arg boolean, relational operators, table 4-8 relational operators operator operation operand results = equal any real, integer , boolean, char , record, array, set, or pointer type boolean <> not equal any real, integer, boolean, char, record, array, set, or pointer type boolean < less than any real, integer, boolean, char, string, or set type boolean <= less than or equal any real, integer, boolean, char, string, or set type boolean > greater than any real, integer, boolean, char, string, or set type boolean >= greater than or equal any real, integer, boolean, char, string, or set type boolean, relational operators on sets, the = and <> operators on records and arrays, string operators, precedence of operators, table 4-9 precedence of operators operators precedence ~, not , highest * , / , div , mod , and , & , . | , , + , - , or , . = , <> , < , <= , > , >= , in , . or else , and then lowest.
404 Not found
Code Translation Project
Don't lose in a world of programming languages
Pascal - operators
Arithmetic operators.
- Addition: + Addition is a matematical operation, it can be used for integers and real numbers. additio...
- Subtraction: - Subtraction is a matematical operation, it can be used for integers and real numbers. from...
- Multiplication: * Multiplication is a matematical operation, it can be used for integers and real numbers.mu...
- Division: / Division is a matematical operation, it can be used for integers and real numbers.
- Integer division: div Integer division is a mathematical operation that can be used only for whole numbers. the ...
- Modulo: mod Using the modulo operator we can calculate the remainder after integer division. by using ...
- Additive inverse: - Operator negative sign is used to change the sign of expressions. it works as a negation: ...
- Logical and: and Logical and works as follows: just in case it's true if both inputs are true in all other ...
- Logical or: or Logical OR works as follows: just in case it's the result false if both inputs are false, ...
- Logical negation: not The logical negation operator is used to determine the oposite value. in our case: if inpu...
- Bitwise left shift: shl Bit shift to the left as many time shifts the input number to the left as many as the valu...
- Bitwise right shift: shr Bit shift to the right as many time shifts the input number to the right as many as the va...
- Bitwise and: and Bitwise AND with another name bit clearing operation. it get the bit clear name after logi...
- Bitwise or: or Bitwise AND or with another name setting to 1. it get the setting to 1 name after logical ...
- Bitwise not: not Bitwise 1 complement, also known as bit negation or bit-denial operation. operates on the ...
- Bitwise xor: xor The main area of application of the bitwise exclusive OR is encryption, because it has suc...
- Less than: < Less than operator is a logical operator that is used to compare two numbers.
- Greater than: > Greater than operator is a logical operator that is used to compare two numbers.
- Less than or equal to: <= Less than or equal to operator is a logical operator that is used to compare two numbers.
- Greater than or equal to: >= Greater than or equal to operator is a logical operator that is used to compare two number...
- Equal to: = Equal to operator is a logical operator that is used to compare two numbers.
- Not equal to: <> Not equal to operator is a logical operator that is used to compare two numbers.
- Assignment: := The assignment operator is used to assign a value to a variable. it can be used for any da...
Examples - Pascal
Pascal Programming/Arrays
An array is a structure concept for custom data types. It groups elements of the same data type. You will use arrays a lot if you are dealing with lots of data of the same data type.
- 1.2 Declaration
- 2.1 Direct assignment
- 2.2 Reading and printing
- 2.3 Primitive comparison
- 4.1 Real literals
- 4.2 Limitations
- 4.3 Transfer functions
- 4.4 Printing real values
- 4.5 Comparisons
- 5.1 Flavors
- 5.2 Off limits divisor
- 6 Arrays as parameters
- 7.1 Special support
- 7.2 Introduction
- 7.3 Application
Lists [ edit | edit source ]
Notion [ edit | edit source ].
In general, an array is a limited and arranged aggregation of objects, all of which having the same data type called base type or component type . [1] An array has at least one discrete, bounded dimension, continuously enumerating all its objects. Each object can be uniquely identified by one or more scalar values, called indices , along those dimensions.
Declaration [ edit | edit source ]
In Pascal an array data type is declared using the reserved word array in combination with the auxiliary reserved word of , followed by the array’s base type:
Behind the word array follows a non-empty comma-separated list of dimensions surrounded by brackets. [fn 1] All array’s dimensions have to be ordinal data types, yet the base type type can be of any kind. If an array has just one dimension, like the one above, we may also call it a list .
