Java byte Array - byte Array in Java, initialize, String
Java byte array, byte array in java.
Java byte Array is used to store byte data type values only . The default value of the elements in a byte array is 0 . With the following Java byte array examples you can learn

How to declare byte array in Java ?
- How to initialize byte array in Java ?
How to initialize empty byte array in Java ?
How to assign value to byte array in java .
- How to get values from Java byte array ?
How to convert String to byte array in Java ?
- How to convert a byte Array to String in Java ?
- How to convert Java byte array to String ?
What is byte ?
A group of binary digits or bits ( usually eight ) operated on as a unit . A byte considered as a unit of memory size .
The byte is a unit of digital information that most commonly consists of eight bits . Historically, the byte was the number of bits used to encode a single character of text in a computer and for this reason it is the smallest addressable unit of memory in many computer architectures .
A byte is a data measurement unit that contains eight bits, or a series of eight zeros and ones . A single byte can be used to represent 2 8 or 256 different values .
What does byte mean in Java ?
The byte is one of the primitive data types in Java . This means that the Java byte is the same size as a byte in computer memory: it's 8 bits, and can hold values ranging from -128 to 127 . The byte data type comes packaged in the Java programming language and there is nothing special you have to do to get it to work .
What do we mean by byte array ?
A byte is 8 bits (binary data).
A byte array is an array of bytes.
You could use a byte array to store a collection of binary data ( byte[] ), for example, the contents of a file. The downside to this is that the entire file contents must be loaded into memory.
How many bits is a byte in Java ?
The byte is equal to 8 bits in Java .
Is byte a keyword in Java ?
The byte is a keyword in Java which designates the 8 bit signed integer primitive type .
The standard Java integer data types are in bytes :
- byte 1 byte -128 to 127
- short 2 bytes -32768 to 32767
- int 4 bytes -2147483648 to 2147483647
- long 8 bytes -9223372036854775808 to 9223372036854775807
What is byte array ?
The byte array is an array of bytes.
Arrays are declared with [] ( square brackets ) . If you put [] ( square brackets ) after any variable of any type only that variable is of type array remaining variables in that declaration are not array variables those are normal variables of that type .
If you put [] ( square brackets ) after any data type all the variables in that declaration are array variables . All the elements in the array are accessed with index . The array element index is starting from 0 to n-1 number i.e. if the array has 5 elements then starting index is 0 and ending index is 4 .
// How to declare byte array in Java Example byte[] byteArray; byte byteArray[]; byte[] byte_array; byte[] java_byte_array;
Related : Java boolean Array
Initializing byte Array
How to initialize ( init ) byte array ( byte[] ) in java .
The byte array will be initialized ( init ) to 0 when you allocate it . All arrays in Java are initialized to the default value for the type . This means that arrays of ints are initialized to 0, arrays of booleans are initialized to false and arrays of reference types are initialized to null .
how to init byte array example
// Java bytearray // how to init byte array or byte[] in java byte[] byteArray = new byte[10];
You can initialize empty byte array in Java following ways.
// How to initialize empty byte array in Java Example byte[] empty_byte_array = new byte[5];
How to Check byte Array is null in Java ?
Empty Check Array Null Using Java 8. If you are working with Java 8 or higher version then you can use the stream() method of Arrays class to call the allMatch() method to check whether array contains null values or not. This example is also useful for How to validate byte array in Java ?
How to Check byte Array Size in Java ?
In Java byte is occupied in memory is 1 byte. The size of byte array is equal to the length of byte array.
Java byte Array Size Example
Related : Java char Array
How to Check Empty byte Array in Java ?
To check byte array is empty or not, check all the elements in a byte array are zeros. If all the elements in a byte array are zeros then it is an empty byte array.
Java byte Array is Empty or not Example
What is the initial or default value of a byte array in java .
The initial value of a byte array is 0 . Because the default value of a byte data type in Java is 0 .
What is the length of a byte Array in Java ?
The length is a property of an array object in Java that returns the number of elements in a given array .
What is the Maximum length of a byte Array in Java ?
The maximum length of a byte array in Java is 2147483647 . You can store elements upto 2147483647 .
What is the Length of an Array in Java ?
