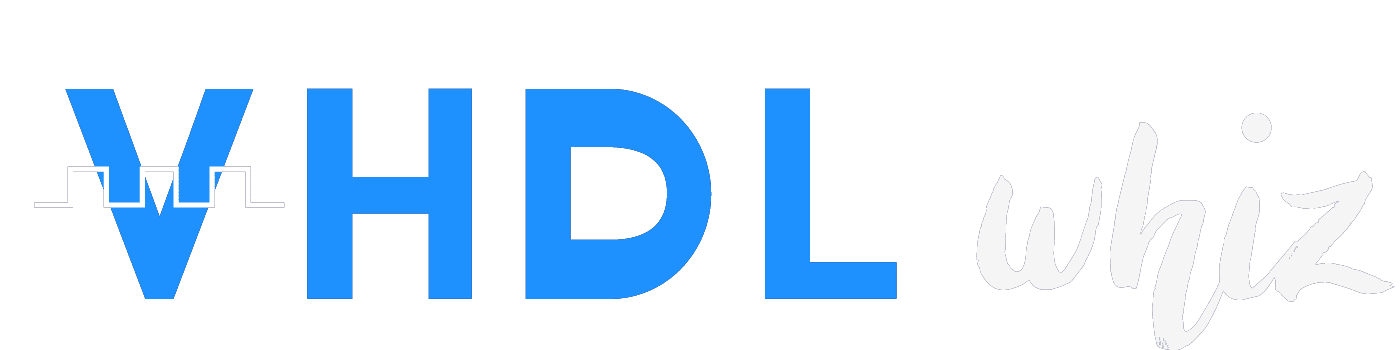

How to create a signal vector in VHDL: std_logic_vector
The std_logic_vector type can be used for creating signal buses in VHDL. The std_logic is the most commonly used type in VHDL, and the std_logic_vector is the array version of it.
While the std_logic is great for modeling the value that can be carried by a single wire, it’s not very practical for implementing collections of wires going to or from components. The std_logic_vector is a composite type, which means that it’s a collection of subelements. Signals or variables of the std_logic_vector type can contain an arbitrary number of std_logic elements.
This blog post is part of the Basic VHDL Tutorials series.
The syntax for declaring std_logic_vector signals is:
where <name> is an arbitrary name for the signal and <initial_value> is an optional initial value. The <lsb> is the index of the least significant bit, and <msb> is the index of the most significant bit.
The to or downto specifies the direction of the range of the bus, basically its endianness. Although both work equally well, it’s most common for VHDL designers to declare vectors using downto . Therefore, I recommend that you always use downto when you declare bit vectors to avoid confusion.
The VHDL code for declaring a vector signal that can hold a byte:
The VHDL code for declaring a vector signal that can hold one bit:
The VHDL code for declaring a vector signal that can hold zero bits (an empty range ):
In this video tutorial, we will learn how to declare std_logic_vector signals and give them initial values. We also learn how to iterate over the bits in a vector using a For-Loop to create a shift register :
The final code we created in this tutorial:
The waveform window in ModelSim after we pressed run, and zoomed in on the timeline:
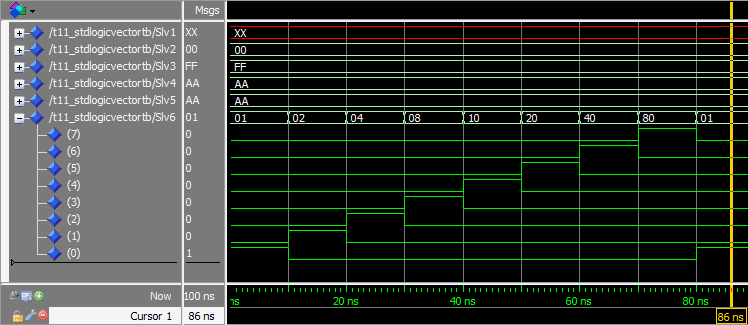
Let me send you a Zip with everything you need to get started in 30 seconds
Unsubscribe at any time
In this exercise we declared six std_logic_vector buses, each eight bits long (one byte).
