Chapter 3 - Data Flow Descriptions
Section 3 - the delay model.

VHDL Delay Type Modeling
In VHDL the designer has the possibility to perform a signal assignment after certain amount of time, implementing the delay in the assignment.
VHDL Delay Type
In VHDL there are two different kind of delay
- Transport delay
- Inertial delay
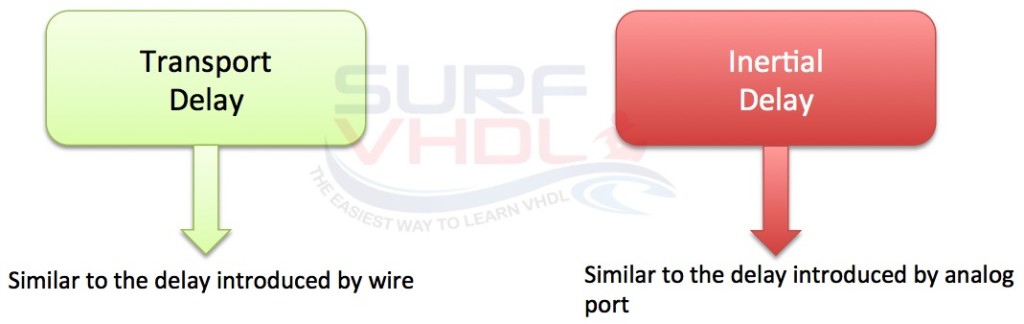
The transport delay is used to model the delay introduced by wire connection or a PCB connection. The inertial delay models the delay introduced by an analog port , which means, it is analogous to the delay in devices that respond only if the signal value persists on their inputs for a given amount of time.
It is useful in order to ignore input glitches whose duration is less than the port delay.
Inertial Delay Model
The inertial dela y model is the default delay implemented in VHDL because it’s behavior is very similar to the delay of the device. The delay assignment syntax is:
b <= a after 20 ns;
In this example b take the value of a after 20 ns second of inertial delay.
This means that if a value varies faster than 20 ns b remain unchanged . This simulation should clarify the concept.
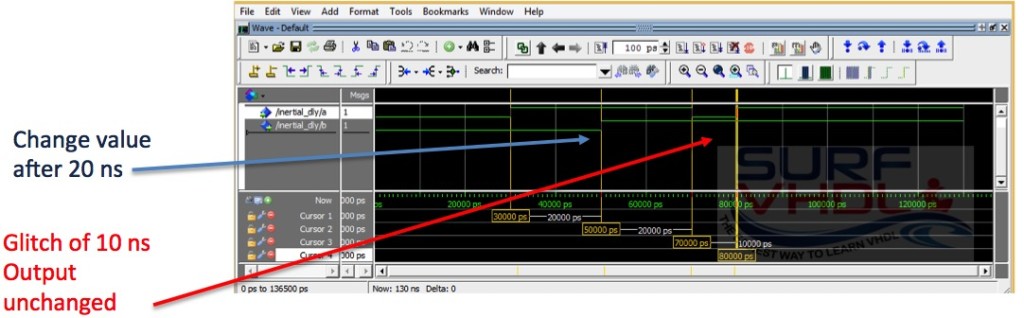
At simulation start a and b are 0 ; a change from 0 to 1 , and b change its value after 20 ns ; then a changes value going to 0 and then to 1 in 10 ns .
This delay is less than inertial delay of 20 ns. So b remains unchanged .
Transport Delay Model
The transport delay is not the default delay implemented in VHDL and must be specified . This delay model is useful to describe delay line, PCB delay, wire delay. The delay assignment syntax is:
b <= transport a after 20 ns;
in this example b take the value of a after 20 ns second of transport delay .
This means that no matter how fast a changes his value, b will follow the behavior of a after the amount of time specified in the delay statement . This simulation should clarify the concept.