In Java all the arrays are indexed and declared by int only . That is the size of an array must be specified by an int value and not long or short . All the arrays index beginning from 0 to ends at 2147483646 . You can store elements upto 2147483647 . If you try to store long ( big ) elements in array, you will get performance problems . If you overcome performance problems you should go to java collections framework or simply use Vector .
Following program shows Java byte Array Example . Save the following Java byte Array Example program with file name JavaByteArray.java .
Java byte Array Example
Related : Java short Array
Assigning Values to byte Array
Following Java byte array example you can learn how to assign values to java byte array at the time of declaration . Following example shows How to assign values to java byte array at the time of declaration . Save the following assign values to java byte array at the time of declaration Example program with file name AssignValuesToByteArray.java .
How to assign values to java byte array at the time of declaration
How to declare java byte array with other java byte array variables .
Following Java byte array example you can learn how to declare Java byte array with other Java byte array variables . Following example shows How to declare Java byte array with other Java byte array variables . Save the following declare Java byte array with other Java byte array variables Example program with file name DeclareMultipleByteArrays.java .
Declare Java byte array with other Java byte array variables Example
Related : Java int Array
How to assign Java byte array to other Java byte array ?
Following Java byte array example you can learn how to assign Java byte array to other Java byte array . Following example shows How to assign Java byte array to other Java byte array . Save the following assign Java byte array to other Java byte array Example program with file name AssignByteArrayToByteArray.java .
Assign Java byte array to other Java byte array Example
Related : Java float Array
Converting to byte Array
Java convert string to byte[].
We can convert String to byte[] in java two ways.
- By Using String.getBytes() method
- By Using Base64 class in Java 8
To convert from String to byte array , use String.getBytes() method . Please note that this method uses the platform’s default charset .
We can use String class getBytes() method to encode the string into a sequence of bytes using the platform’s default charset . This method is overloaded and we can also pass Charset as argument .
Following example shows How to convert String to byte array in Java . Save the following convert String to byte array in Java Example program with file name StringToByteArray.java .
Convert String to byte array in Java Example
Related : Java long Array
How to convert String to byte array in Java 8 ?
To convert from String to byte array in Java 8, use Base64.getDecoder().decode() method . Base64.getDecoder().decode() method converts a string to byte array . The Base64 class is since java 1.8 so this code won't work before java 1.8 .
Following example shows How to convert String to byte array in Java 8 . Save the following convert String to byte array in Java 8 Example program with file name StringToByteArrayJava8.java . Please note in the ByteArrayToFile.java program the import statement import java.util.* is used, in detail the import statements java.util.Arrays, java.util.Base64 used.
Convert String to byte array in Java 8 Example
Related Topic Java Byte Array to SQL Server : How to store byte array in SQL Server using Java ?
How to convert byte[] array to String in Java ?
In Java, we can use new String(bytes, StandardCharsets.UTF_8) to convert a byte[] to a String. For text or character data, we use new String(bytes, StandardCharsets.UTF_8) to convert a byte[] to a String.
We can use String class getBytes() method to encode the string into a sequence of bytes using the platform's default charset.
We can convert Java byte array to String in two ways .
- Without character encoding.
- With character encoding.
How to convert a byte Array to String in Java without character encoding ?
We can convert the byte array to String without even specifying the character encoding . Just pass the byte array to the String constructor .
Following example shows How to convert a byte Array to String in Java without character encoding . Save the following convert a byte Array to String in Java without character encoding Example program with file name ByteArrayToString.java .
Convert a byte Array to String in Java without character encoding Example
How to convert a byte array to string in java with character encoding .
We can convert the byte array to String with UTF-8 character encoding .
Following example shows How to convert a byte Array to String in Java with character encoding . Save the following convert a byte Array to String in Java with character encoding Example program with file name ByteArrayToString2.java .
Convert a byte Array to String in Java with character encoding Example
Related : Java double Array
How to convert byte array to String in Java 8 ?
To convert from byte array to String in Java 8, use Base64.getEncoder().encodeToString() method . Base64.getEncoder().encodeToString() method converts byte array to String . The Base64 class is since java 1.8 so this code won't work before java 1.8 .
Following example shows How to convert byte array to String in Java 8 . Save the following convert byte array to String in Java 8 Example program with file name ByteArrayToStringJava8.java . Please note in the ByteArrayToFile.java program the import statement import java.util.* is used, in detail the import statements java.util.Arrays, java.util.Base64 used.