Signal Slv1 was declared without a initial value. The bus is seen having the value XX in the waveform screenshot. This is because the value that is displayed on the bus is in hexadecimals, and XX indicates a non-hex value. But when we expanded the bus in the waveform, we could see that the individual bits were indeed U’s.
Signal Slv2 was declared using an initial value of all 0’s. Instead of specifying the exact value for each bit, we used (other => '0') in place of the initial value. This is known as an aggregate assignment. The important part is that it will set all bits in the vector to whatever you specify, no matter how long it is.
Signal Slv3 was declared using an aggregate assignment to give all bits the initial value of 1. We can see FF displayed on this signal in the waveform, which is hex for eight 1’s.
Signal Slv4 was declared with an initial value specified in hex, AA. Each hex digit is 4 bits long, therefore we must supply two digits (AA) for our vector which is 8 bits long.
Signal Slv5 declares exactly the same initial value as Slv4 , but now we specified it as the binary value 10101010. We can see from the waveform that both signals have the hex value AA.
Signal Slv6 was declared with an initial value of all zeros, except for the rightmost bit which was '1' . We used a process to create a shift register from this signal. The shift register, as the name implies, shifts the contents of the vector one place to the left every 10 nanoseconds.
Our process wakes up every 10 ns, and the For-Loop shifts all bits in the vector one place to the left. The final bit is shifted back into the first index by the Slv6(Slv6'right) <= Slv6(Slv6'left); statement. In the waveform we can see the '1' ripple through the vector.
By using the 'left' and 'right attributes, we made our code more generic. If we change the width of Sig6 , the process will still work. It’s good design practice to use attributes where you can instead of hardcoding values.
You may be wondering if there are more attributes that you can use, and there are . I won’t be talking more about them in this tutorial series, because I consider them to be advanced VHDL features.
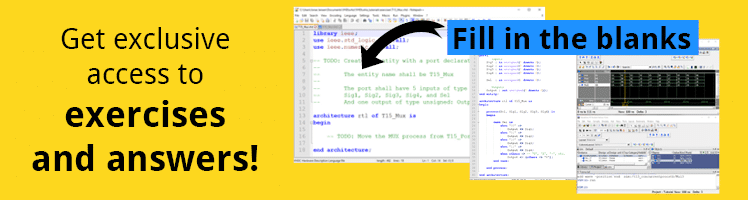
- N-bit vectors should be declared using std_logic_vector(N-1 downto 0)
- A vector can be assigned as a whole or bits within it can be accessed individually
- All bits in a vector can be zeroed by using the aggregate assignment (others => '0')
- Code can be made more generic by using attributes like 'left and 'right
Take the Basic VHDL Quiz – part 2 » or Go to the next tutorial »
I’m from Norway, but I live in Bangkok, Thailand. Before I started VHDLwhiz, I worked as an FPGA engineer in the defense industry. I earned my master’s degree in informatics at the University of Oslo.
Similar Posts
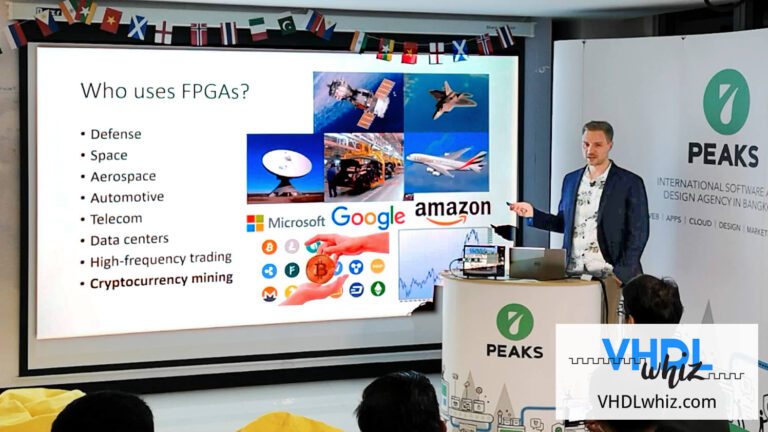
An Introduction to FPGAs & Programmable Logic
This video is an introductory presentation about FPGA and programmable logic technology. I delivered this 45 minutes talk at an event hosted by 7 Peaks Software in Bangkok, Thailand, on November 19th, 2019.