Access [ edit | edit source ]
A variable of the data type integerList as declared above, holds five independent integer values. Accessing them follows a specific scheme:
This program will print 125 , since it is the value of powerN that has the index value 3 . Arrays are like a series of “buckets” each holding one of the base data type’s values. Every bucket can be identified by a value according to the dimension specifications. When referring to one of the buckets, we have to name the group, that is the variable name (in this case powerN ), and a proper index surrounded by brackets.
Character array [ edit | edit source ]
Lists of characters frequently have and had special support with respect to I/O and manipulation. This section is primarily about understanding, as in the next chapter we will get to know a more sophisticated data type called string .
Direct assignment [ edit | edit source ]
String literals can be assigned to array [ … ] of char variables using an assignment statement, thus instead of writing:
You can simply write:
Note, that you do not need to specify any index anymore. You are referring to the entire array variable on the LHS of the assignment symbol ( := ). This only works for overwriting the values of the whole array. There are extensions allowing you to overwrite merely parts of a char array, but more on that in the next chapter.
Most implementations of string literal to char array assignments will pad the given string literal with insignificant char values if it is shorter than the variable’s capacity. Padding a string means to fill the remaining positions with other characters in order meet a certain size. The GPC uses space characters ( ' ' ), whereas the FPC uses char values whose ord inal value is zero ( #0 ).
Reading and printing [ edit | edit source ]
Although not standardized, [fn 2] read / readLn and write / writeLn usually support writing to and reading from array [ … ] of char variables.
This works because text files, like the standard files input and output , are understood to be infinite sequences of char values. [fn 3] Since our array [ … ] of char is also, although finite sequence of char values, individual values can be copied pretty much directly to and from text files, not requiring any kind of conversion.
Primitive comparison [ edit | edit source ]
Unlike other arrays, array [ … ] of char variables can be compared using = and <> .
This kind of comparison only works as expected for identical data types. It is a primitive character-by-character comparison. If either array is longer or shorter, an = comparison will always fail, because not all characters can be compared to each other. Correspondingly, an <> comparison will always succeed for array [ … ] of char values that differ in length.
Note, the EP standard also defines the EQ and NE functions, beside many more. The difference to = and <> is that blank padding (i. e. #0 in FPC or ' ' in GPC) has no significance in EQ and NE . In consequence, that means using these functions you can compare strings and char arrays regardless of their respective maximum capacity and still get the naturally expected result. The = and <> comparisons on the other hand look at the memory’s internal representation.

Matrices [ edit | edit source ]
An array’s base type can be any data type, [fn 4] even another array. If we want to declare an “array of arrays” there is a short syntax for that:
This has already been described above . The last line is identical to:
It can be expanded to two separate declarations allowing us to “re-use” the “inner” array data type:
Note that in the latter case plot uses row as the base type which is an array by itself. Yet in the short notation we specify char as the base type, not a row but its base type.
When an array was declared to contain another array, there is a short notation for accessing individual array items, too:
This corresponds to the array’s declaration. It is vital to ensure the indices you are specifying are indeed valid. In the latter loop the if branch checks for that. Attempting to access non-existent array values, i. e. by supplying illegal indices, may crash the program, or worse remain undetected thus causing difficult to find mistakes.
Note, the “unusual” order of x and y has been chosen to facilitate drawing an upright graph:
That means, it is still possible to refer to entire “sub”-arrays as a whole. You are not forced to write all dimension an array value has, given it makes sense.
Array data types that have exactly two dimensions are also called matrices, singular matrix .
Real values [ edit | edit source ]
As introduced in one of the first chapters the data type real is part of the Pascal programming language. It is used to store integer values in combination with an optional fractional part.
Real literals [ edit | edit source ]
In order to distinguish integer literals from real literals, specifying real values in your source code (and also for read / readLn ) differs slightly. The following source code excerpt shows some real literals:
To summarize the rules:
- There is at least one Western-Arabic decimal digit before and one after the . (if there is any).
- The entire number and exponent are preceded by signs, yet a positive sign is optional.
As it has always been, all number values cannot contain spaces.