Convert byte array to String in Java 8 Example
Related Topic Java Byte Array to MySQL : How to store byte array in MySQL using Java ?
How to convert byte array to base64 String in Java 6 ?
To convert from byte array to base64 String in Java 6, use javax.xml.bind.DatatypeConverter class.
Following example shows How to convert byte array to base64 String in Java 6 . Save the following convert byte array to base64 String in Java 6 Example program with file name ByteArrayToBase64String.java .
Convert byte array to base64 String in Java 6 Example
What is bytebuffer in java .
A byte buffer is either direct or non-direct . Given a direct byte buffer , the Java virtual machine will make a best effort to perform native I/O operations directly upon it . That is , it will attempt to avoid copying the buffer's content to ( or from ) an intermediate buffer before ( or after ) each invocation of one of the underlying operating system's native I/O operations .
The allocate() method of java.nio.ByteBuffer class is used to allocate a new byte buffer .
The new buffer's position will be zero , its limit will be its capacity , its mark will be undefined , and each of its elements will be initialized to zero . It will have a backing array , and its array offset will be zero .
How to convert Java integer to byte Array ?
We will discuss various ways of converting int to a byte array . In java int data type take 4 bytes ( 32 bits ) and it’s range is -2147483648 to 2147483647 .
You can convert Java integer to byte Array using Java NIO's ByteBuffer is very simple . Following example shows how to convert Java integer to byte Array .
Following example shows How to convert Java integer to byte Array . Save the following convert Java integer to byte Array Example program with file name IntToByteArray.java .
Convert Java integer to byte Array Example
How to convert java byte array to long .
Java provide ByteBuffer class to do the same . To convert any byte array , first we need to allocate 8 bytes using ByteBuffer's static method allocate , then put byteArray using put method and flip bytebuffer by calling getLong() method we can get long value of that byte array .
You can convert Java byte Array to long using Java NIO's ByteBuffer is very simple .
Following example shows How to convert Java byte Array to long . Save the following convert Java byte Array to long program with file name ByteArrayToLong.java .
Convert Java byte Array to long Example
How to convert an object to byte array in java .
- Make the required object serializable by implementing the Serializable interface .
- Create a ByteArrayOutputStream object .
- Create an ObjectOutputStream object by passing the ByteArrayOutputStream object created in the previous step .
- Write the contents of the object to the output stream using the writeObject() method of the ObjectOutputStream class .
- Flush the contents to the stream using the flush() method .
- Finally , convert the contents of the ByteArrayOutputStream to a byte array using the toByteArray() method .
Following example shows How to convert an object to byte array in Java . Save the following convert an object to byte array program with file name ObjectToByteArray.java . Please note in the ObjectToByteArray.java program the import statement import java.io.* is used in detail, the import statements import java.io.ByteArrayOutputStream, import java.io.ObjectOutputStream, import java.io.Serializable, import java.io.IOException used.
Convert an object to byte array in Java Example
How to convert byte[] ( array ) to file using java .
- To convert byte[] to file getBytes() method of String class is used, and simple write() method can be used to convert that byte into a file.
- "hello".getBytes(); encodes given String into a sequence of bytes using the platform's default charset , Which stores the result into a new byte array .
- new File("c:\\demo.txt") Creates a new File instance by converting the given pathname string into an abstract pathname .
- new FileOutputStream(file) Creates a file output stream to write to the file represented by the specified File object .
- os.write(bytes) Writes number of bytes from the specified byte array to output stream .
Following example shows How to convert byte array to File Example . Save the following convert Java byte array to File program with file name ByteArrayToFile.java . Please note in the ByteArrayToFile.java program the import statement import java.io.* is used in detail, the import statements import java.io.BufferedReader, import java.io.File, import java.io.FileOutputStream, import java.io.FileReader, import java.io.OutputStream used.
Convert byte[] ( array ) to File using Java Example
More related tutorials.
Java Byte Explained [Easy Examples]
Bashir Alam
September 24, 2021
Introduction to Java byte
Every variable in java must have a data type. A variable's data type determines the values that the variable can contain and the operations that can be performed on it. There are eight different data types available in the java programming language. You can read more about the data types from the article on java data types . In this article, we will specifically focus on java byte data type.