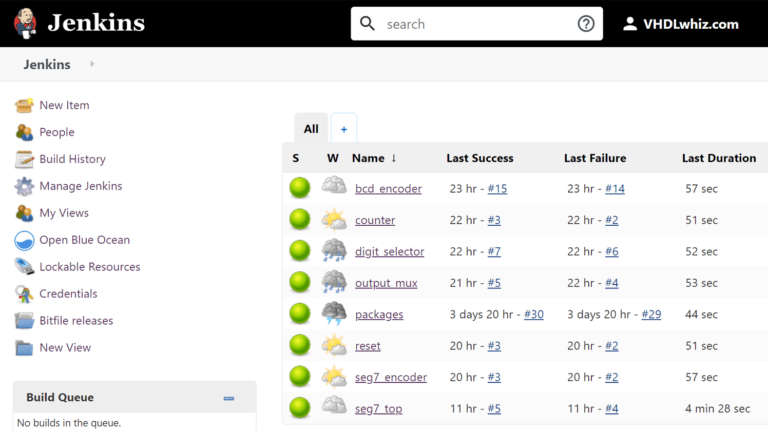
Jenkins for FPGA projects using Vivado and GitHub on a Linux VPS
This tutorial teaches you how to set up an automation server on a Virtual Private Server (VPS) using Jenkins, Xilinx Vivado, and the Git / GitHub source-control management (SCM) system.
Jenkins can be a valuable tool also for FPGA teams. Automating tasks can save your company time and improve the quality of your code. By using automatic build triggers and automated job pipelines, fewer coding errors will go unnoticed.
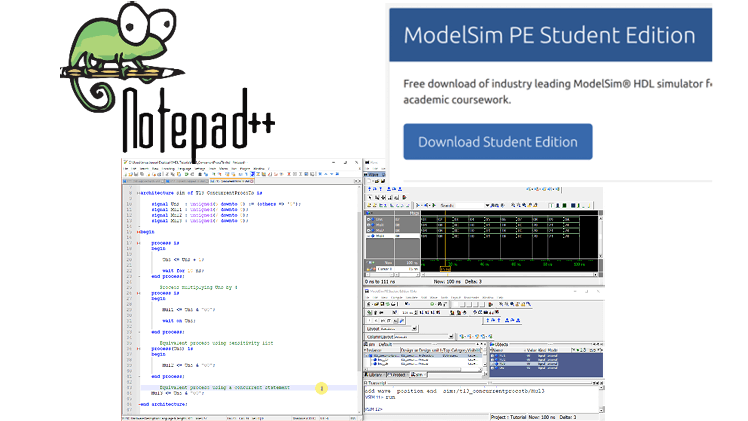
How to install a VHDL simulator and editor for free
Although VHDL and FPGA tools are often very expensive, it is easy to access state-of-the-art software for free if you are a student. There are several simulators, editors, and IDEs for working with VHDL. This article shows you how to install two of the most popular programs used by VHDL engineers. VHDL simulator Siemens EDA’s…
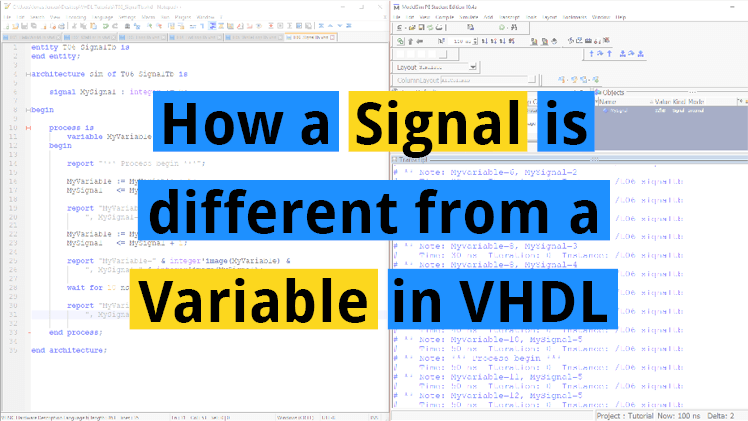
How a signal is different from a variable in VHDL
In the previous tutorial we learned how to declare a variable in a process. Variables are good for creating algorithms within a process, but they are not accessible to the outside world. If a scope of a variable is only within a single process, how can it interact with any other logic? The solution for…
