Home » PHP Tutorial » PHP Array Destructuring

PHP Array Destructuring
Summary : in this tutorial, you’ll learn how to use PHP array destructuring to assign elements of an array to multiple variables.
Introduction to the PHP array destructuring
Suppose that you have an array returned by a function parse_url() :
To assign the elements of the array to multiple variables, you can use the list() construct like this:
PHP 7.1 introduced a new way of unpacking the array’s elements to variables. It’s called array destructuring:
Like the list() , the array destructuring works with both indexed and associative arrays . For example:
Skipping elements
The array destructuring syntax also allows you to skip any elements. For example, the following skips the second element of the array:
PHP array destructuring examples
Let’s take some examples of using the PHP array destructuring syntax.
1) Swaping variables
To swap the values of two variables, you often use a temporary variable like this:
Now, you can use the array destructuring syntax to make the code shorter:
2) Parsing an array returned from a function
Many functions in PHP return an array. The array destructuring allows you to parse an array returned from a function.
For example:
- Use the PHP array destructuring to unpack elements of an array to variables.
Array destructuring in PHP
In my day to day job I write in a number of programming languages. The majority of my time is spent writing PHP but I very much enjoy writing other languages, such as Go and Javascript, too!
One of the things I like the most about JavaScript, and PHP also to some extent, is how flexible and expressive they CAN be (but not always are). I also believe that JavaScript, PHP, and Python have a number of features that make them a good fit for serving the forefront of the web. Most of those features have to do with loose and dynamic typing. Personally I like how freely you can transform data without worrying too much about the structure.
The most versatile type of PHP, to me, is the array . The array can be used to act like many different classic data types. You can use them as a List , a Set (although that requires some specific handling), a HashMap , just to name a few.
PHP 7.1 array super-powers!
In PHP 7.1 the array type has become even more powerful! An RFC was accepted (and implemented) to provide square bracket syntax for array destructuring assignment .
This change to the language made it possible to extract values from array more easily. For example, consider the following options array:
If we'd want to assign the options to variables we could use something like this:
This is not so bad, but using the features of this new RFC we could have a slightly more expressive way to define this. You might know this kind of expression if you've used ES6's form of this.
In this example the enabled and compression key are extracted into variables in a language contract that can be viewed as the inverse of the initial options assignment. In this example the variable names are implicit. In the full form the example is a little more verbose.
The expansion also allows for renamed and can be mixed with the shorter version:
Since version 7.1 we can use these same type of syntax in PHP too!
In this example the value of the enabled key is assigned to the $enabled variable and the same goes for the compression key.
List assignments
Apart from associative array destructuring, list destructuring is also possible. This type of destructuring is particularly useful for when you're using arrays as you would a Tuple . A tuple is a finite ordered list that holds a fixed number of items.
It also works when you're using variables in the source array, so you can effectively swap variables using this syntax:
Nested destructuring
In many PHP application the application is responsible for retrieving a large set of nested data. In this case the nested form of array destructuring can come in handy.
This nested form can also be combined with the list destructuring, so you can go as wild as you feel like it.
Or if you really want to loose your mind, consider this example:
I hope you have fun exploring the new possibilities using these techniques!
Subscribe for updates
Get the latest posts delivered right to your inbox.
Continue reading