Limitations [ edit | edit source ]
epsReal is short for “epsilon real ”. The small Greek letter ε (epsilon) frequently denotes in mathematics an infinitely small (positive) value, yet not zero. According to the ISO standard 10206 (“Extended Pascal”), epsReal is the result of subtracting 1.0 from the smallest value that is greater than 1.0 . [3] No other value can be represented between this value and 1.0 , thus epsReal represents the highest precision available, but just at that point. [fn 6] Most implementations of the real data type will show a significantly varying degree of precision. Most notable, the precision of real data type implementations complying with the IEEE standard 754 format, decays toward the extremes, when approaching (and going beyond) - maxReal and maxReal . Therefore you usually use, if at all, a reasonable multiple of epsReal that fits the given situation.
Transfer functions [ edit | edit source ]
Pascal’s strong typing system prevents you from assigning real values to integer variables. The real value may contain a fractional part that an integer variable cannot store.
Pascal defines, as part of the language, two standard functions addressing this issue in a well-defined manner.
- The function trunc , short for “truncation”, simply discards any fractional part and returns, as an integer , the integer part. As a consequence this is effectively rounding a number toward zero . trunc ( - 1.999 ) will return the value - 1 .
- If this feels “unnatural”, the round function rounds a real number to its closest integer value. round ( x ) is (regarding the resulting value) equivalent to trunc ( x + 0.5 ) for non-negative values, and equivalent to trunc ( x - 0.5 ) for negative x values. [fn 7] In other words, this is what you were probably taught in grade school, or the first rounding method you learned in homeschooling. It is commonly referred to as “commercial rounding”.
Both functions will fail if there is no such integer value fulfilling the function’s respective definition.
There is no function if you want to (explicitly) “transfer” an integer value to a real value. Instead, one uses arithmetically neutral operations:
- integerValue * 1.0 (using multiplicative neutral element), or
- integerValue + 0.0 (using summing neutral element)
These expressions make use of the fact, as it was mentioned earlier as a passing remark in the chapter on expressions , that the expression’s overall data type will become real as soon as one real value is involved. [4]
Printing real values [ edit | edit source ]
By default write / writeLn print real values using “scientific notation”.
- sign, where a positive sign is replaced with a blank
- a positive number of post-decimal digits
- an E (uppercase or lowercase)
- the sign of the exponent, but this time a positive sign is always written and a zero exponent is preceded by a plus sign, too
- the exponent value, with a fixed minimum width (here 2) and leading zeros
While this style is very universal, it may also be unusual for some readers. Particularly the E notation is something now rather archaic, usually only seen on pocket calculators, i. e. devices lacking of enough display space.
Luckily, write / writeLn allow us to customize the displayed style.
The procedures write / writeLn accept for real type values (and only for real values) another colon-separated format specifier. This second number determines the (exact) number of post-decimal digits, the “fraction part”. Supplying two format specifiers also disables scientific notation. All real values are printed using regular positional notation. That may mean for “large” numbers such as 1e100 printing a one followed by a hundred zeros (just for the integer part).
In some regions and languages it is customary to use a , (comma) or other character instead of a dot as a radix mark. Pascal’s on-board write / writeLn procedures will, on the other hand, always print a dot, and for that matter – read / readLn will always accept dots as radix marks only. Nevertheless, all current Pascal programming suites, Delphi, FPC and GPC provide appropriate utilities to overcome this restriction. For further details we refer to their manuals. This issue should not keep us from continuing learning Pascal.
Comparisons [ edit | edit source ]
First of all, all (arithmetic) comparison operators do work with real values. The operators = and <> , though, are particularly tricky to handle.
In most applications you do not compare real values to each other when checking for equality or inequality. The problem is that numbers such as ⅓ cannot be stored exactly with a finite series of binary digits, only approximated, yet there is not one valid approximation for ⅓ but many legit ones. The = and <> operators, however, compare – so to speak — for specific bit patterns. This is usually not desired (for values that cannot be represented exactly). Instead, you want to ensure the values you are comparing are within a certain range, like:
Delphi and the FPC ’s standard RTS provide the function sameValue as part of the math unit . You do not want to program something other people already have programmed for you, i. e. use the resources.
Division [ edit | edit source ]
Now that we know the data type used for storing (a subset of) rational numbers, in Pascal known as real , we can perform and use the result of another arithmetic operation: The division.
Flavors [ edit | edit source ]
Pascal defines two different division operators:
- The div operator performs an integer division and discards, if applicable, any remainder. The expression’s resulting data type is always integer . The div operator only works if both operands are integer expressions.