We will see how to declare variables with java byte type and will solve various examples. We will also cover how to declare and initialize a java byte array and will perform different operations including typecasting and initializing an array using for loop. All in all, this tutorial, will contain all the necessary information and examples about java bytes.

Getting started with Java byte
A data type is an attribute of a variable that tells the compiler or interpreter how the programmer intends to use the variables. It defines the operations that can be done on the data and what type of values can be stored. The eight different data types in java can be categories into two main groups; Primitive data type and non-primitive data type .
A primitive data type is pre-defined by the programming language. The size and type of variable values are specified, and it has no additional methods. While the non-primitive data type is defined by the programming language but is created by the programmer. They are also called “ reference variables ” or “ object references ” since they reference a memory location that stores the data. Now the byte is simply a small section/ area which can store information. In the upcoming sections, we will focus on java byte and will cover the kind of data that they can store and will solve various examples related to them.
Java byte data type
A group of binary digits or bits operated on as a unit is called byte. A java byte is considered as a unit of memory size. A byte is a unit of digital information that most commonly consists of eight bits. Historically, the byte was the number of bits used to encode a single character of text in a computer and for this reason, it is the smallest addressable unit of memory in many computer architectures. A byte is a data measurement unit that contains eight bits, or a series of eight zeros and ones. A single byte can be used to represent 2 8 or 256 different values.
As we already discussed that the java byte is one of the primitive data types. This means that the Java byte is the same size as a byte in computer memory: it's 8 bits and can hold values ranging from -128 to 127 .
Declaring and initializing Java byte
Now we already have some theoretical knowledge about java byte. Let us now go into more details and start the implementation part. Here we will see how we can declare a java byte and initialize it to some values. See the syntax of java byte declaration below:
We use the keyword byte to declare java byte typed variable and then the name of variable ending with a semi-colon. Once we declared a byte variable, then we can initialize it to any value which must be in between -128 to 127. See the initialization of the byte variable below:
Another simple way is to declare and initialize the byte variable at the same time. For example, see the syntax below:
Here on the left side, we declared the variable type byte and then at the same time initialize it to any valid value on the right side.
Example-1 Using Java byte type
Now we know how to declare and initialize byte data type in java. Let us take a practical example and see how we can use byte data type in our java programming language. See the java program below:
Notice that we added the two-byte variables using plus operators.
Example-2 Java byte type error
We already discussed that the byte can take values from -128 to 127 but let us see what will happens if we try to store value beyond the range. See the example below where we stored the value to 140 to a one-byte type variable.
When we run the program we will get the following error.
![Java Byte Explained [Easy Examples] java byte](https://www.golinuxcloud.com/wp-content/uploads/java-byte-e1632484604423.png)
The error says that the byte value cannot contain a value of 140 and it cannot convert the byte to an integer ( which can contain values more than 127).
Java byte array type
An array in Java is a set of variables referenced by using a single variable name combined with an index number. Each item of an array is an element. All the elements in an array must be of the same type. You can read more about java arrays from the article on java arrays. As we already discussed that all elements of the array must be of the same type. We declare the type before initializing the array. In the following sections, we will see how we can declare and initialize a java array of type bytes.
Declaration and initialization of array byte in Java
We already had discussed that an array can store any type of element depending on the type we defined while declaring the array. Now we let us see how we can declare a java array of type byte. See the simple syntax below:
When we declare array variables in java, actually we only declare the variables (reference) to the arrays. The declaration does not actually create an array. We have to create an array. See the syntax below.
Inside the square brackets, we have to provide the size of the array. It can be any integer value. We can also declare and defined byte array type in one line as well instead of declaring and initializing separately. See the syntax below:
Notice that we declared and initialized the byte array in one line.
Example of Java byte array
Now we already know to declare and initialize byte array in java. Let us take an example and see how we can practically implement a byte typed array in java. See the example below:
Notice that we were able to successfully printed all the byte array in java.
Converting String to Java byte array
A String is stored as an array of Unicode characters in Java. You can learn more about string from the article on java strings . To convert it to a byte array, we translate the sequence of characters into a sequence of bytes. For this translation, we use an instance of charset. This class specifies a mapping between a sequence of chars and a sequence of bytes. Now let us take an example and see how we can convert a string into a java byte array. See the example below:
Notice that we get all the integer values as an output. It is because the getbytes() method converts the string values into their corresponding ASCII value of alphabets are integer values. For example, the integer value in ASCII for 'W' is 87 as we get it.