Array destructuring in PHP
# list or [].
In PHP, list or [] is a so called "language construct", just like array() . This language construct is used to "pull" variables out of an array. In other words: it will "destructure" the array into separate variables.
Note that the word is "destructure", not "destruction" — that's something different 😉
Here's what that looks like:
Whether you prefer list or its shorthand [] is up to you. People might argue that [] is ambiguous with the shorthand array syntax, and therefor prefer list . I'll be using the shorthand version in code samples though.
So what more can list do?
# Skip elements
Say you only need the third element of an array, the first two can be skipped by simply not providing a variable.
Also note that list will always start at index 0. Take for example the following array:
The first variable pulled out with list would be null , because there's no element with index 0 . This might seem like a shortcoming, but luckily there are more possibilities.
# Non-numerical keys
PHP 7.1 allows list to be used with arrays that have non-numerical keys. This opens a world of possibilities.
As you can see, you can change the order however you want, and also skip elements entirely.
# In practice
One of the uses of list are functions like parse_url and pathinfo . Because these functions return an array with named parameters, we can use list to pull out the information we'd like:
As you can see, the variables don't need the same name as the key. Also note that destructuring an array with an unknown key will trigger a notice:
In this case, $query would be null .
One last detail: trailing commas are allowed with named destructs, just like you're used to with arrays.
You can also use the list construct in loops:
This could be useful when parsing for example a JSON or CSV file. Be careful though that undefined keys will still trigger a notice.
In summary, there are some pretty good cases in which list can be of help!
- Language Reference
An array in PHP is actually an ordered map. A map is a type that associates values to keys . This type is optimized for several different uses; it can be treated as an array, list (vector), hash table (an implementation of a map), dictionary, collection, stack, queue, and probably more. As array values can be other array s, trees and multidimensional array s are also possible.
Explanation of those data structures is beyond the scope of this manual, but at least one example is provided for each of them. For more information, look towards the considerable literature that exists about this broad topic.
Specifying with array()
An array can be created using the array() language construct. It takes any number of comma-separated key => value pairs as arguments.
The comma after the last array element is optional and can be omitted. This is usually done for single-line arrays, i.e. array(1, 2) is preferred over array(1, 2, ) . For multi-line arrays on the other hand the trailing comma is commonly used, as it allows easier addition of new elements at the end.
Note : A short array syntax exists which replaces array() with [] .
Example #1 A simple array
The key can either be an int or a string . The value can be of any type.
- String s containing valid decimal int s, unless the number is preceded by a + sign, will be cast to the int type. E.g. the key "8" will actually be stored under 8 . On the other hand "08" will not be cast, as it isn't a valid decimal integer.
- Float s are also cast to int s, which means that the fractional part will be truncated. E.g. the key 8.7 will actually be stored under 8 .
- Bool s are cast to int s, too, i.e. the key true will actually be stored under 1 and the key false under 0 .
- Null will be cast to the empty string, i.e. the key null will actually be stored under "" .
- Array s and object s can not be used as keys. Doing so will result in a warning: Illegal offset type .
If multiple elements in the array declaration use the same key, only the last one will be used as all others are overwritten.
Example #2 Type Casting and Overwriting example
The above example will output:
As all the keys in the above example are cast to 1 , the value will be overwritten on every new element and the last assigned value "d" is the only one left over.
PHP arrays can contain int and string keys at the same time as PHP does not distinguish between indexed and associative arrays.
Example #3 Mixed int and string keys
The key is optional. If it is not specified, PHP will use the increment of the largest previously used int key.
Example #4 Indexed arrays without key
It is possible to specify the key only for some elements and leave it out for others:
Example #5 Keys not on all elements
As you can see the last value "d" was assigned the key 7 . This is because the largest integer key before that was 6 .
Example #6 Complex Type Casting and Overwriting example
This example includes all variations of type casting of keys and overwriting of elements.
Accessing array elements with square bracket syntax
Array elements can be accessed using the array[key] syntax.
Example #7 Accessing array elements
Note : Prior to PHP 8.0.0, square brackets and curly braces could be used interchangeably for accessing array elements (e.g. $array[42] and $array{42} would both do the same thing in the example above). The curly brace syntax was deprecated as of PHP 7.4.0 and no longer supported as of PHP 8.0.0.
Example #8 Array dereferencing
Note : Attempting to access an array key which has not been defined is the same as accessing any other undefined variable: an E_WARNING -level error message ( E_NOTICE -level prior to PHP 8.0.0) will be issued, and the result will be null .
Note : Array dereferencing a scalar value which is not a string yields null . Prior to PHP 7.4.0, that did not issue an error message. As of PHP 7.4.0, this issues E_NOTICE ; as of PHP 8.0.0, this issues E_WARNING .
Creating/modifying with square bracket syntax
An existing array can be modified by explicitly setting values in it.
This is done by assigning values to the array , specifying the key in brackets. The key can also be omitted, resulting in an empty pair of brackets ( [] ).
If $arr doesn't exist yet or is set to null or false , it will be created, so this is also an alternative way to create an array . This practice is however discouraged because if $arr already contains some value (e.g. string from request variable) then this value will stay in the place and [] may actually stand for string access operator . It is always better to initialize a variable by a direct assignment.
Note : As of PHP 7.1.0, applying the empty index operator on a string throws a fatal error. Formerly, the string was silently converted to an array.
Note : As of PHP 8.1.0, creating a new array from false value is deprecated. Creating a new array from null and undefined values is still allowed.
To change a certain value, assign a new value to that element using its key. To remove a key/value pair, call the unset() function on it.
Note : As mentioned above, if no key is specified, the maximum of the existing int indices is taken, and the new key will be that maximum value plus 1 (but at least 0). If no int indices exist yet, the key will be 0 (zero). Note that the maximum integer key used for this need not currently exist in the array . It need only have existed in the array at some time since the last time the array was re-indexed. The following example illustrates: <?php // Create a simple array. $array = array( 1 , 2 , 3 , 4 , 5 ); print_r ( $array ); // Now delete every item, but leave the array itself intact: foreach ( $array as $i => $value ) { unset( $array [ $i ]); } print_r ( $array ); // Append an item (note that the new key is 5, instead of 0). $array [] = 6 ; print_r ( $array ); // Re-index: $array = array_values ( $array ); $array [] = 7 ; print_r ( $array ); ?> The above example will output: Array ( [0] => 1 [1] => 2 [2] => 3 [3] => 4 [4] => 5 ) Array ( ) Array ( [5] => 6 ) Array ( [0] => 6 [1] => 7 )
Array destructuring
Arrays can be destructured using the [] (as of PHP 7.1.0) or list() language constructs. These constructs can be used to destructure an array into distinct variables.
Array destructuring can be used in foreach to destructure a multi-dimensional array while iterating over it.
Array elements will be ignored if the variable is not provided. Array destructuring always starts at index 0 .
As of PHP 7.1.0, associative arrays can be destructured too. This also allows for easier selection of the right element in numerically indexed arrays as the index can be explicitly specified.
Array destructuring can be used for easy swapping of two variables.
Note : The spread operator ( ... ) is not supported in assignments.
Useful functions
There are quite a few useful functions for working with arrays. See the array functions section.
Note : The unset() function allows removing keys from an array . Be aware that the array will not be reindexed. If a true "remove and shift" behavior is desired, the array can be reindexed using the array_values() function. <?php $a = array( 1 => 'one' , 2 => 'two' , 3 => 'three' ); unset( $a [ 2 ]); /* will produce an array that would have been defined as $a = array(1 => 'one', 3 => 'three'); and NOT $a = array(1 => 'one', 2 =>'three'); */ $b = array_values ( $a ); // Now $b is array(0 => 'one', 1 =>'three') ?>
The foreach control structure exists specifically for array s. It provides an easy way to traverse an array .
Array do's and don'ts
Why is $foo[bar] wrong.
Always use quotes around a string literal array index. For example, $foo['bar'] is correct, while $foo[bar] is not. But why? It is common to encounter this kind of syntax in old scripts:
This is wrong, but it works. The reason is that this code has an undefined constant ( bar ) rather than a string ( 'bar' - notice the quotes). It works because PHP automatically converts a bare string (an unquoted string which does not correspond to any known symbol) into a string which contains the bare string . For instance, if there is no defined constant named bar , then PHP will substitute in the string 'bar' and use that.
The fallback to treat an undefined constant as bare string issues an error of level E_NOTICE . This has been deprecated as of PHP 7.2.0, and issues an error of level E_WARNING . As of PHP 8.0.0, it has been removed and throws an Error exception.
Note : This does not mean to always quote the key. Do not quote keys which are constants or variables , as this will prevent PHP from interpreting them. <?php error_reporting ( E_ALL ); ini_set ( 'display_errors' , true ); ini_set ( 'html_errors' , false ); // Simple array: $array = array( 1 , 2 ); $count = count ( $array ); for ( $i = 0 ; $i < $count ; $i ++) { echo "\nChecking $i : \n" ; echo "Bad: " . $array [ '$i' ] . "\n" ; echo "Good: " . $array [ $i ] . "\n" ; echo "Bad: { $array [ '$i' ]} \n" ; echo "Good: { $array [ $i ]} \n" ; } ?> The above example will output: Checking 0: Notice: Undefined index: $i in /path/to/script.html on line 9 Bad: Good: 1 Notice: Undefined index: $i in /path/to/script.html on line 11 Bad: Good: 1 Checking 1: Notice: Undefined index: $i in /path/to/script.html on line 9 Bad: Good: 2 Notice: Undefined index: $i in /path/to/script.html on line 11 Bad: Good: 2
More examples to demonstrate this behaviour:
When error_reporting is set to show E_NOTICE level errors (by setting it to E_ALL , for example), such uses will become immediately visible. By default, error_reporting is set not to show notices.
As stated in the syntax section, what's inside the square brackets (' [ ' and ' ] ') must be an expression. This means that code like this works:
This is an example of using a function return value as the array index. PHP also knows about constants:
Note that E_ERROR is also a valid identifier, just like bar in the first example. But the last example is in fact the same as writing:
because E_ERROR equals 1 , etc.
So why is it bad then?
At some point in the future, the PHP team might want to add another constant or keyword, or a constant in other code may interfere. For example, it is already wrong to use the words empty and default this way, since they are reserved keywords .
Note : To reiterate, inside a double-quoted string , it's valid to not surround array indexes with quotes so "$foo[bar]" is valid. See the above examples for details on why as well as the section on variable parsing in strings .
Converting to array
For any of the types int , float , string , bool and resource , converting a value to an array results in an array with a single element with index zero and the value of the scalar which was converted. In other words, (array)$scalarValue is exactly the same as array($scalarValue) .
If an object is converted to an array , the result is an array whose elements are the object 's properties. The keys are the member variable names, with a few notable exceptions: integer properties are unaccessible; private variables have the class name prepended to the variable name; protected variables have a '*' prepended to the variable name. These prepended values have NUL bytes on either side. Uninitialized typed properties are silently discarded.
These NUL can result in some unexpected behaviour:
The above will appear to have two keys named 'AA', although one of them is actually named '\0A\0A'.
Converting null to an array results in an empty array .
It is possible to compare arrays with the array_diff() function and with array operators .
Array unpacking
An array prefixed by ... will be expanded in place during array definition. Only arrays and objects which implement Traversable can be expanded. Array unpacking with ... is available as of PHP 7.4.0.
Example #9 Simple array unpacking
Example #10 Array unpacking with duplicate key
Note : Keys that are neither integers nor strings throw a TypeError . Such keys can only be generated by a Traversable object.
Note : Prior to PHP 8.1, unpacking an array which has a string key is not supported: <?php $arr1 = [ 1 , 2 , 3 ]; $arr2 = [ 'a' => 4 ]; $arr3 = [... $arr1 , ... $arr2 ]; // Fatal error: Uncaught Error: Cannot unpack array with string keys in example.php:5 $arr4 = [ 1 , 2 , 3 ]; $arr5 = [ 4 , 5 ]; $arr6 = [... $arr4 , ... $arr5 ]; // works. [1, 2, 3, 4, 5] ?>
The array type in PHP is very versatile. Here are some examples:
Example #11 Using array()
Example #12 Collection
Changing the values of the array directly is possible by passing them by reference.
Example #13 Changing element in the loop
This example creates a one-based array.
Example #14 One-based index
Example #15 Filling an array
Array s are ordered. The order can be changed using various sorting functions. See the array functions section for more information. The count() function can be used to count the number of items in an array .
Example #16 Sorting an array
Because the value of an array can be anything, it can also be another array . This enables the creation of recursive and multi-dimensional array s.
Example #17 Recursive and multi-dimensional arrays
Array assignment always involves value copying. Use the reference operator to copy an array by reference.
Improve This Page
User contributed notes 5 notes.


DEV Community

Posted on Aug 8, 2020
Array Destructuring In PHP
Prerequisite :.
To understand this article, you should have a basic understanding of what arrays are and how they work in PHP. If you don't, it's okay, watch the video, I think you would understand, better.
What exactly is array destructuring?
Array destructuring is simply a way to extract array elements into separate variables.
Let's quickly look at some examples to better understand what this is, shall we?
Assuming we have this array of brands.
$brands = [ 'Apple', 'Dell', 'Lenovo' ];
Let's pretend that we have received this from a server or something. We might not necessarily want to loop through everything to extract their values. In that case, we might do the following.
Consider this code below:
Now, this is a valid PHP code of course, and there's nothing wrong with it, but wouldn't it be nice if we could trim the code a little? Hell yes, we definitely can!
There are two ways to destructure Arrays in PHP.
- Using the list() construct
- Using the [] shorthand/symmetric syntax
Destructuring Indexed/numerical Arrays
Using the list() construct.
Consider the code below:
Skipping an element(s)?
The example above is not very practical because we have an indexed array. So, imagine if we have up to ten, twenty, fifty, or a hundred elements. We are better off with loops and some conditional statements within the loop. The example is merely to illustrate how this works with indexed arrays.
Using The Shorthand Syntax
Now that we have seen how to destructure an indexed array using the list() construct, let's also see how to do the same with the shorthand syntax (square brackets[]).
Consider the following code:
Destructuring Associative Arrays
Here we have an array of a person with two elements.
Before PHP 7.1.0, the list() construct only worked on indexed arrays. To know the version of PHP installed on your server, run the code below: echo PHP_VERSION . PHP_EOL; The PHP_VERSION constant gives you the version number, PHP_EOL is only ending the line for us.
When working with associative arrays, we don't have to worry about putting the variables in order of the elements. All we need to do is specify the key of the array element we want.
Destructuring Nested Arrays
Consider the following example:
Destructuring In Loops
Destructuring in practice.
Thanks for reading, I hope it was helpful. 🙂
Top comments (0)
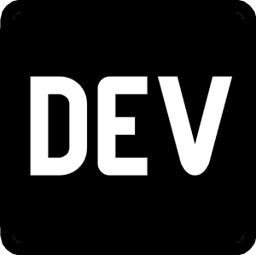
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse

Backup and Restore MongoDB in a Docker Environment
Denis AKPAGNONITE - Jul 21
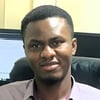
✨ Simple trick for printing a div
Joshua Amaju - Aug 1