- The, probably more familiar, operator / (a forward slash), divides the LHS number (the dividend) by the RHS number (the divisor), too, but a / -division always yields a real type value. [4] This is also the case if the fractional part is zero. A “remainder” does not exist for the / operation.
The div operation can be put in terms of / :
However, this is only a semantic equivalent, [fn 9] it is not how it is actually calculated. The reason is, the / operator would first convert both operands to real values and since, as explained above , not all integer values can necessarily be represented exactly as real values, this would produce results potentially suffering from rounding imprecisions. The div operator on the other hand is a pure integer operator and works with “integer precision”, that means no rounding is involved in actually calculating the div result.
Off limits divisor [ edit | edit source ]
Note, since there is no generally accepted definition for division by zero, a zero divisor is illegal and will result in your program to abort. If your divisor is not a constant and depends on run-time data (such as a variable read from user input), you should check that it is non-zero before doing a division:
Alternatively, you can declare data types excluding zero, so any assignment of a zero value will be detected:
Some Pascal dialects introduce the concept of “exceptions” that can be used to identify such problems. Exceptions may be mentioned again in the “Extensions” part of this Wikibook.
Arrays as parameters [ edit | edit source ]
Arrays can be copied with one simple assignment dataOut := dataIn ; . This requires, however, as it is customary with Pascal’s strong type safety, that both arrays are assignment-compatible: That means their base type and dimension specifications are the same. [fn 10]
Because calling a routine involves invisible assignments, writing general-purpose code dealing with lots of different situations would be virtually impossible if the entire program had to use one array type only. In order to mitigate this situation, conformant array type parameters allow writing routines accepting differing array dimension lengths . Array dimension data types still have to match .
Let’s look at an example program using this feature:
A conformant-array parameter looks pretty similar to a regular array variable declaration, but the dimensions are specified differently. Usually, when declaring new arrays you provide constant values as dimension limits. Since we do not want constants, though, we name placeholder identifiers for the actual dimension limits of any array printTableRows will receive. In this case they are named minimum and maximum , joined by .. inbetween indicating a range .
minimum and maximum become variables inside the definition of printTableRows , but you are not allowed to assign any values to them. [fn 11] You are not allowed to declare new identifiers bearing the same name as the array boundary variables.
In Pascal all constants implicitly have an unambiguously determinable data type. Since our array limit identifiers are in fact variables they require a data type. The : integer indicates both minimum and maximum have the data type integer .
Once we have declared and defined printTableRows we can use it with lots of differently sized arrays:
Delphi and the FPC (as of version 3.2.0 released in 2020) do not support conformant-array parameters, but the GPC does.
Logarithms [ edit | edit source ]
Special support [ edit | edit source ].
Prior the 21 st century logarithm tables and slide rules were heavily utilized tools in manual calculations, so much so it led to the inclusion of two basic functions in Pascal.
Both functions always return real values.
Introduction [ edit | edit source ]
Since the use of logarithms is not necessarily taught in all curricula, or you might just need a refresher, here is a short primer: The basic idea of logarithms is that all operations are lifted one level.
Once you are done, you have descend one level again to get the actual “real” result of the intended operation (on the underlying level). The reverse operation of ln is exp . [fn 12] To put this principle in Pascal terms:
Remember, x and y have to be positive in order to be valid parameters to ln .
Application [ edit | edit source ]
Taking the logarithm and then exponentiating values again are considered “slow” operations and introduce a certain overhead. In programming, overhead means taking steps that are not directly related to the actual underlying problem, but only facilitate solving it. In general, overhead is avoided, since (at first) it takes us even farther away from the solution.
As you can see, this goes to the detriment of precision. It is a compromise between fast operations, and “accurate enough” results.
Tasks [ edit | edit source ]
All tasks, including those in the following chapters, can be solved without conformant-array parameters. This takes account of the fact that not all major compilers support them. [fn 13] Nonetheless, using routines with conformant-array parameters are often the most elegant solution.
Calculating the maximum and minimum expected value now (as constants ) has the advantage that the compiler will emit a warning during compilation if any value exceeds maxInt .
The abs were inserted as a means of documentation: The program only works properly for non -negative values.
Using z as the table array’s base type (and not just integer ) has the advantage that if we accidentally implement the multiplication incorrectly , assigning out-of-range values will fail. For such a trivial task like this one it is of course irrelevant, but for more difficult situations deliberately constricting the allowed range can thwart programming mistakes. Do not worry if you just used a plain integer .