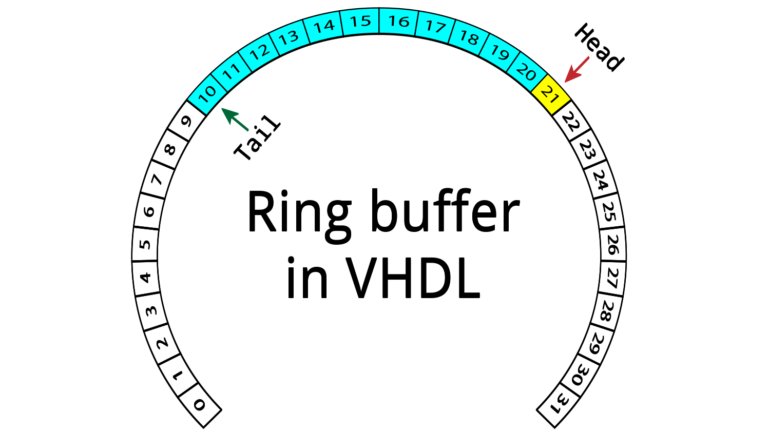

How to create a ring buffer FIFO in VHDL
Circular buffers are popular constructs for creating queues in sequential programming languages, but they can also be implemented in hardware. In this article, we will create a ring buffer in VHDL to implement a FIFO in block RAM. There are many design decisions you will have to make when implementing a FIFO. What kind of…
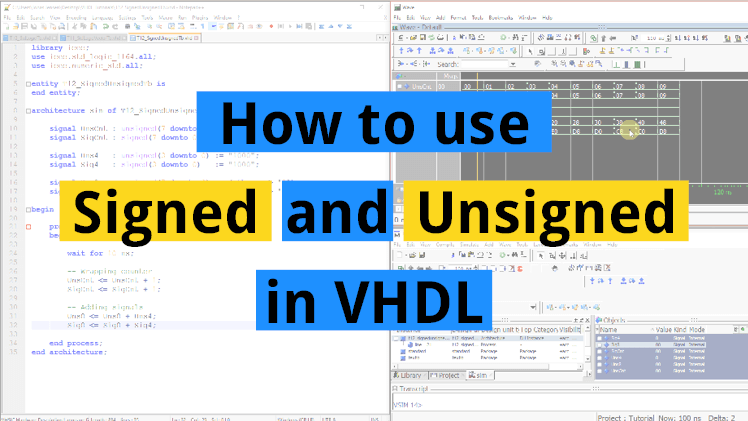
How to use Signed and Unsigned in VHDL
The signed and unsigned types in VHDL are bit vectors, just like the std_logic_vector type. The difference is that while the std_logic_vector is great for implementing data buses, it’s useless for performing arithmetic operations. If you try to add any number to a std_logic_vector type, ModelSim will produce the compilation error: No feasible entries for…
18 Comments
Hi! Excellent article. I have a question, why would you ever use std_logic_vector(0 downto 0) instead of just std_logic?
I have seen std_logic_vector(0 downto 0) being used for mapping a std_logic to a module that has a vector with generic width as an input. Consider this port declaration:
If we want to use a std_logic as input here, we need to first convert it to a std_logic_vector .