We already discussed that there are eight primitive data types that are supported by the Java programming language and byte is one of them. The byte data type is an 8-bit signed two's complement integer. It has a minimum value of -128 and a maximum value of 127 (inclusive). In this tutorial, we learned about the java byte in more detail. We covered how we can declare and initialize a byte type variable in java through various examples. At the same time, we had discussed what would happen if we provide a value larger than the range of byte.
Moreover, we also learned how we can create a byte typed array in java. Towards the end, we also covered how we can convert a string into a java byte array using an example. In a nutshell, this tutorial contains all the necessary information that you need to know in order to get started using java bytes.
Further Reading
Java bytes Java data types Java strings
He is a Computer Science graduate from the University of Central Asia, currently employed as a full-time Machine Learning Engineer at uExel. His expertise lies in OCR, text extraction, data preprocessing, and predictive models. You can reach out to him on his Linkedin or check his projects on GitHub page.
Can't find what you're searching for? Let us assist you.
Enter your query below, and we'll provide instant results tailored to your needs.
If my articles on GoLinuxCloud has helped you, kindly consider buying me a coffee as a token of appreciation.
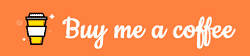
For any other feedbacks or questions you can send mail to [email protected]
Thank You for your support!!
Leave a Comment Cancel reply
Save my name and email in this browser for the next time I comment.
Notify me via e-mail if anyone answers my comment.
We try to offer easy-to-follow guides and tips on various topics such as Linux, Cloud Computing, Programming Languages, Ethical Hacking and much more.
Programming Languages
Exam certifications.
Certified Kubernetes Application Developer (CKAD)
CompTIA PenTest+ (PT0-002)
Red Hat EX407 (Ansible)
Hacker Rank Python
Privacy Policy
How to Initialize a Byte Array in Java?
In this tutorial, we will learn how to initialize a byte array in Java.
What is a byte?
We all know that 8 bits = 1 byte or we can say a combination of eight zeros and ones. A byte represents a sort of digital information or data in binary format.
Byte in Java
A byte in Java is one of the primitive data types. It means, a byte stores the same size as that of computer memory. If you look at some primitive data types in Java, you will see a byte stores values ranging from -128 to +127. That means we can store the values only from -128 to 127 and if we want some value greater than this range, then we can simply use datatype conversion .
Byte Array in Java
As we have seen, a byte is a combination of eight zeros and ones. A byte array is a combination of bytes values. It means if you want to load some content directly into the memory then this can be helpful.
How to Initialize a byte array in Java?
Now, there are many ways in which we can initialize a byte array. Examples are given below:
- byte[] array_name;
- byte array_name[];
When you assign memory to a byte array, initially the default value is zero. This is not only in byte array but also in all the arrays in Java. There is always some initial value allocated to every array.
Assigning Elements to byte array in Java
In Java, we assign elements to the Java array by indexing only. An example is given below:
In this example, you can see, we have declared an array for byte with the size of 5. Then we have assigned values to it with the help of indexing. And printing all values with the help of for-loop iteration. The output:
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Please enable JavaScript to submit this form.
Related Posts
- How to convert BLOB to Byte Array in java
- How to concatenate byte array in java with an easy example
- How to convert Byte Array to BLOB in java
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
- Creating a Dynamic Array in Java
- Array Copy in Java
- How to Create Array of Objects in Java?
- Simplest and Best method to print a 2D Array in Java
- Array Declarations in Java (Single and Multidimensional)
- How to Take Array Input From User in Java
- Sorting a 2D Array according to values in any given column in Java
- How to Initialize an Array in Java?
- How to Return an Array in Java?
- Print a 2 D Array or Matrix in Java
- Iterating over Arrays in Java
- Find max or min value in an array of primitives using Java
- Difference between Arrays and Collection in Java
- Arrays in Java
- Java Array mismatch() Method with Examples
- Array Literals in Java
- Is an array a primitive type or an object in Java?
- Anonymous Array in Java
- Java Array Programs
Array Variable Assignment in Java
An array is a collection of similar types of data in a contiguous location in memory. After Declaring an array we create and assign it a value or variable. During the assignment variable of the array things, we have to remember and have to check the below condition.