Note, the product variable has to be declared outside and before populateTable and printTable are defined. This way both routines refer to the same product variable. [fn 14]
It is also possible to reuse previously calculated values, make use of the fact that the table can be mirrored along the diagonal axis, or do other “optimization stunts”. The important thing for this task , though, is to correctly nest the for loops.
An advanced implementation would, of course, first determine the maximum expected length and store it as a variable, instead of using the hardcoded format specifier : 5 . This, though, is out of this task’s scope. [fn 15] It just should be mentioned hardcoded values like this one are considered bad style.
- The user will terminate his input with an empty line. Print this instruction beforehand.
- When done, print the message.
- When printing, a line may be at most 80 characters long (or whatever is reasonable for you). You are allowed to presume the user’s input lines are at most 80 characters long.
- Ensure you only wrap lines at space characters (unless there are no space characters in a line).
More tasks you can solve can be found on the following Wikibook pages:
- A-level Computing 2009/AQA/Problem Solving, Programming, Data Representation and Practical Exercise/Fundamentals of Programming/One-Dimensional Arrays
- A-level Computing 2009/AQA/Problem Solving, Programming, Data Representation and Practical Exercise/Fundamentals of Programming/Two-Dimensional Arrays
- ↑ a b Joslin, David A. (1989-06-01). "Extended Pascal – Numerical Features". Sigplan Notices . 24 (6): 77–80. doi : 10.1145/71052.71063 . The programmer can obtain some idea of the real range and precision from the (positive) implementation-defined constants MINREAL , MAXREAL and EPSREAL . Arithmetic in the ranges [ - maxreal ,- minreal ] , 0 , and [ minreal , maxreal ] "can be expected to work with reasonable approximations", whereas outside those ranges it cannot. As what constitutes a "reasonable approximation" is a matter of opinion, however, and is not defined in the standard, this statement may be less useful than it appears at first sight. The measure of precision is on somewhat firmer ground: EPSREAL is the commonly employed measure of (typically floating-point) accuracy, i.e the smallest value such that 1.0 + epsreal > 1.0 . {{ cite journal }} : line feed character in |quote= at position 259 ( help )
- ↑ Some (old) computers did not know the bracket characters. Seriously, that’s not a joke. Instead, a substitute bigram was used: var signCharacter : array ( . Boolean . ) of char ; , and signCharacter ( . true . ) := '-' ; . You may encounter this kind of notation in some (old) textbooks on Pascal. Anyway, using brackets is still the preferred method.
- ↑ Only I/O concerning a packed array [ 1 .. n ] of componentType , where n is greater than 1 and componentType is or is a subrange of char , is standardized. However, in this part of the book you are not introduced to the concept of packing , the packed keyword. Therefore, the shown behavior is non-standard.
- ↑ More precisely, text files are (possibly empty) sequences of lines , each line consisting of a (possibly empty) sequence of char values.
- ↑ Some compilers (such as the FPC ) allow zero-sized data types [not allowed in any ISO standard]. If that is the case, an array that has a zero-size base type will be rendered ineffective, virtually forfeiting all characteristics of arrays.
- ↑ Modern real arithmetic processors can indicate a precision loss , i. e. when the result of an arithmetic operation had to be “approximated”. However, there is no standardized way to access this kind of information from your Pascal source code, and usually this kind of signaling is also not favorable, since the tiniest precision loss will set off the alarm, thus slowing down your program. Instead, if it matters, one uses software that allows arbitrary precision arithmetics, like for example the GNU Multiple Precision Arithmetic Library .
- ↑ This number is not completely arbitrary. The most prevalent real number implementation IEEE 754 uses a “hidden bit”, making the value 1.0 special.
- ↑ Not all compilers comply with this definition of the standard. The FPC ’s standard round implementation will round in the case of equidistance toward even numbers. Knowing this is relevant for statistical applications. The GPC uses for its round implementation functionality provided by the hardware. As such, the implementation is hardware-dependent, on its specific configuration, and may deviate from the ISO 7185 standard definition.
- ↑ Given the most prevalent implementations Two’s complement for integer values and IEEE 754 for real values, you have to consider the fact that (virtually) all bits in an integer contribute to its (mathematical) value, whereas a real number stores values for the expression mantissa * base pow exponent . In very simple terms, the mantissa stores the integer part of a value, but the problem is that it occupies fewer bits than an integer would use, thus there is (for values that require more bits) a loss in information (i. e. a loss in precision).