Me and my new work 👩💻
Nadia Barra - Aug 4

Integrating C/C++ Libraries to .NET Applications with P/Invoke
Tural Suleymani - Aug 1
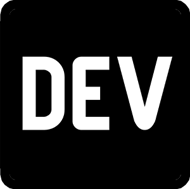
We're a place where coders share, stay up-to-date and grow their careers.
Destructuring Arrays
In PHP, array destructuring, also known as array unpacking, is a feature that allows you to assign elements of an array or properties of an object to separate variables in a single statement. It's a handy way to extract multiple values from an array or object at once.
The problem
Consider the following array:
Traditionally, if you wanted to assign each of these values to a separate variable, you would have to do it individually like this:
Destructuring the array
But with array destructuring, you can do this in one line:
After this line, $name would contain 'Alice' , $age would contain 30 , and $profession would contain 'Engineer' . You can then use these variables in your code as you see fit.
We can use the list keyword to instruct PHP to extract the values from the $data variable.
The variables on the left-hand side of the assignment must match the number of elements in the array. If there are fewer variables than array elements, the remaining elements will not be assigned.
An alternative syntax to the list keyword is to wrap the variables with the [] characters. It's shorter than using list .
However, list() is less flexible because it only works with numerically indexed arrays and doesn't support skipping values or nested destructuring.
Associative arrays
Array destructuring works with associative arrays, too. The keys in the array do not need to be in order.
Skipping values
You can also skip values in the array that you're not interested in by leaving empty spaces.
Key takeaways
- Array destructuring in PHP is a way to assign values from an array to variables in a single statement.
- The number of variables and array elements should match. If not, the remaining array elements are ignored, or extra variables get a NULL value.
- By leaving empty spaces, you can skip over values in the array you're not interested in.
- list was used for a similar purpose prior to PHP 7.1, but it has limitations compared to modern array destructuring.
- Despite making the code more concise, improper use of array destructuring can lead to code that is harder to read and understand. Balance is key.
Please read this before commenting
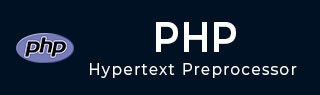
- PHP Tutorial
- PHP - Introduction
- PHP - Installation
- PHP - History
- PHP - Features
- PHP - Syntax
- PHP - Hello World
- PHP - Comments
- PHP - Variables
- PHP - Echo/Print
- PHP - var_dump
- PHP - $ and $$ Variables
- PHP - Constants
- PHP - Magic Constants
- PHP - Data Types
- PHP - Type Casting
- PHP - Type Juggling
- PHP - Strings
- PHP - Boolean
- PHP - Integers
- PHP - Files & I/O
- PHP - Maths Functions
- PHP - Heredoc & Nowdoc
- PHP - Compound Types
- PHP - File Include
- PHP - Date & Time
- PHP - Scalar Type Declarations
- PHP - Return Type Declarations
- PHP Operators
- PHP - Operators
- PHP - Arithmatic Operators
- PHP - Comparison Operators
- PHP - Logical Operators
- PHP - Assignment Operators
- PHP - String Operators
- PHP - Array Operators
- PHP - Conditional Operators
- PHP - Spread Operator
- PHP - Null Coalescing Operator
- PHP - Spaceship Operator
- PHP Control Statements
- PHP - Decision Making
- PHP - If…Else Statement
- PHP - Switch Statement
- PHP - Loop Types
- PHP - For Loop
- PHP - Foreach Loop
- PHP - While Loop
- PHP - Do…While Loop
- PHP - Break Statement
- PHP - Continue Statement
- PHP - Arrays
- PHP - Indexed Array
- PHP - Associative Array
- PHP - Multidimensional Array
- PHP - Array Functions
- PHP - Constant Arrays
- PHP Functions
- PHP - Functions
- PHP - Function Parameters
- PHP - Call by value
- PHP - Call by Reference
- PHP - Default Arguments
- PHP - Named Arguments
- PHP - Variable Arguments
- PHP - Returning Values
- PHP - Passing Functions
- PHP - Recursive Functions
- PHP - Type Hints
- PHP - Variable Scope
- PHP - Strict Typing
- PHP - Anonymous Functions
- PHP - Arrow Functions
- PHP - Variable Functions
- PHP - Local Variables
- PHP - Global Variables
- PHP Superglobals
- PHP - Superglobals
- PHP - $GLOBALS
- PHP - $_SERVER
- PHP - $_REQUEST
- PHP - $_POST
- PHP - $_GET
- PHP - $_FILES
- PHP - $_ENV
- PHP - $_COOKIE
- PHP - $_SESSION
- PHP File Handling
- PHP - File Handling
- PHP - Open File
- PHP - Read File
- PHP - Write File
- PHP - File Existence
- PHP - Download File
- PHP - Copy File
- PHP - Append File
- PHP - Delete File
- PHP - Handle CSV File
- PHP - File Permissions
- PHP - Create Directory
- PHP - Listing Files
- Object Oriented PHP
- PHP - Object Oriented Programming
- PHP - Classes and Objects
- PHP - Constructor and Destructor
- PHP - Access Modifiers
- PHP - Inheritance
- PHP - Class Constants
- PHP - Abstract Classes
- PHP - Interfaces
- PHP - Traits
- PHP - Static Methods
- PHP - Static Properties
- PHP - Namespaces
- PHP - Object Iteration
- PHP - Encapsulation
- PHP - Final Keyword
- PHP - Overloading
- PHP - Cloning Objects
- PHP - Anonymous Classes
- PHP Web Development
- PHP - Web Concepts
- PHP - Form Handling
- PHP - Form Validation
- PHP - Form Email/URL
- PHP - Complete Form
- PHP - File Inclusion
- PHP - GET & POST
- PHP - File Uploading
- PHP - Cookies
- PHP - Sessions
- PHP - Session Options
- PHP - Sending Emails
- PHP - Sanitize Input
- PHP - Post-Redirect-Get (PRG)
- PHP - Flash Messages
- PHP - AJAX Introduction
- PHP - AJAX Search
- PHP - AJAX XML Parser
- PHP - AJAX Auto Complete Search
- PHP - AJAX RSS Feed Example
- PHP - XML Introduction
- PHP - Simple XML Parser
- PHP - SAX Parser Example
- PHP - DOM Parser Example
- PHP Login Example
- PHP - Login Example
- PHP - Facebook and Paypal Integration
- PHP - Facebook Login
- PHP - Paypal Integration
- PHP - MySQL Login
- PHP Advanced
- PHP - MySQL
- PHP.INI File Configuration
PHP - Array Destructuring
- PHP - Coding Standard
- PHP - Regular Expression
- PHP - Error Handling
- PHP - Try…Catch
- PHP - Bugs Debugging
- PHP - For C Developers
- PHP - For PERL Developers
- PHP - Frameworks
- PHP - Core PHP vs Frame Works
- PHP - Design Patterns
- PHP - Filters
- PHP - Callbacks
- PHP - Exceptions
- PHP - Special Types
- PHP - Hashing
- PHP - Encryption
- PHP - is_null() Function
- PHP - System Calls
- PHP - HTTP Authentication
- PHP - Swapping Variables
- PHP - Closure::call()
- PHP - Filtered unserialize()
- PHP - IntlChar
- PHP - CSPRNG
- PHP - Expectations
- PHP - Use Statement
- PHP - Integer Division
- PHP - Deprecated Features
- PHP - Removed Extensions & SAPIs
- PHP - FastCGI Process
- PHP - PDO Extension
- PHP - Built-In Functions
- PHP Useful Resources
- PHP - Questions & Answers
- PHP - Quick Guide
- PHP - Useful Resources
- PHP - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
In PHP, the term Array destructuring refers to the mechanism of extracting the array elements into individual variables. It can also be called unpacking of array. PHP’s list() construct is used to destrucrure the given array assign its items to a list of variables in one statement.
As a result, val1 is assigned to $var1, val2 to $var2 and so on. Even though because of the parentheses, you may think list() is a function, but it’s not as it doesn’t have return value. PHP treats a string as an array, however it cannot be unpacked with list(). Moreover, the parenthesis in list() cannot be empty.
Instead of list(), you can also use the square brackets [] as a shortcut for destructuring the array.
Take a look at the following example −
It will produce the following output −
Destructuring an Associative Array
Before PHP 7.1.0, list() only worked on numerical arrays with numerical indices start at 0. PHP 7.1, array destructuring works with associative arrays as well.
Let us try to destructure (or unpack) the following associative array, an array with non-numeric indices.
To destructure this array the list() statement associates each array key with a independent variable.
Instead, you can also use the [] alternative destructuring notation.
Try and execute the following PHP script −
Skipping Array Elements
In case of an indexed array, you can skip some of its elements in assign only others to the required variables
In case of an associative array, since the indices are not incremental starting from 0, it is not necessary to follow the order of elements while assigning.
Destructuring a Nested Array
You can extend the concept of array destructuring to nested arrays as well. In the following example, the subarray nested inside is an indexed array.
Destructuring works well even if the nested array is also an associative array.
Destructuring Arrays in PHP: Everything You Need To Know
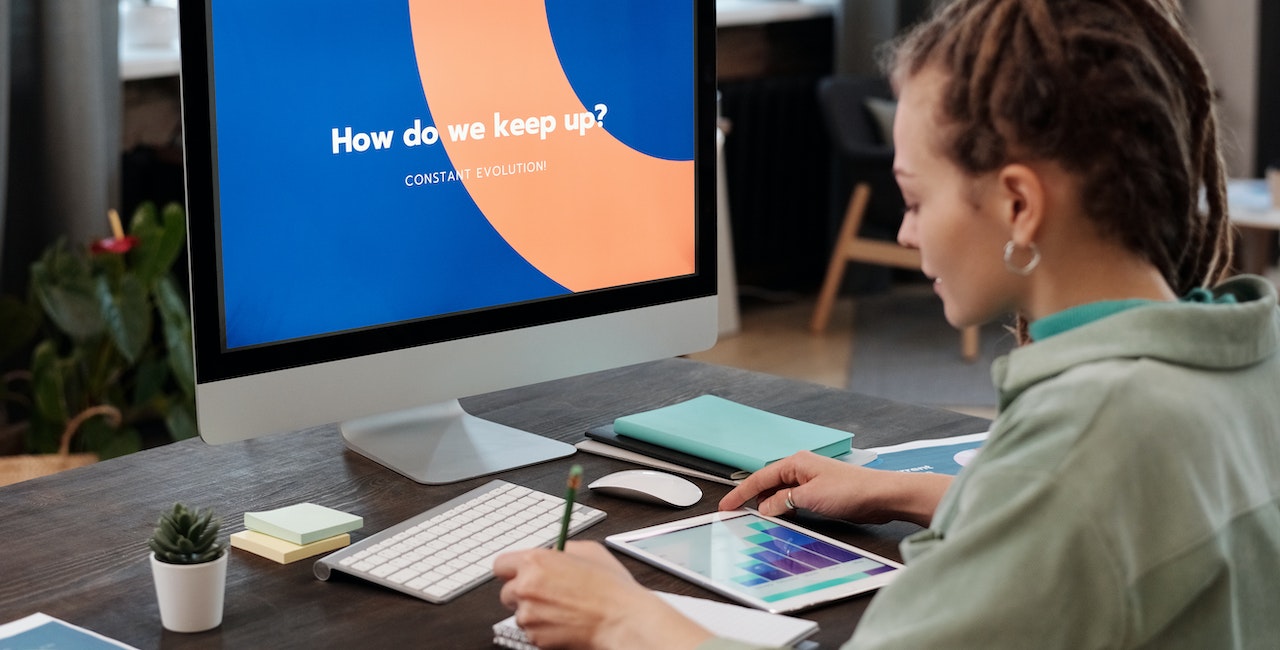
- Post author: Edward Stephen Jr.
- Post published: July 8, 2023
- Post category: Computer Programming / PHP
- Post comments: 0 Comments
Array destructuring is a powerful feature in PHP that allows you to extract individual elements from an array and assign them to separate variables. It simplifies the process of accessing and working with array elements by providing a concise and convenient syntax. This article covers array destructuring in PHP, exploring its syntax, use cases, and code examples to help you understand each concept thoroughly.
Table of Contents
What is Array Destructuring?
Array destructuring is a technique that allows you to unpack an array and assign its individual elements to separate variables. It provides a concise way to access array values without explicitly using indexes or accessing elements one by one. Array destructuring is available in PHP 7.1 and later versions.
Basic Array Destructuring
To perform array destructuring, you enclose the array within square brackets on the left side of the assignment operator (=). Then, you specify variables on the right side, separated by commas, to which the corresponding array elements will be assigned.
Skipping Array Elements
If you want to skip certain elements while destructuring an array, you can use an empty space (underscore ‘_’) as a placeholder.
Nested Array Destructuring
Array destructuring can be performed on nested arrays as well, allowing you to access elements within multidimensional arrays effortlessly.
Combining Array Destructuring with Function Returns
Array destructuring can be used in combination with function returns, allowing you to assign multiple values returned by a function to separate variables directly.
Array Destructuring with list()
In addition to using square brackets for array destructuring, PHP provides the list() function, which has been available since earlier versions of PHP. It allows you to achieve similar results but with a slightly different syntax.
Array destructuring is a powerful feature in PHP that simplifies working with arrays by unpacking their elements into individual variables. It provides a concise and convenient syntax, making your code more readable and reducing the need for explicit array element access. Whether it’s extracting elements from simple arrays or handling nested arrays and function returns, array destructuring offers a flexible and efficient solution.
By mastering array destructuring, you can enhance your PHP programming skills and write cleaner, more expressive code.
- Official PHP Documentation: Array Destructuring
I hope you found this information informative. If you would like to receive more content, please consider subscribing to our newsletter!
You Might Also Like
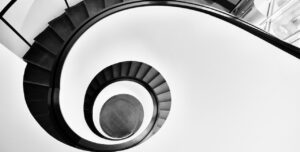
GoLang Looping: Everything You Need to Know
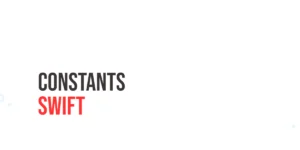
Swift Constants
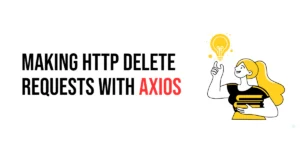
Handling HTTP DELETE Requests with Axios
Leave a reply cancel reply.
Save my name, email, and website in this browser for the next time I comment.