1. Element Level Promotion
Element-level promotions are not applicable at the array level. Like a character can be promoted to integer but a character array type cannot be promoted to int type array.
2. For Object Type Array
In the case of object-type arrays, child-type array variables can be assigned to parent-type array variables. That means after creating a parent-type array object we can assign a child array in this parent array.
When we assign one array to another array internally, the internal element or value won’t be copied, only the reference variable will be assigned hence sizes are not important but the type must be matched.
3. Dimension Matching
When we assign one array to another array in java, the dimension must be matched which means if one array is in a single dimension then another array must be in a single dimension. Samely if one array is in two dimensions another array must be in two dimensions. So, when we perform array assignment size is not important but dimension and type must be matched.
Please Login to comment...
Similar reads.
- What are Tiktok AI Avatars?
- Poe Introduces A Price-per-message Revenue Model For AI Bot Creators
- Truecaller For Web Now Available For Android Users In India
- Google Introduces New AI-powered Vids App
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Learn Java practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn java interactively, java introduction.
- Java Hello World
- Java JVM, JRE and JDK
- Java Variables and Literals
- Java Data Types
- Java Operators
- Java Input and Output
- Java Expressions & Blocks
- Java Comment
Java Flow Control
- Java if...else
- Java switch Statement
- Java for Loop
- Java for-each Loop
- Java while Loop
- Java break Statement
- Java continue Statement
- Java Arrays
- Multidimensional Array
- Java Copy Array
Java OOP (I)
- Java Class and Objects
- Java Methods
- Java Method Overloading
- Java Constructor
- Java Strings
- Java Access Modifiers
- Java this keyword
- Java final keyword
- Java Recursion
- Java instanceof Operator
Java OOP (II)
- Java Inheritance
- Java Method Overriding
- Java super Keyword
- Abstract Class & Method
- Java Interfaces
- Java Polymorphism
- Java Encapsulation
Java OOP (III)
- Nested & Inner Class
- Java Static Class
- Java Anonymous Class
- Java Singleton
- Java enum Class
- Java enum Constructor
- Java enum String
- Java Reflection
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java List Interface
- Java ArrayList
- Java Vector
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue Interface
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet
- Java EnumSet
- Java LinkedhashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
- Java Algorithms
- Java Iterator
- Java ListIterator
- Java I/O Streams
- Java InputStream
- Java OutputStream
- Java FileInputStream
- Java FileOutputStream
- Java ByteArrayInputStream
- Java ByteArrayOutputStream
- Java ObjectInputStream
- Java ObjectOutputStream
- Java BufferedInputStream
- Java BufferedOutputStream
- Java PrintStream
Java Reader/Writer
- Java Reader
- Java Writer
- Java InputStreamReader
- Java OutputStreamWriter
- Java FileReader
- Java FileWriter
- Java BufferedReader
- Java BufferedWriter
- Java StringReader
- Java StringWriter
- Java PrintWriter
Additional Topics
- Java Scanner Class
- Java Type Casting
- Java autoboxing and unboxing
- Java Lambda Expression
- Java Generics
- Java File Class
- Java Wrapper Class
- Java Command Line Arguments
Java Tutorials
Java InputStream Class
Java FileInputStream Class
Java BufferedInputStream Class
Java InputStreamReader Class
- Java ByteArrayOutputStream Class
- Java Reader Class
Java ByteArrayInputStream Class
The ByteArrayInputStream class of the java.io package can be used to read an array of input data (in bytes).
It extends the InputStream abstract class.
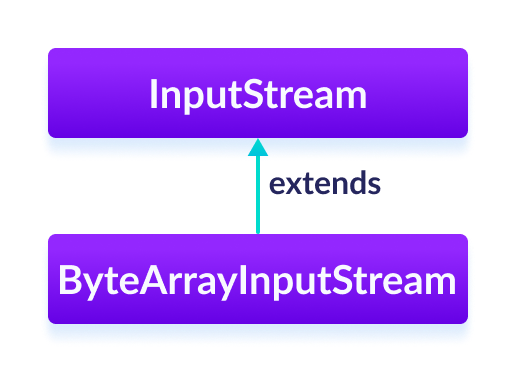
Note : In ByteArrayInputStream , the input stream is created using the array of bytes. It includes an internal array to store data of that particular byte array.