- ↑ The exact technical definition reads like: The value of dividend div divisor shall be such that abs ( dividend ) - abs ( divisor ) < abs (( dividend div divisor ) * divisor ) <= abs ( dividend ) where the value shall be zero if abs ( dividend ) < abs ( divisor ) ; otherwise, […]
- ↑ Furthermore, both arrays have to be either packed or “unpacked”.
- ↑ The EP standard calls this characteristic protected .
- ↑ The ISO standard 7185 (“Standard Pascal”) calls this, lack of conformant-array parameters, “Level 0 compliance”. “Level 1 compliance” includes support for conformant array parameters. Of the compilers presented in Getting started only the GPC is a “Level 1”-compliant compiler.
- ↑ This style of programming is slightly disfavored, keyword “global variables”, but as for now we do not know appropriate syntax ( var parameters ) to not do that.
- ↑ In EP there also exists a real power operator ** . The difference is similar to that of the division operators : pow only accepts integer values as operands and yields an integer value, whereas ** always yields a real value. Your choice for either of which, again, should be based on the required degree of precision.
- Book:Pascal Programming
- CS1 errors: invisible characters
Navigation menu
Once you have declared a variable, you can store values in it. This is called assignment .
To assign a value to a variable, follow this syntax:
Note that unlike other languages, whose assignment operator is just an equals sign, Pascal uses a colon followed by an equals sign, similarly to how it's done in most computer algebra systems.
The expression can either be a single value:
or it can be an arithmetic sequence:
The arithmetic operators in Pascal are:
div and mod only work on integers. / works on both reals and integers but will always yield a real answer. The other operations work on both reals and integers. When mixing integers and reals, the result will always be a real since data loss would result otherwise. This is why Pascal uses two different operations for division and integer division. 7 / 2 = 3.5 (real), but 7 div 2 = 3 (and 7 mod 2 = 1 since that's the remainder).
When the following block of statements executes,
some_real will have a value of 375.0.
Changing one data type to another is referred to as typecasting . Modern Pascal compilers support explicit typecasting in the manner of C, with a slightly different syntax. However, typecasting is usually used in low-level situations and in connection with object-oriented programming, and a beginning programming student will not need to use it. Here is information on typecasting from the GNU Pascal manual .
In Pascal, the minus sign can be used to make a value negative. The plus sign can also be used to make a value positive, but is typically left out since values default to positive.
Do not attempt to use two operators side by side, like in:
This may make perfect sense to you, since you're trying to multiply by negative-2. However, Pascal will be confused — it won't know whether to multiply or subtract. You can avoid this by using parentheses to clarify:
The computer follows an order of operations similar to the one that you follow when you do arithmetic. Multiplication and division ( * / div mod ) come before addition and subtraction ( + - ), and parentheses always take precedence. So, for example, the value of: 3.5*(2+3) will be 17.5 .
Pascal cannot perform standard arithmetic operations on Booleans. There is a special set of Boolean operations. Also, you should not perform arithmetic operations on characters.
│ Deutsch (de) │ English (en) │ suomi (fi) │ An operator is a special kind of function . It can be invoked by placing keywords adjacent to suitable operands.
The word operator colloquially refers to the symbol or keyword identifying the function that implements the actual operation. operator is also a reserved word that appears in the course of operator overloading .
- 2.1 evaluation order
Most operators are binary, that means require two operands. Binary operator symbols appear between two operands, like this:
The plus character identifies the addition operation (Standard Pascal). x and 5 are its operands. This style is called infix notation.
Unary operators – those which require only one operand – appear in front of their operands (prefix notation), with the exception of ^ , the de-referencing operator, which appears after its operand (postfix notation). Example:
Here, the minus character identifies the sign inversion operation (Standard Pascal). Blanks between operator and operand do not harm, confer the following section regarding precedence . However, it is common practice to place unary operator symbols back to back with their operands.
Operator symbols always appear explicitly, except the implicit typecast and enumerator operator. Unlike in mathematics, no invisible “times” is assumed between two identifiers.