Amit Merchant Verified ($10/year for the domain)
A blog on PHP, JavaScript, and more
Array destructuring in PHP
October 30, 2018 · PHP
Folks who are familiar with the JavaScript’s ES6 may very well aware of the destructuring feature which allows us to extract data from arrays, objects, maps and sets. For instance, Using ES6 way, we can assign the top level variables of the objects to local variables using destructuring like below:
So, color and type has been assigned the variables from the Car object respectively. This is how object destructuring is achieved in JavaScript. What about PHP?
PHP >=7.1 has introduced associative array destructuring along with the list destructuring which was present in the earlier versions of PHP. Let’s take a very simple example. For earlier versions than 7.1, to assign the array values to the variables, you’d achieve using list() like this:
But from version 7.1, you can just use shorhand array syntax([]) to achieve the same:
As you can see, it’s just more cleaner and expressive now, just like ES6. Another area where you can use Array destructuring is in loops.
If you want to be specific about the key you assign to the variable, you can do it like this:
Wrapping up
Although, we have ‘list()’ already, utilizing the shorthand array syntax for destructuring is just more cleaner way and makes more sense.
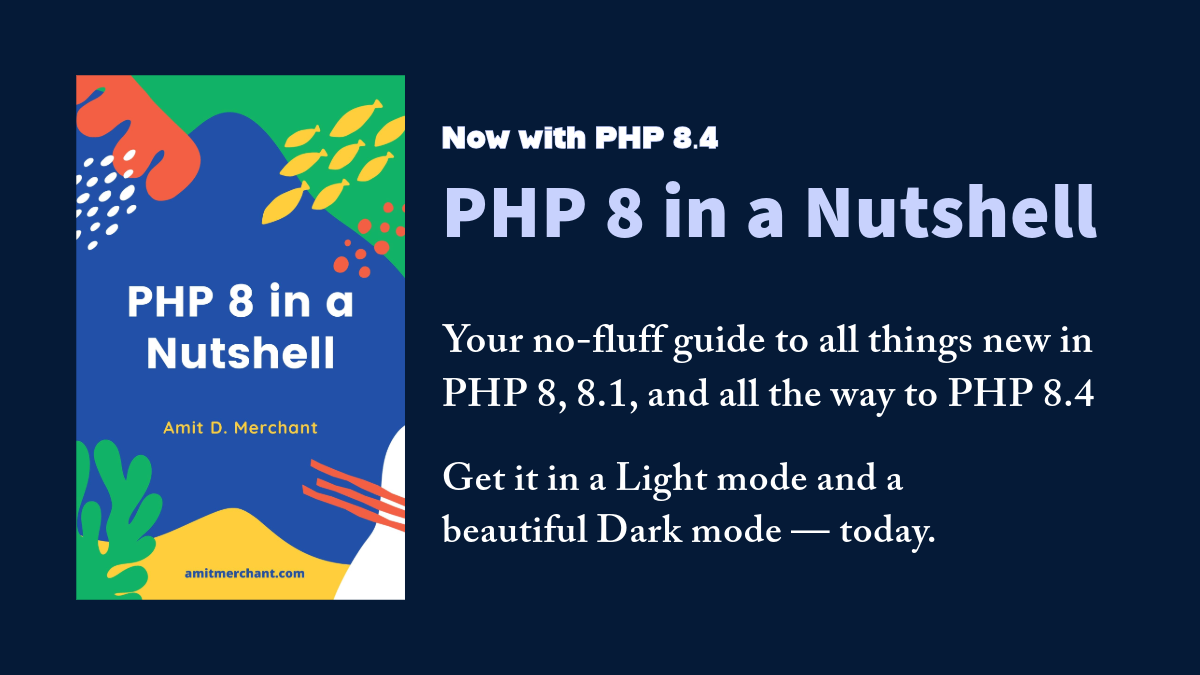
» Share: Twitter , Facebook , Hacker News
Like this article? Consider leaving a
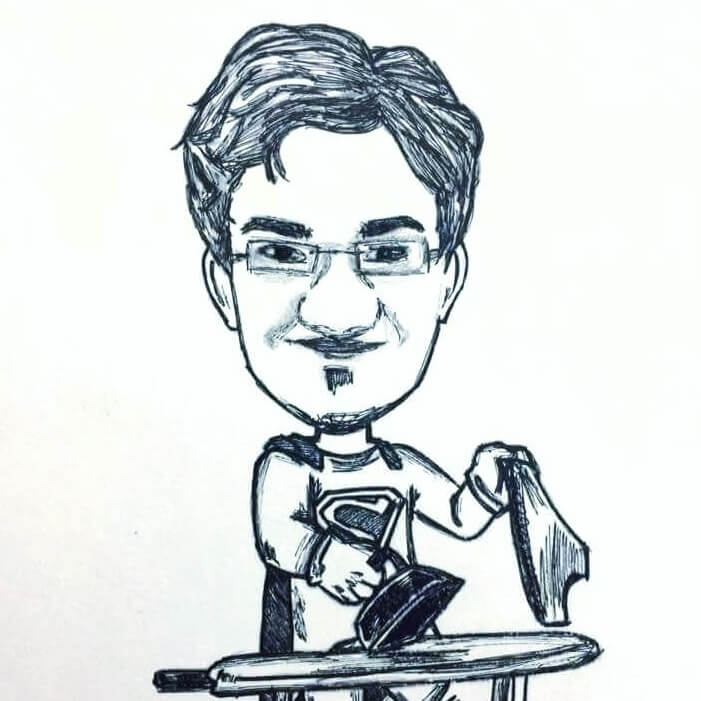
👋 Hi there! I'm Amit . I write articles about all things web development. You can become a sponsor on my blog to help me continue my writing journey and get your brand in front of thousands of eyes.
More on similar topics
Never run composer update on your server
A few new array functions are making their way into PHP 8.4
Scraping Google Web Search using ScraperAPI in PHP
Property hooks are coming in PHP 8.4
Awesome Sponsors
Download my eBook
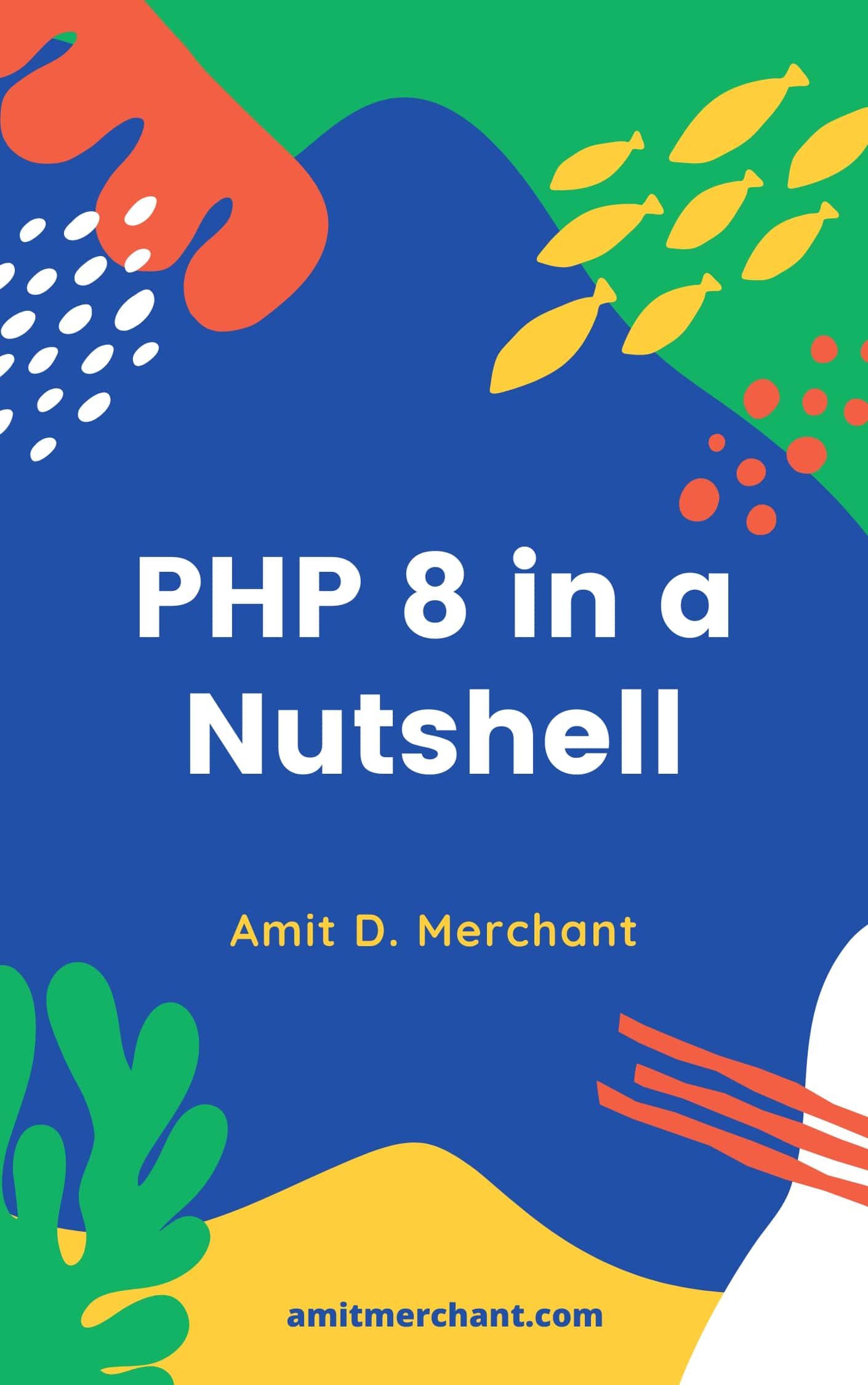
Get the latest articles delivered right to your inbox!
No spam guaranteed.
Follow me everywhere
More in "PHP"
How to return multi-line JavaScript code from PHP
Recently Published
Using Human-friendly dates in Git NEW
Keeping a check on queries in Laravel
Popover API 101
Request fingerprints and how to use them in Laravel
Separating Database Hosts to Optimize Read and Write Operations in Laravel
Top Categories
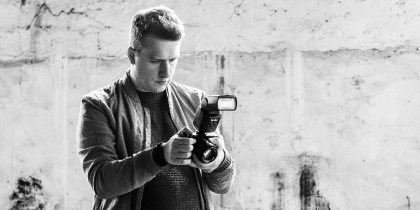
thoughts and inspiration on designing, programming, and writing for the web
The list function & practical uses of array destructuring in PHP
15 May 2017
PHP 7.1 introduced a new syntax for the list() function . I've never really seen too much list() calls in the wild, but it enables you to write some pretty neat stuff.
This post is a primer of list() and it's PHP 7.1 short notation, and an overview of some use cases I've been applying them to.
The list() construct (it's actually not a function but a language construct like array() ) has been around since PHP 4. list() allows you to pull variables from an array based on their index. Let's start with a quick primer!
Here's what a basic list() call looks like:
You can give list() less arguments than the array's size if you don't care about the rest of the items.
If you try to pull out an index that isn't set, an undefined offset error is thrown.
To skip an intermediate value, you can provide an empty argument.
As of PHP 7.1, you can specify the key of the item you want to unpack from an associative array. (RFC here )
Fun fact: since list() is an assignment operator, you could also assign things in arrays. Not saying this is particularly useful, but it's possible!
The list() operator is pretty cool, but has one caveat: it's ugly . PHP 7.1 fixes this with some syntactic sugar: plain old vanilla square brackets. These two statements do the same:
While it's not as powerful as JavaScript's array destructuring , it's still a cool tool to have baked in the language.
Now that we know how array destructuring works, let's move on to some practical examples.
Exhibit A: Some Classic Unpacking Examples
In this exhibit, we'll glide over some with some real world situations.
You've exploded a string and want to immediately assing the result as two seperate variables.
You've created a few objects at once, and want them all as their own variables.
You want to swap two variables.
Voila, nothing too fancy here, let's move over to some more specific use cases, borrowed from other languages.
Exhibit B: Tuples
The most concise definition of a tuple is a "data structure consisting of multiple parts" . Tuples are pretty much arrays with a fixed size and a predefined structure, for example a tuple of a status code and a message, a tuple of a longitude and a latitude, etc.
Tuples are useful in PHP when a key-value pair just doesn't cut it (maybe we need duplicate keys, or would want more that one value) and when we don't want to bother creating a value object.
Let's start with an array of some fruits and vegetables. Every piece of produce has an id , a name and a type .
Using the index to access the values requires us to reason about the array contents on every statement. Though process: "This is $item[0] , which means is the first item in the array. Next is $item[1] , which means it's the second item, etc."
By destructuring, we can immediately assign the values to a variable, making the contents of the loop clearer. Thought process: "I have an array containing entries that have an id, a name and a type."
Bonus snippet: we could use compact to create the array with a single statement (although I don't like compact because it's just as ugly as list() ) .
A more real world example: I recently had to make a pretty large form that only contained simple text inputs. I defined every input as a [$name, $value, $required] tuple and looped over all of them.