- Create a ByteArrayInputStream
In order to create a byte array input stream, we must import the java.io.ByteArrayInputStream package first. Once we import the package, here is how we can create an input stream.
Here, we have created an input stream that reads entire data from the arr array. However, we can also create the input stream that reads only some data from the array.
Here the input stream reads the number of bytes equal to length from the array starting from the start position.
Methods of ByteArrayInputStream
The ByteArrayInputStream class provides implementations for different methods present in the InputStream class.
- read() Method
- read() - reads the single byte from the array present in the input stream
- read(byte[] array) - reads bytes from the input stream and stores in the specified array
- read(byte[] array, int start, int length) - reads the number of bytes equal to length from the stream and stores in the specified array starting from the position start
Example: ByteArrayInputStream to read data
In the above example, we have created a byte array input stream named input .
Here, the input stream includes all the data from the specified array. To read data from the input stream, we have used the read() method.
- available() Method
To get the number of available bytes in the input stream, we can use the available() method. For example,
In the above example,
- We have used the available() method to check the number of available bytes in the input stream.
- We have then used the read() method 2 times to read 2 bytes from the input stream.
- Now, after reading the 2 bytes, we have checked the available bytes. This time the available bytes decreased by 2.
- skip() Method
To discard and skip the specified number of bytes, we can use the skip() method. For example,
In the above example, we have used the skip() method to skip 2 bytes of data from the input stream. Hence 1 and 2 are not read from the input stream.
close() Method
To close the input stream, we can use the close() method.
However, the close() method has no effect in ByteArrayInputStream class. We can use the methods of this class even after the close() method is called.
- Other Methods Of ByteArrayInputStream
To learn more, visit Java ByteArrayInputStream (official Java documentation) .
Table of Contents
- Introduction
Sorry about that.
Related Tutorials
Java Tutorial
Java Array assignment
In this tutorial on Java Arrays, we will see to how to assign an array – both Primitive Array assignment and Reference Array assignment.
Java Primitive Array Assignment
If an array is declared as int (int[]) then you can assign another int array of any size but cannot assign anything that is not an int array including primitive int variable. Following is an example of int[] array assignment.
Java Reference Array Assignment
If an array is declared as a reference type then you can assign any other array reference which is a subtype of the declared reference type, i.e.) it should pass the “IS-A relationship”. Following is an example of reference array assignment.
Similarly, if an array is declared as interface type then it can reference an array of any type that implements that interface.
Leave a Comment Cancel reply
This site uses Akismet to reduce spam. Learn how your comment data is processed .
- Visitor Master
- Garage Master
- Builder Master
- Call Service Management
- Mobile Solutions
- Cloud Deployment Services
- Web Solutions
- Business Technology Consulting
- Graphic Designs
- Search Engine Optimization
- Social Media Marketing
- Software Training
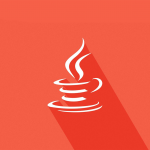

IMAGES
VIDEO
COMMENTS
27. If you're trying to assign hard-coded values, you can use: byte[] bytes = { (byte) 204, 29, (byte) 207, (byte) 217 }; Note the cast because Java bytes are signed - the cast here will basically force the overflow to a negative value, which is probably what you want. If you're actually trying to parse a string, you need to do that - split the ...
To initialize a byte array in Java, you can use the array initializer syntax, like this: byte [] bytes = { 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09 }; This will create an array of bytes with the specified values. Alternatively, you can use the new operator to create an array of a specified size and then assign values to its ...
Following Java byte array example you can learn how to assign Java byte array to other Java byte array . Following example shows How to assign Java byte array to other Java byte array . Save the following assign Java byte array to other Java byte array Example program with file name AssignByteArrayToByteArray.java .
The Charset class provides encode(), a convenient method that encodes Unicode characters into bytes. This method always replaces invalid input and unmappable-characters using the charset's default replacement byte array. Let's use the encode method to convert a String into a byte array: @Test public void whenEncodeWithCharset_thenOK() { String inputString = "Hello ਸੰਸਾਰ!";
We can rewrite the code above in a more straightforward way using autoboxing to convert a byte value into a Byte instance and vice versa, utilizing unboxing.. If we disassemble our next code fragments - we can use the javap tool for this purpose - we'll see that autoboxing calls the Byte.valueOf() method, while unboxing calls byteValue() of a Byte object under the hood.