Implicit typecasts occur, where a value has to be stored in a memory location. Note, that calling a routine triggers implicit typecasts, too. In order to pass the parameters to the routine, they are stored somewhere. Implicit typecasts are not limited to run-of-the-mill assignment statements.
operator precedence
Operands and operators are the building blocks of expressions . The use of operators is a powerful notational tool, since LOCs do not get cluttered by function calls consisting of function identifiers and parentheses, but instead the operands and (ideally) a short symbol achieve the same. Instead of, for example, sum ( x , - 8 ) the expression x - 8 evaluates to the same value, while writing five less characters.
In order to increase conciseness subexpressions of expressions propagate their intermediate result in a predefined hierarchy. This hierarchy can be superseded by placing parentheses ( ) around subexpressions that shall be treated as one expression, so the regular precedence rules apply in the enclosed part remaining uninfluenced from the expression’s rest. Operators that have have higher precedence, bind to operands stronger.
† ) This symbol does not refer to an actual operator, but is (imprecisely) called as one.
Inc and dec are pseudo operators: They may be redefined via the operator overloading mechanism, but they can not appear in expressions, like any function could. Therefore their precedence is moot.
Include and exclude are also not operators, but shorthand for common LOCs.
@ and ^ albeit being called operators, are not operators, but rather instruct the compiler to interpret an identifier differently than usual. This is not done via any function, but compiler intrinsics.

evaluation order
No assumptions shall be made, and there is no guarantee, that expressions are evaluated from left to right (or the reverse direction). The FPC for instance, evaluates more “complex” subexpressions first before moving on to “trivial” parts (ratio: avoiding register spilling).
If a subexpression has to be evaluated first, e.g. a function triggering some side-effects, the expression has to be split up into two separate statements. For example:
The compiler may evaluate foo () or bar () first. If bar () ought to be evaluated first at all events, the statement has to be split into two separate ones:
- “Operators” in the FreePascal Reference Guide
- Tutorial: assignment and operations
- management operators – a collection of specially supported routines
- Reserved words
Navigation menu
Page actions.
- View source
- In other languages
Personal tools
- Create account
- Documentation
- Recent changes
- Random page
- What links here
- Related changes
- Special pages
- Printable version
- Permanent link
- Page information
- This page was last edited on 6 August 2022, at 16:37.
- Content is available under unless otherwise noted.
- Privacy policy
- About Lazarus wiki
- Disclaimers
[ next ] [ tail ] [ up ]
1.1 Symbols
[ next ] [ front ] [ up ]

IMAGES
VIDEO
COMMENTS
1E - Assignment and Operations (author: Tao Yue, state: unchanged) Once you have declared a variable, you can store values in it. This is called assignment. To assign a value to a variable, follow this syntax: variable_name := expression; Note that unlike other languages, whose assignment operator is just an equals sign, Pascal uses a colon ...
15.3 Assignment operators. The assignment operator defines the action of a assignment of one type of variable to another. The result type must match the type of the variable at the left of the assignment statement, the single parameter to the assignment operator must have the same type as the expression at the right of the assignment operator.
In some languages, the symbol used is regarded as an operator (meaning that the assignment statement as a whole returns a value). Other languages define assignment as a statement (meaning that it cannot be used in an expression). ... In ALGOL and Pascal, the assignment operator is a colon and an equals sign (":=") while the equality operator is ...
An operator is a symbol that tells the compiler to perform specific mathematical or logical manipulations. Pascal allows the following types of operators −. Arithmetic operators. Relational operators. Boolean operators. Bit operators. Set operators. String operators. Let us discuss the arithmetic, relational, Boolean and bit operators one by one.
Use the relational operators to compare sets of identical types. The result is a boolean ( true or false) value. The Pascal program, sets.p, which applies the < and > operators to two sets of colors. The < operator tests if a set is a subset of another set. The > operator tests if a set is a proper subset of another set.
Assignments give a value to a variable, replacing any previous value the variable might have had: In addition to the standard Pascal assignment operator ( := ), which simply replaces the value of the variable with the value resulting from the expression on the right of the := operator, Free Pascal supports some C-style constructions.
types. It does not, however, give a detailed explanation of the Pascal language: it is not a tuto-rial. The aim is to list which Pascal constructs are supported, and to show where the Free Pascal implementation differs from the Turbo Pascal or Delphi implementations.---˙---˙---˙---˙---˙
Chapter 12 Expressions. Expressions occur in assignment statements or in tests. Expressions are built of two components: operators and their operands. Most operators are binary, i. e. require two operands. Binary operators occur always between the operands (as in X/Y ). Few operators are unary, i. e. require only one operand.