Further reading: Tuples in Python , Tuples in Elixir
Exhibit C: Multiple Returns
Some languages allow multiple returns. Consider a function that expects an integer, and returns two integers, one of them being $input + 5 , the other $input - 5 . Here's what that would look like in Go:
We could achieve something similar by returning an array in PHP and immediately destructuring:
One large downside here: there's currently no way to document this, neither with PHP 7's return types nor with PHPDoc (unless both returns have the same type, then you could hint with @return int[] ).
A more real world situation where multiple returns can be useful is cases where you're expecting a status and a message that you may or may not care about, like validation.
The previous example could also be interpreted as a tuple containing a status and a reason.
Further reading: Multiple returns in Go
That concludes today's presentation. I'll update the post in the future when I come accross other nifty use cases. Meanwhile, feel free to hit me up on Twitter if you're using list() in other cool ways!
Destructuring Assignments in PHP7
January 29, 2020
As with other programming languages, PHP also has a way to unpack arrays and objects. In this blog, we will look at destructuring assignments in PHP7.
PHP Array Destructuring:
There are functions available in PHP that allow you to unpack array values. These are,
The List is not function but a language construct like an array. It helps assign array values to multiple variables at a time. Before php7.1.0, list() supported only a numerically indexed array, however it now supports arrays of non-integer or non-sequential keys (associative array). Also, the list() function has a shorthand syntax ([]). The List function assigns values of an array on the right to a variable given as a parameter on the left side. Let’s see the use of the list function in array destructuring.
List function with a numerically indexed array
For numerically indexed arrays, the list function assumes that numerical indices start from 0 and that the keys are in sequence. While destructuring numerical keys array with the list function or shorthand [], the assignment of values to the variable is based on the index of values. The first value of the array will be assigned to the first variable given in the list function and so on. Performing destructuring on a numerically indexed array using the list function
Unpack all values
By providing a variable name for each value in the array we can easily unpack the array values.
Skip some values
To skip a value from the array does not state the variable declaration for that value. The list function will skip that particular value.
Swap variables
This requires a third variable when swapping two values, but list() or [] shorthand does it for you without any temporary variable to store the values during the swapping process.
List function with an associative array
For an associative array, the list() function variable can be assigned only for the keys that are available in the array. Unlike a numerically indexed array, you can assign array values to variables in any sequence by their key name. Performing destructuring on the associative array using a list,
To unpack all available values, we just need to assign a variable to each key of that array.
Assignment on the basis of the key name
Associative arrays do not need to maintain a variable assignment as per the array index. Key names can be used to assign variables for array values.
By simply not specifying the key on the left-hand we can skip the values of arrays that we do not wish to unpack.
In foreach loop
We can use the list function within a
The Extract Function
The extract() is an inbuilt function within PHP. It does not allow specifying variable names as it auto-generates the variable names that are the same as the keys of an array. It mostly works with an associative array as it uses the key name for creating variable names. A numerically indexed array will only be used with EXTR_PREFIX_ALL or EXTR_PREFIX_INVALID flags parameter. Flags are also helpful in many conditions such as managing invalid variable names, overwriting existing variables etc. extract() associative array
extract() numerically indexed array
Object Destructuring
In PHP, there is no object destructuring, but by converting an object into an associative array we can apply destructuring to it. To convert an object into an array we simply typecast it to an array or use the get_object_vars() function of PHP. Both methods return the properties of an object with their values in an associative array and any property with no assigned value is returned as NULL. In the following example we can see how we can destructure object properties:
If you liked this post, here are a few more that may interest you,
- A modern way of AJAX in WordPress using REST API
- Destructuring assignment in ES6 for more clarity
About Encora
Encora accelerates enterprise modernization and innovation through award-winning digital engineering across cloud, data, AI, and other strategic technologies. With robust nearshore and India-based capabilities, we help industry leaders and digital natives capture value through technology, human-centric design, and agile delivery.
Share this post
Case Studies
Learn More about Encora
We are the software development company fiercely committed and uniquely equipped to enable companies to do what they can’t do now.
Global Delivery
Related Insights
Approaching Agility in Non-Development Teams
Explore how agile methodologies can revolutionize non-development teams in various industries. ...
Boosting Productivity: Optimizing the Value Stream
Boosting financial companies' operational efficiency through Value Stream Mapping (VSM) practices. ...
Reimagine the Energy Supply Chain with Digital Twins
Discover how digital twins revolutionize energy and utilities, enhancing efficiency, ...