We can combine two or more byte arrays using the above method: byte [] combined = Bytes.concat(first, second, third); assertArrayEquals(expectedArray, combined); As we can see, the resultant combined array contains elements from all the arrays passed to the concat () method. 3.2.
variableName = value; Another simple way is to declare and initialize the byte variable at the same time. For example, see the syntax below: bash. byte variableName = value; Here on the left side, we declared the variable type byte and then at the same time initialize it to any valid value on the right side. ALSO READ.
When you assign memory to a byte array, initially the default value is zero. This is not only in byte array but also in all the arrays in Java. There is always some initial value allocated to every array.
The java.lang.reflect.Array.setByte () is an inbuilt method in Java and is used to set a specified byte value to a specified index of a given object array. Syntax: Array.setByte(Object []array, int index, byte value) Parameter: This method takes 3 parameters: array: This is an array of type Object which is to be updated.
What you need to do is to return a new byte[] from the method like this: public byte[] methodThatAllocatesByteArray() {. // create and populate array. return array; } and call it like this: byte[] temp = methodThatAllocatesByteArray(). Or you can initialise the array first, then pass the reference to that array to the other method like this:
In the above example, we have created a byte array output stream named output. ByteArrayOutputStream output = new ByteArrayOutputStream(); To write the data to the output stream, we have used the write() method. Note: The getBytes () method used in the program converts a string into an array of bytes.
After Declaring an array we create and assign it a value or variable. During the assignment variable of the array things, we have to remember and have to check the below condition. 1. Element Level Promotion. Element-level promotions are not applicable at the array level. Like a character can be promoted to integer but a character array type ...
As with the Java NIO package, we can write our byte [] in one line: Files.write(dataForWriting, outputFile); Guava's Files.write method also takes an optional OptionOptions and uses the same defaults as java.nio.Files.write. There's a catch here though; the Guava Files.write method is marked with the @Beta annotation.
The ByteArrayInputStream class of the java.io package can be used to read an array of input data (in bytes).. It extends the InputStream abstract class.. Java ByteArrayInputStream. Note: In ByteArrayInputStream, the input stream is created using the array of bytes.It includes an internal array to store data of that particular byte array.
In Java, an array where each element is itself an array is effectively just a 2D array, although Java doesn't require that each row have the same number of elements. In this particular case where you have a List<byte[]> and you wish to turn that into an array where each element of the array is itself a byte array you could use:
String class also has a method to convert a subset of the byte array to String. byte[] byteArray1 = { 80, 65, 78, 75, 65, 74 }; String str = new String(byteArray1, 0, 3, StandardCharsets.UTF_8); Above code is perfectly fine and 'str' value will be 'PAN'. That's all about converting byte array to String in Java.
int value = 0; for (byte b : bytes) { value = (value << 8) + (b & 0xFF); } Normally, the length of the bytes array in the above code snippet should be equal to or less than four. That's because an int value occupies four bytes. Otherwise, it will lead to the int range overflow.. To verify the correctness of the conversion, let's define two constants:
Java Reference Array Assignment. If an array is declared as a reference type then you can assign any other array reference which is a subtype of the declared reference type, i.e.) it should pass the "IS-A relationship". Following is an example of reference array assignment. animals = cats; //OK. Cat IS-A Animal.
byte [] byteArray = FileUtils.readFileToByteArray( new File (FILE_NAME)); assertArrayEquals(expectedByteArray, byteArray); As we see above, readFileToByteArray () reads the content of the specified file into a byte array in a straightforward way. The good thing about this method is that the file is always closed.
It is not possible to assign a byte array to an object in java and have all the member variables populated automatically, but it is possible to get a java object out of a byte array using serialization. You can use ObjectInputStream and ObjectOutputStream to get objects in and out of a stream.
The following constructor will read the byte array and decode it and according to the default charset.. new String(arr); So when you do. String s= new String(arr); s.getBytes() the bytes() returns the array again as was previously decoded according to the default charset.. If you inspect with debugger you can see how the new String(byte []) method works for UTF-8 default charSet.