In deep logic where generics are used, you may also encounter the zero-width bus. That is, when generics causes a bus to evaluate to this: std_logic_vector(-1 downto 0) . It is legal, but it is also a source of synthesis warnings. For example this warning message from Vivado:
"WARNING: [Synth 8-3919] null assignment ignored"
Hey, I would like to thank you for these tutorials, it not easy to find short and well-explained VHDL lessons nowadays.
I’m glad you found the tutorials helpful. Stay tuned for more advanced topics about VHDL on the blog in the upcoming weeks and months.
Hi sir, i want to take values to array of bit vector from my input bit stream how can i assign? i can not do it by slicing of my array and assigning input value. then how can i do the same
Hi Archana,
To deserialize data that is arriving over a one-bit interface, you can clock the data into a shift register like I’m doing in this blog post. Read out the data word (or byte) when the shift register is full.
The other method is to assign the received bits directly to the correct index in the receive buffer.
Either way, you will have to count the number of received bits to keep track of the position within the received word. You should implement a simple state machine with a counter to take care of this.
Thank you sir..
I have a signal of type “” type ram is array( 0 to 15) of bit_vector(3 down to 0);”” and i have to read elements from text file to this . how can i do this? i am using Textio library. now i am doing it by creating individual 16 signals of bit_vector(3 down to 0) and read the file text to this from the variable and then assigning four such signal to input of type ram is array( 0 to 15) of bit_vector(3 down to 0); like,
how can i do the same by avoiding these steps?
Oh, I see. You want to initialize a RAM from a text file. In the example below, I am initializing your RAM in the declarative region of the VHDL file by using an impure function.
I’ve used your types and signal names. First, we declare the type. Then, we declare an impure function which reads the bits from the data.txt file. Finally, we use the return value from the function as an initial value for the indata1 RAM signal.
Thank You Sir.
I would also like to say that your website has been very helpful! Thanks for all of your efforts on the website and also for any input on my question below, if you have time.
Is it possible to create a port with mixed inputs and outputs other than using inout? That generates a lot of warnings which doesn’t hurt anything however I usually try to avoid warning.
Hello Steve,
A common way to handle this is to declare a local copy of the output signal. Look at the example below to see what I mean.
Thanks for your reply!
I was hoping to have a single “Bus name” in the entity port list with some of the signals in the bus being inputs and others being outputs.
I might be misinterpreting your response or my question might not be clear. Either way, thanks for your time and if you have any additional suggestions, feel free to post them but if not, no worries. Warnings really don’t matter but I prefer to avoid them.
For example: – CPLD_B2:26 – CPLD_B2:01 are dedicated outputs – CPLD_B2:27 is a dedicated input.
I can assign them “inout” but warnings are generated as follows:
Following 27 pins have no output enable or a GND or VCC output enable Info (169065): Pin CPLD_B2[1] has a permanently enabled output enable File.
Oh, I see. That’s only possible in VHDL-2019. Unfortunately, not all FPGA tools support the newest VHDL revision yet. You can read about the change here: https://vhdlwhiz.com/vhdl-2019/#interfaces
Thanks and keep up the great website!
Could you shed some light on toggling multiple bits in a logic vector at the same time?
Hello Sankalp,
You can use a For loop to flip every bit in the vector:
Would this vhdl code be on an infinite loop? Continuously shifting the 1 left?
Hello Jareb,
I wouldn’t consider the shift register process to be an infinite loop because there is a wait for 10 ns; statement inside of it. But I guess from a software perspective, it is an infinite loop because it never stops.
After the simulator executes the last line of a process without a sensitivity list, it immediately continues from the first line again. That’s why we always need to have a Wait statement inside of the process, or we have to use a sensitivity list.
Try to remove the Wait statement from the process and simulate! You will see ModelSim complaining because it’s an infinite loop that continues without any time passing.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Notify me of replies to my comment via email
Most logic synthesis tools accept one-dimensional arrays of other supported types. 1-D arrays of 1-D arrays are often supported. Some tols also allow true 2-D arrays, but not more dimensions.
Note that arrays are usually implemented using gates and flip-flops, not ROM's and RAM's.