Accelerate Your Path to Market Leadership
Santa Clara, CA
+1 (480) 991 3635
Innovation Acceleration
Speak With an Expert
Strategic Services
Digital Engineering Services
Managed Services
All Insights
Partnerships
©Encora Digital LLC - Santa Clara, CA
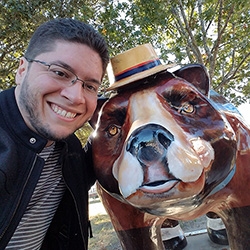
Frank Perez
Keep in touch
An experienced Web Developer with years of experience in design, programming and server administration.
© 2024 by Frank Perez.
Symmetric Array Destructuring in PHP
As of PHP 7.1, you can now use the shorthand array syntax to destructure your arrays for assignment. Previously you would have had to use a function like list, but now you can use the simple new array shorthand syntax.
Let’s look at some examples.
Basic Example
Example using loops.
PHP RFC: Square bracket syntax for array destructuring assignment
- Version: 1.0
- Date: 2016-04-07
- Authors: Andrea Faulds ajf@ajf.me , Bob Weinand bwoebi@php.net
- Status: Implemented (PHP 7.1)
- First Published at: http://wiki.php.net/rfc/short_list_syntax
The fundamental complex data type in PHP is the array . This data type is so frequently used that PHP has special syntax for handling it. This RFC focuses, in particular, on the syntax for two different array operations in PHP: construction of an array from values, and destructuring assignment from an array to variables.
Arrays can be constructed in PHP syntax using one of two syntax forms. The first of these, introduced in PHP 3, resembles a function call, where a series of comma-separated values (and optionally keys) is placed between array ( and ) :
The second syntax form, the so-called short array syntax introduced in PHP 5.4, is a more concise form that replaces array ( and ) with [ and ] :
Beyond being more concise, this second syntax has the benefit of not resembling a function call (preventing misunderstandings from new users), and being familiar to users of other languages like JavaScript, which use similar syntax for constructing arrays.
Similar to the array ( ) syntax for constructing arrays, PHP has had a syntax form for assigning to variables from array elements (“destructuring”) since PHP 3, where a series of comma-separated variables are placed between list ( and ) :
As of the upcoming PHP 7.1, there will also be a syntax form for specifying keys when destructuring :
However, while array ( ) has the more concise counterpart syntax [ ] , there is currently no such counterpart for list ( ) .
This RFC proposes introducing a second syntax form for destructuring assignment, where list ( and ) are replaced with [ and ] :
This syntax is more concise, and like the [ ] alternative to array ( ) , this new syntax does not resemble a function call.
Importantly, this syntax for destructuring an array means there is now symmetry between array construction and destructuring, which should make it clearer what the function of the syntax is:
This symmetry between construction and destructuring is a feature in some other languages. The following code, for example, is valid ECMAScript 6, and would behave identically in PHP:
The list ( ) syntax is not only permitted on the left-hand side of an assignment operation, but also as variable in a foreach loop. The new [ ] syntax for destructuring would likewise be permitted here:
Both due to implementation issues, and for consistency's sake, list ( ) cannot be nested inside [ ] , nor vice-versa:
Aside from this restriction, assignment with [ ] on the left-hand side behaves identically to list ( ) in all respects.
- Backward Incompatible Changes
- Proposed PHP Version(s)
This is proposed for the next minor or major version of PHP, whichever comes first. At the time of writing, this would be PHP 7.1.
This RFC has no impact upon OPcache or other extensions dealing with PHP opcodes, because the compiled result is identical to the list ( ) syntax.
This RFC would, however, impact upon projects which try to parse PHP syntax or inspect the PHP interpreter's abstract syntax tree.
- Open Issues
- Unaffected PHP Functionality
This RFC does not remove nor deprecate the existing list ( ) syntax, and it continues to function identically.
- Future Scope
We may wish to introduce destructuring assignment syntax for objects in future.
Because this proposal affects the language syntax (and also therefore the specification), it is a language change and requires at least a 2/3 majority to be accepted when put to a vote.
The vote will be a simple Yes/No vote on whether or not to accept the RFC for PHP 7.1 and merge the patch into master.
Voting started on 2016-04-27 and ended on 2016-05-08.
Accept the RFC Square bracket syntax for array destructuring assignment for PHP 7.1? | ||
---|---|---|
Real name | Yes | No |
(ajf) | ||
(beberlei) | ||
(bishop) | ||
(bwoebi) | ||
(cmb) | ||
(colinodell) | ||
(danack) | ||
(daverandom) | ||
(davey) | ||
(dragoonis) | ||
(francois) | ||
(galvao) | ||
(jhdxr) | ||
(jwage) | ||
(klaussilveira) | ||
(krakjoe) | ||
(lbarnaud) | ||
(leigh) | ||
(levim) | ||
(lstrojny) | ||
(marcio) | ||
(mariano) | ||
(mattwil) | ||
(mcmic) | ||
(nikic) | ||
(ocramius) | ||
(pauloelr) | ||
(pierrick) | ||
(pollita) | ||
(ralphschindler) | ||
(rasmus) | ||
(rmf) | ||
(sammyk) | ||
(santiagolizardo) | ||
(svpernova09) | ||
(thorstenr) | ||
(tpunt) | ||
(treffynnon) | ||
(trowski) | ||
(weierophinney) | ||
(yohgaki) | ||
This poll has been closed. |
- Patches and Tests
There is a pull request, with tests, for the PHP interpreter ( php-src ) here: https://github.com/php/php-src/pull/1849
There is not yet a patch or pull request for the language specification ( php-langspec ).
- Implementation
Merged into master for PHP 7.1: http://git.php.net/?p=php-src.git;a=commitdiff;h=4f077aee836ad7d8335cf62629a8364bdf939db9
Not yet in the language specification.
After the project is implemented, this section should contain
- a link to the PHP manual entry for the feature
- Nikita Popov's Abstract Syntax Tree RFC , which was accepted into PHP 7, noted that a short list syntax like this would not be possible without having the abstract syntax tree
- Rejected Features
Keep this updated with features that were discussed on the mail lists.
- Show pagesource
- Old revisions
- Back to top
Table of Contents