1E - Assignment press Operation. Once you have declared a variable, them can store values in it. This is called assignment. To assign a value into a variable, keep this syntax: variable_name:= expression; Note that unlike other languages, who assignment operator can simply an equals sign, Pascal uses a colon followed by an equals sign.
Free Pascal operators Address operator. The address operator @ returns the start address of a variable, a procedure or a function. If the compiler switch is {$ T-}, the return value is an untyped pointer. If the compiler switch is {$ T +}, the return value is a typed pointer. The default setting for the compiler is {$ T-}. Assignment operator
The semantics of this line of code varies among every programming language. For instance, in Fortran and in Basic this line means "Compare the values m and x, and if they are equal to each other n becomes true, false otherwise." In contrast to that the C programming language will assign m the value of x, and subsequently assign the n the value of m, so n and m both have the value x.
Pascal - Assignment: :=. The assignment operator is used to assign a value to a variable. it can be used for any data type with the only condition that the first parameter (left side) must be a value receiver, for example a variable. depending on the programming language can be different terms for the assignment of values to the variables, for ...
$\begingroup$ Actually, = and := for assignment both date back to the dawn of high-level languages: FORTRAN used = in 1957, and ALGOL 58 used :=.Today the main distinction is between C-like syntax (with = and ==) and Pascal-like syntax (with := and =); C and Pascal were both initially developed in the early 1970s.It doesn't seem really meaningful do call that a distinction between "early" and ...
Using these symbols yield Boolean expressions. The value of the expression will be either true or false depending on the operator's definition.. All those relational operators require operands on both sides to be of the same data type. Although we can say '$' = 1337 is wrong, that means it should evaluate to the value false, it is nevertheless illegal, because '$' is a char ‑expression and ...
The two languages use different operators for assignment. Pascal, like ALGOL, uses the mathematical equality operator = for the equality test and the symbol := for assignment, whereas C, like B, uses the mathematical equality operator for assignment. In C (and B) the == symbol of FORTRAN was chosen for the equality test.
Pascal. Operators Pascal - operators Operators perform an operation between the operands, this operation can be mathematical, logical, or bitwise. Arithmetic operators. ... = The assignment operator is used to assign a value to a variable. it can be used for any da... Examples - Pascal. Pascal Other pieces of example codes: if y < 5 then y:= y ...
Note, that you do not need to specify any index anymore. You are referring to the entire array variable on the LHS of the assignment symbol (:=).This only works for overwriting the values of the whole array. There are extensions allowing you to overwrite merely parts of a char array, but more on that in the next chapter.. Most implementations of string literal to char array assignments will ...
No difference. Problem with = is that it confuses many people as it is more commonly used by scientists as the equals to operator.:= was used by Pascal programming language to express the assignment, and makes the difference with = to test equality. Algorithmic pseudo-language frequently use <-for assignment, suggesting that the value of the right part is pushed onto the variable on the left.
This is why Pascal uses two different operations for division and integer division. 7 / 2 = 3.5 (real), but 7 div 2 = 3 (and 7 mod 2 = 1 since that's the remainder). Each variable can only be assigned a value that is of the same data type. Thus, you cannot assign a real value to an integer variable. However, certain data types will convert to a ...
5) The line Sum := 25 + 33 + 75; is an assignment statement. The symbol := means "is assigned the value". Note the colon : must be used. The statement assigns what ever is on the right hand side of the := to the variable name on the left hand side. PASCAL arithmetic operators are + - * / operands may be integer or real result is real
Operator. An operator is a special kind of function . It can be invoked by placing keywords adjacent to suitable operands. The word operator colloquially refers to the symbol or keyword identifying the function that implements the actual operation. operator is also a reserved word that appears in the course of operator overloading .
When used in a range specifier, the character pair (. is equivalent to the left square bracket [.Likewise, the character pair .) is equivalent to the right square bracket ].When used for comment delimiters, the character pair (* is equivalent to the left brace { and the character pair *) is equivalent to the right brace }.These character pairs retain their normal meaning in string expressions.
The symbol goes much further back, to APL and the Pascal family of languages. It's meant to resemble APL's left-pointing arrow, which is of course not part of ASCII. That's why people associate it with "imperative assignment" in the discussion. - alexis. Apr 18, 2013 at 10:17