Array types have not changed in VHDL -93.
Success! Subscription added.
Success! Subscription removed.
Sorry, you must verify to complete this action. Please click the verification link in your email. You may re-send via your profile .
- Intel Community
- Product Support Forums
- Programmable Devices
Quick VHDL question about splitting/joining std_logic_vectors
- Subscribe to RSS Feed
- Mark Topic as New
- Mark Topic as Read
- Float this Topic for Current User
- Printer Friendly Page
- Mark as New
- Report Inappropriate Content
- All forum topics
- Previous topic
Link Copied

Community support is provided during standard business hours (Monday to Friday 7AM - 5PM PST). Other contact methods are available here .
Intel does not verify all solutions, including but not limited to any file transfers that may appear in this community. Accordingly, Intel disclaims all express and implied warranties, including without limitation, the implied warranties of merchantability, fitness for a particular purpose, and non-infringement, as well as any warranty arising from course of performance, course of dealing, or usage in trade.
For more complete information about compiler optimizations, see our Optimization Notice .
- ©Intel Corporation
- Terms of Use
- *Trademarks
- Supply Chain Transparency
jliu83 (Member) 已询问问题。
- process ( clk ) begin
- if rising_edge ( clk ) then
- -- multiply , truncate then assign
- res <= ( a * b )( 15 downto 8 );
- end process ;
- General Discussion
hgleamon1 (Member)
jliu83 (Member)

bassman59 (Member)
> -------------------------------------------------------------------------------- > > @jliu83 wrote: > > > Hi, I was wondering if anyone with a greater understanding of VHDL syntax can > help me with this. > > > > I am using "use IEEE.std_logic_unsigned.all;" so that all my > STD_LOGIC_VECTOR's are treated as unsigned numbers. > > > > --------------------------------------------------------------------------------
That's a mistake.
> -------------------------------------------------------------------------------- > > @jliu83 wrote: > > > Thank you. I guess a few extra registers in the pipeline doesn't hurt. On > the subject of the libraries, numeric_std would require manual casting of all > incoming signals from vectors to unsigned. Any tricks around this tedious > task? If doing something like bicubic interpolation, that's a lot of casts > (to and from unsigned). > > > > -J > > --------------------------------------------------------------------------------
The simple answer is that ports don't need to be std_logic_vector. You can declare ports as unsigned or signed. It works. Keep the signals as unsigned throughout the design.
Related Questions
See All Topics

IMAGES
VIDEO
COMMENTS
26. I'm developing a little thing in VHDL and am quite new to it. I'm having trouble figuring out how to slice a bigger std_logic_vector into a smaller one. For instance I have 3 signals: signal allparts: std_logic_vector(15 downto 0); signal firstpart: std_logic_vector(7 downto 0); signal secondpart: std_logic_vector(7 downto 0);
For example: process (clk) begin. if rising_edge(clk) then. --multiply, truncate then assign. res <= (a * b)(15 downto 8); end if; end process; Where res, a, b are all 8 bit wide std_logic_vectors. This would be very useful in multiplcations and additions since the number of bits grow all the time and would save a several lines of code, making ...
\$\begingroup\$ Note that ghdl, which is possibly one of the strictest VHDL compilers according to standards compliance, has no problem with this code (assuming a is declared in the same architecture and thus its length is static) \$\endgroup\$
The std_logic_vector is a composite type, which means that it's a collection of subelements. Signals or variables of the std_logic_vector type can contain an arbitrary number of std_logic elements. This blog post is part of the Basic VHDL Tutorials series. The syntax for declaring std_logic_vector signals is: signal <name> : std_logic_vector ...
1 1. Bits, Vectors, Signals, Operators, Types 1.1 Bits and Vectors in Port Bits and vectors declared in port with direction. Example: port ( a : in std_logic; -- signal comes in to port a from outside b : out std_logic; -- signal is sent out to the port b c : inout std_logic; -- bidirectional port x : in std_logic_vector(7 downto 0); -- 8-bit input vector
An array contains multiple elements of the same type. When an array object is declared, an existing array type must be used. An array type definition can be unconstrained, i.e. of undefined length. String, bit_vector and std_logic_vector are defined in this way. An object (signal, variable or constant) of an unconstrained array type must have ...