Destructuring in JavaScript – How to Destructure Arrays and Objects

Destructuring is a useful feature in JavaScript that allows you to easily unpack values from arrays or properties from objects into distinct variables. This can greatly simplify your code and make it more readable.
In this comprehensive guide, we will cover the basics of destructuring as well as some more advanced use cases. By the end, you‘ll have a solid grasp of how to take advantage of destructuring in your own code.
What is Destructuring?
Destructuring allows you to extract data from arrays and objects and assign them to variables with a syntax that mirrors the structure of the source data.
Here‘s a simple example of array destructuring:
Instead of accessing the array elements with fruits[0] and fruits[1] , you can assign them directly to variables first and second in one step.
And here‘s an example with object destructuring:
So destructuring neatly assigns object properties to variables firstName and lastName .
Some key advantages that destructuring provides:
- More readable and concise code compared to traditional property access
- Avoid having to come up with variable names like personFirstName
- Ability to pull just the properties you need into distinct variables
Keep reading to learn all about how destructuring works under the hood!
How Destructuring Works
Destructuring relies on some deeper JavaScript features:
Array matching
Positions in the array on the left side are matched with positions on the right side:
Object property matching
The shape of the object on the left matches the shape of the object on the right:
So the positions in arrays and names of properties create a pattern that is matched from left to right. The values are then unpacked and assigned to the variables.
Destructuring also works recursively:
So you can keep destructuring deeper into nested data structures.
One thing to note is that destructuring produces references to the original data, not copies. So if you modify a variable, you are changing the source array/object:
Destructuring Arrays
Destructuring is often used to extract elements from arrays. Let‘s explore some useful examples.
Pulling Parts of an Array
You can grab just the elements you need:
Omitting a position like , , will skip that element entirely. This provides a lot of flexibility in picking out array elements.
Swapping Variables
Array destructuring makes swapping variables easy:
No temporary variable needed!
Returning Multiple Values
You can return multiple values from a function using an array:
This keeps things tidy without needing to wrap the values in an object.
Destructuring Function Parameters
You can destructure incoming arguments into distinct variables:
This can clean up functions that take lots of configuration object parameters.
Ignoring Some Returned Values
If you only want some returned values, use a comma but don‘t declare a variable:
val1 gets 1, val3 gets 3, and the middle value is ignored.
Capturing Remaining Values
You can capture leftover values as their own array using the rest syntax:
This is useful when you want to split the first part but maintain the full set.
Parameter Default Values
You can assign defaults which are used if values are undefined:
So defaults provide a safer experience if values are missing.
Destructuring Objects
Destructuring is also frequently used for working with objects.
Unpacking Properties
You can unpack properties to local variables:
Much simpler than repeatedly accessing person.name and person.age !
Destructuring Renamed Properties
Sometimes the property names don‘t match the ideal variable names. You can rename them:
The syntax prop: var lets you customize the variable names.
Default Values
Object destructuring can also have default values just like arrays:
So first will get a default "Unknown" if no first property exists.
Nested Object Destructuring
You can chain together property dereferences:
This digs into objects recursively to get deeper values.
For…in Loops
destructuring also works nicely with for..in loops for iterating over objects:
So you immediately get access to key/value as variables on each loop iteration.
When to Avoid Destructuring
While destructuring can really clean up code when used properly, there are some downsides too:
- Overusing it can make code difficult to understand at a glance
- It pairs nicely with ES6 syntax but doesn‘t work in older browsers
- It may have minor performance implications in bottlenecks
- Poor naming loses context that plain objects provide
So avoid premature optimization with destructuring, and use it judiciously where it enhances readability.
Destructuring is a sweet feature in modern JavaScript, providing an easier way to access data in arrays, objects, and functions.
The key concepts include:
- Array destructuring with positions matched to variables
- Object destructuring with property names matched to variables
- Custom naming and default values
- Recursive destructure chaining
- Rest elements and ignored values
Once you grasp the basics from this article, consider digging deeper into real-world examples and advanced use cases to unlock the full potential of destructuring.
I hope this guide gives you the tools to start simplifying your JavaScript code! Let me know if you have any other destructuring tips.
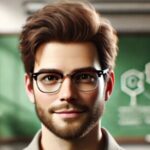
Dr. Alex Mitchell is a dedicated coding instructor with a deep passion for teaching and a wealth of experience in computer science education. As a university professor, Dr. Mitchell has played a pivotal role in shaping the coding skills of countless students, helping them navigate the intricate world of programming languages and software development.
Beyond the classroom, Dr. Mitchell is an active contributor to the freeCodeCamp community, where he regularly shares his expertise through tutorials, code examples, and practical insights. His teaching repertoire includes a wide range of languages and frameworks, such as Python, JavaScript, Next.js, and React, which he presents in an accessible and engaging manner.
Dr. Mitchell’s approach to teaching blends academic rigor with real-world applications, ensuring that his students not only understand the theory but also how to apply it effectively. His commitment to education and his ability to simplify complex topics have made him a respected figure in both the university and online learning communities.
Similar Posts

The Best Java 8 Tutorials: A Full-Stack Developer‘s Guide
As a full-stack developer who has worked extensively in Java since the initial release, Java 8…

How to Destructure Object Properties Using array.map() in React
Destructuring allows convenient access to object and array values without repetitive dot notation. Combined with the…

Demystifying the Difference Between Computer Science and Information Technology
As our world grows increasingly digital and technology-dependent, two related fields stand at the center of…

Amazon Web Services Explained by Operating a Brewery
If you understand how a brewery works, you can understand Amazon Web Services (AWS). Photo by…

Deep Copy vs Shallow Copy in Swift: A Complete Guide
Copying objects is a fundamental concept in programming. Whether you‘re working in Swift, Java, Python or…

8 Ways AI Makes Virtual & Augmented Reality More Immersive
Virtual reality (VR) and augmented reality (AR) aim to immerse users in digital worlds and enhance…
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
Get unique values from a column in a 2d array
I have a 2d array and need to isolate the unique columns from one column.
How do I add elements to the result array only if they aren't in there already? I have the following:
Instead, I want $a to consist of the unique values. (I know I can use array_unique() after looping to get the desired results, but I just want to know of any other ways.)
- multidimensional-array

- For those searching for Laravel solution, the Arr::wrap() is the answer, see docs . – peter.babic Commented Jul 9, 2022 at 7:00
16 Answers 16
You should use the PHP function in_array (see http://php.net/manual/en/function.in-array.php ).
This is what the documentation says about in_array :
Returns TRUE if needle is found in the array, FALSE otherwise.

- Making iterated value-based checks is likely to be the least performant process. – mickmackusa Commented yesterday
You'd have to check each value against in_array :
- 4 As the volume of the input data increases, in_array() will experience increasingly worse performance. – mickmackusa Commented Sep 14, 2020 at 23:14
Since you seem to only have scalar values an PHP’s array is rather a hash map, you could use the value as key to avoid duplicates and associate the $k keys to them to be able to get the original values:
Then you just need to iterate it to get the original values:
This is probably not the most obvious and most comprehensive approach but it is very efficient as it is in O( n ).
Using in_array instead like others suggested is probably more intuitive. But you would end up with an algorithm in O( n 2 ) ( in_array is in O( n )) that is not applicable. Even pushing all values in the array and using array_unique on it would be better than in_array ( array_unique sorts the values in O( n ·log n ) and then removes successive duplicates).
- There's a suggested edit by @xmedeko that suggests that the cost is O(n logn) not O(n) because of "the cost on PHP hashing", could both of you reach an agreement here? :-) – Kos Commented Nov 22, 2012 at 12:33
- IMHO array_unique preserves the original order values, while your solutions doesn't. – xmedeko Commented Nov 22, 2012 at 12:33
Easy to write, but not the most effective one:
This one is probably faster:

- Great solution, Thanks – Malus Jan Commented Nov 1, 2017 at 12:57
- 1 According to my IDE (PHPStorm, since I have not done performance comparison) the first one is faster. PHPStorm gave me a "resource intensive operation used in loop" warning when using array_merge + array_diff. – Catar4 Commented Jan 6, 2020 at 19:04
Try adding as key instead of value:
Adding an entry
Getting all entries
- This is how Java implements HashSets (with an embedded HashMap and a value, I think consisting of a static new Object() ) – Erk Commented Nov 7, 2018 at 0:35
If you're okay with using shorthands in your code (instead of writing explicit if blocks, like some coding standards recommend), you can further simplify Marius Schulz's answer with this one-liner:

If you don't care about the ordering of the keys, you could do the following:

Since there are a ton of ways to accomplish the desired results and so many people provided !in_array() as an answer, and the OP already mentions the use of array_unique , I would like to provide a couple alternatives.
Using array_diff (php >= 4.0.1 || 5) you can filter out only the new array values that don't exist. Alternatively you can also compare the keys and values with array_diff_assoc . http://php.net/manual/en/function.array-diff.php
http://ideone.com/SWO3D1
Another method is using array_flip to assign the values as keys and compare them using isset , which will perform much faster than in_array with large datasets. Again this filters out just the new values that do not already exist in the current values.
http://ideone.com/cyRyzN
With either method you can then use array_merge to append the unique new values to your current values.
- http://ideone.com/JCakmR
- http://ideone.com/bwTz2u

you can try using "in_array":
Taking Gumbo's idea , making the code work:
Gives this:
With array_flip() it could look like this:
With array_unique() - like this:
Notice that array_values(...) — you need it if you're exporting array to JavaScript in JSON form. array_unique() alone would simply unset duplicate keys, without rebuilding the remaining elements'. So, after converting to JSON this would produce object, instead of array.
To populate a flat, indexed array of unique values from a single column of scalar values from a 2d array, you can use array_column() and nominate the 2nd and 3rd parameters as the same desired column.
Because PHP doesn't allow keys on the same level of an array to be duplicated, you get the desired unique value results.
This technique is reliable because none of the values being isolated will be mutated by PHP upon being used as keys -- this is not true of nulls, floats, booleans, and more data types.
array_values() is only necessary if you want to re-index the keys; otherwise you can leave the temporary keys and avoid the extra function call.
Code: ( Demo )
For the implementation of a language construct for iteration, you can use a body-less foreach() and access the key column value twice to push associative values into the result array. Demo
Topical reading: While destructuring an array, can the same element value be accessed more than once?
You can also use +=
will result in $options1 equal to:
will result in $options2 equal to:
Try this code, I got it from here
Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged php arrays multidimensional-array filtering unique or ask your own question .
- The Overflow Blog
- Battling ticket bots and untangling taxes at the frontiers of e-commerce
- Ryan Dahl explains why Deno had to evolve with version 2.0
- Featured on Meta
- We've made changes to our Terms of Service & Privacy Policy - July 2024
- Bringing clarity to status tag usage on meta sites
- Feedback requested: How do you use tag hover descriptions for curating and do...
Hot Network Questions
- Trigger (after delete) restricts from deleting records
- What should I [34/F] do about my rude and mean supervisor [60s/F] when I want to finish my PhD?
- Difference of infinite measures
- What is this surface feature near Shackleton crater classified as?
- Who is affected by Obscured areas?
- Find all pairs of positive compatible numbers less than 100 by proof
- What prevents applications from misusing private keys?
- How can I understand op-amp operation modes?
- A possibly Varley short fiction about a clone, his sister-progenitor and a complicated family history
- Is there a way to search by Middle Chinese rime?
- Why is the identity of the actor voicing Spider-Man kept secret even in the commentary?
- Print lines between two patterns where first pattern appears more than once before second pattern
- Why would an incumbent politician or party need to be re-elected to fulfill a campaign promise?
- Seven different digits are placed in a row. The products of the first 3, middle 3 and last 3 are all equal. What is the middle digit?
- Ecuador: what not to take into the rainforest due to humidity?
- Is "UN law" a thing?
- Multiple unds in a sentence
- For applying to a STEM research position at a U.S. research university, should a resume include a photo?
- Momentary solo circuit
- No Kippa while in a car
- Is this misleading "convenience fee" legal?
- What did Scott Lang mean by "living as a tenant of the state"?
- Shape functions on the triangle using vertex values and derivatives
- What might cause these striations in this solder joint?

IMAGES
COMMENTS
As a follow up, What's so wrong with extract: it can put unsanitized, unknown variables in the global (or local function) namespace, or overwrite your own variables.It can also lead other programmers to wonder where a variable introduced this way came into play. Basically, use wisely only when sure of the data coming in is safe, and you are aware of all variables that could be introduced.
Summary: in this tutorial, you'll learn how to use PHP array destructuring to assign elements of an array to multiple variables. ... Let's take some examples of using the PHP array destructuring syntax. 1) Swaping variables. To swap the values of two variables, you often use a temporary variable like this: ...
PHP 7.1 array super-powers! In PHP 7.1 the array type has become even more powerful! An RFC was accepted (and implemented) to provide square bracket syntax for array destructuring assignment. This change to the language made it possible to extract values from array more easily. For example, consider the following options array:
Array destructuring in PHP # List or [] In PHP, list or [] is a so called "language construct", just like array(). This language construct is used to "pull" variables out of an array. In other words: it will "destructure" the array into separate variables. Note that the word is "destructure", not "destruction" — that's something different 😉
However, with the advent of version 8.0, we now have two ways. They are as follows: The [] notation (introduced in PHP 8.0) The list() construct. We'll focus on the former, i.e. using [], since it's syntactically more concise. All the ideas that apply to destructuring via [] also apply to destructuring via list().
Arrays. ¶. An array in PHP is actually an ordered map. A map is a type that associates values to keys. This type is optimized for several different uses; it can be treated as an array, list (vector), hash table (an implementation of a map), dictionary, collection, stack, queue, and probably more. As array values can be other array s, trees and ...
Before PHP 7.1.0, the list () construct only worked on indexed arrays. To know the version of PHP installed on your server, run the code below: echo PHP_VERSION . PHP_EOL; The PHP_VERSION constant gives you the version number, PHP_EOL is only ending the line for us. Consider the following code:
Destructuring Arrays. In PHP, array destructuring, also known as array unpacking, is a feature that allows you to assign elements of an array or properties of an object to separate variables in a single statement. It's a handy way to extract multiple values from an array or object at once. The problem. Consider the following array:
PHP - Array Destructuring. In PHP, the term Array destructuring refers to the mechanism of extracting the array elements into individual variables. It can also be called unpacking of array. PHP's list () construct is used to destrucrure the given array assign its items to a list of variables in one statement.
Array destructuring is a technique that allows you to unpack an array and assign its individual elements to separate variables. It provides a concise way to access array values without explicitly using indexes or accessing elements one by one. Array destructuring is available in PHP 7.1 and later versions. Basic Array Destructuring
Array destructuring in PHP. Folks who are familiar with the JavaScript's ES6 may very well aware of the destructuring feature which allows us to extract data from arrays, objects, maps and sets. For instance, Using ES6 way, we can assign the top level variables of the objects to local variables using destructuring like below: So, color and ...
When you need to pull values from arrays, destructuring often makes sense. In this course, we'll dive into everything you need to know about destructuring ar...
PHP 7.1 introduced a new syntax for the list() function.I've never really seen too much list() calls in the wild, but it enables you to write some pretty neat stuff.. This post is a primer of list() and it's PHP 7.1 short notation, and an overview of some use cases I've been applying them to.. list() 101 The list() construct (it's actually not a function but a language construct like array ...
In this blog, we will look at destructuring assignments in PHP7. PHP Array Destructuring: There are functions available in PHP that allow you to unpack array values. These are, List; Extract; List. The List is not function but a language construct like an array. It helps assign array values to multiple variables at a time.
This data can often be handled in an elegant way using the existing capabilities of array destructuring. While simple variable assignments can be casted during the assignment this isn't possible during assignments via array destructuring. This proposal adds a new syntax for casting in array assignments.
Symmetric Array Destructuring in PHP. As of PHP 7.1, you can now use the shorthand array syntax to destructure your arrays for assignment. Previously you would have had to use a function like list, but now you can use the simple new array shorthand syntax. As of PHP 7.1, you can now use the shorthand array syntax to destructure your arrays for ...
This RFC focuses, in particular, on the syntax for two different array operations in PHP: construction of an array from values, and destructuring assignment from an array to variables. Arrays can be constructed in PHP syntax using one of two syntax forms.
Indeed, it is listed as a new feature in PHP 7.1: The shorthand array syntax ( []) may now be used to destructure arrays for assignments (including within foreach ), as an alternative to the existing list() syntax, which is still supported. The new shorthand also supports destructuring arrays with string keys by key rather than by order, a very ...
Destructuring assignment; Nested destructuring; Destructuring maps; Common destructuring mistakes; Smart ways to use destructuring; Tips and best practices; Conclusion; Let's dive in! What is Destructuring? Destructuring allows you to unpack values from arrays, objects, or maps into distinct variables. Here is a common example:
Since PHP7.1, a foreach() expression can implement array destructuring as a way of unpacking row values and make individualized variable assignments. When using array destructuring within a foreach() loop, can a specific value be accessed by its associative key more than once? For example:
Destructuring is a sweet feature in modern JavaScript, providing an easier way to access data in arrays, objects, and functions. The key concepts include: Array destructuring with positions matched to variables; Object destructuring with property names matched to variables; Custom naming and default values; Recursive destructure chaining
I want to perform Destructuring in php just like in javascript code below: [a, b, ...rest] = [10, 20, 30, 40, 50]; console.log(a,b,rest); ... PHP will not allow you to unpack a dynamic amount of data using the left side of the assignment, so for a fully dynamic one-liner, use the right side of the assignment to prepare/group the data after the ...
Since PHP7.1, a foreach() expression can implement array destructuring as a way of unpacking row values and make individualized variable assignments for later use. When using array destructuring within the head/signature of a foreach() loop, can new elements be declared instead of merely being accessed? For example:
Since there are a ton of ways to accomplish the desired results and so many people provided !in_array() as an answer, and the OP already mentions the use of array_unique, I would like to provide a couple alternatives.. Using array_diff (php >= 4.0.1 || 5) you can filter out only the new array values that don't exist. Alternatively you can also compare the keys and values with array_diff_assoc.