The VHDL keyword "std_logic_vector" defines a vector of elements of type std_logic. For example, std_logic_vector (0 to 2) represents a three-element vector of std_logic data type, with the index range extending from 0 to 2. Let's use the "std_logic_vector" data type to describe the circuit in Figure 3. We will use three vectors a_vec ...
VHDL is a strongly typed language. All vectors which you concatanate on the right side should be of same data type. And the data type of the result of the right side expression should match with the data type of the left side expression. In VHDL, "bit" is a 2-valued data type and "std_logic" is an 8-valued data type. They both are different.
VHDL signal assignment with the OTHERS keyword. 03-06-2009 10:28 PM. I'm not sure I'm posting this in the right place but I want to assign an unsigned or std_logic_vector to the same type of a larger size. Input is 8 bits wide, outputsignal is 32 bits wide and I want to assign inputsignal (7 downto 0) to outputsignal (23 downto 16) with all ...
1. It's not possible to mix connected and open signals in a port map. You could introduce a *_float signal as an intermediate signal. The advantage is that you can filter for warnings related to these signal names and ignore all "unconnected signal" warnings. - Paebbels.
I have a memory block in my VHDL code and it has output std_logic_vectors that are 32 bit wide. ... If the original signal is std_logic_vector, the partial alias expressions have to be std_logic_vector or std_logic. In individual assignments, you are free to cast to a different type, e.g. signed or unsigned. SIGNAL controlreg : STD_LOGIC_VECTOR ...
I am using "use IEEE.std_logic_unsigned.all;" so that all my STD_LOGIC_VECTOR's are treated as unsigned numbers. Suppose I perform a math operatrion but I want to truncate using the same line in VHDL. Would this be possible? For example: process (clk) begin; if rising_edge (clk) then--multiply, truncate then assign; res <= (a * b)(15 downto 8 ...
See LRM sections 7.3.2. Rules and Examples. Aggregates are a grouping of values to form an array or record expression. The first form is called positional association, where the values are associated with elements from left to right: signal Z_BUS : bit_vector (3 downto 0); signal A_BIT, B_BIT, C_BIT, D_BIT : bit; ...
As others said, use ieee.numeric_std, never ieee.std_logic_unsigned, which is not really an IEEE package.. However, if you are using tools with VHDL 2008 support, you can use the new package ieee.numeric_std_unsigned, which essentially makes std_logic_vector behave like unsigned.. Also, since I didn't see it stated explicitly, here's actual code example to convert from an (unsigned) integer to ...
I'm receiving from outside std_logic_vector with binary value, that is represent the bit which should be set to one and others to 0. As I understand it's decoder, but solving this problem with "when" ... Concurrent signal assignment with barrel shifter in VHDL 2002 (exercise: understand that concurrent signal assignments are processes, imagine ...
The term aggregate mean "collection". That is true for the right hand side of an assignment, but not for the left hand side (LHS). The LHS is the reverse operation - a split operation. It is called decomposition or unpacking. The correct way in the VHDL LRM would be to have a e.g. decomposition BNF rule, that is an alias for the aggregate rule.
I would like to enter a number in a a variable of type STD_LOGIC_VECTOR but I have problems with the compiler. signal cl_output_ChA : STD_LOGIC_VECTOR (16-1 downto 0); cl_ouput_ChA <= 111111111111111; The compiler give me these two messages: The integer value of 111111111111111 is greater than integer'high.
begin. result_sig <= input_1 * input_2; end architecture multiplicator_test_arch; The result-signed-type has to be double width from the two input-signed-types. Declare another signed-signal with the double width from your multiplication. put the result from the multiplication in there.
VHDL also supports user defined scalar enumeration types which are supported by FPGA vendors most particularly for describing finite state machines which would also document state transitions in a readable form without resorting to constructing truth tables.