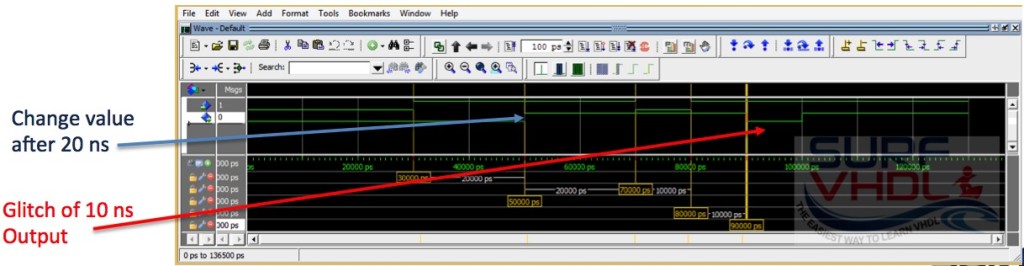
The simulation is the same as the inertial delay. In this case the value of b follow the value of a after 20 ns even when a has a glitch of 10 ns
VHDL transport and inertial delay model allow the designer to model different type of behavior on VHDL hardware implementation. They are very useful in test bench modeling and in VHDL macro model delay modeling such as RAM, ROM, and peripheral interfacing.
Previous – Next
A signal assignment statement modifies the target signal
Description:
A Signal assignment statement can appear inside a process (sequential statement) or directly in an architecture (concurrent statement). The target signal can be either a name (simple, selected, indexed, or slice) or an aggregate .
A signal assignment with no delay (or zero delay) will cause an event after delta delay, which means that the event happens only when all of the currently active processes have finished executing (i.e. after one simulation cycle).
The default delay mode ( inertial ) means that pulses shorter than the delay (or the reject period if specified) are ignored. Transport means that the assignment acts as a pure delay line.
VHDL'93 defines the keyword unaffected which indicates a choice where the signal is not given a new assignment. This is roughly equivalent to the use of the null statement within case (see Examples).
- Delays are usually ignored by synthesis tool.
Aggregate , Concurrent statement , Sequential statement , Signal , Variable assignment
In VHDL -93, any signal assignment statement may have an optional label:
vhdl_reference_93:signal_assignment
Signal assignment ... <= ..."
Signal_assignment_statement.
- function_statement_part
- procedure_statement_part
- process_statement_part
Further definitions
Delay_mechanism.
[ reject time _ expression ] inertial
waveform_element { , waveform_element }
The keyword unaffected is allowed only in concurrent signal assignments.
The default delay mechanism is inertial .
With transport all pulses are transmitted.
With inertial only those pulses are transmitted, with width is greater than the a given limit. This limit is given by the value after reject or the first time value in the waveform.
The value after reject must not be greater than the first time value of the waveform.
The value of B is assigned to signal A after 5 ns.
In the first example the inertial delay model is used, in the second example the transport delay model is used.
A waveform is assigned to signal data , according to which data receives the value 2 after 1 ns, the value 4 after 3ns and the value 10 after 8ns.
Signal A is assigned the result of the function my_function by the inertial delay model
In the first example this happens after 5ns; in the second and third example this happens after a time which is determined by the constant delay. In the third example the value 0 is transferred to A 2ns after the preceding assignment.
These assignments are equivalent. The value of input is driven to output with a delay of 6 ns. Pulses smaller 6 ns are suppressed.
Here the pulse rejection limit is smaller than the first time expression in the waveform. All pulses smaller 3 ns are suppressed.
The signal assignments to output1 and ouput2 are equivalent to those of ouput3 and output4 .
No pulses are suppressed.
In VHDL'87 one had to choose the above assignments. Both variants are equivalent.
VHDL Logical Operators and Signal Assignments for Combinational Logic
In this post, we discuss the VHDL logical operators, when-else statements , with-select statements and instantiation . These basic techniques allow us to model simple digital circuits.
In a previous post in this series, we looked at the way we use the VHDL entity, architecture and library keywords. These are important concepts which provide structure to our code and allow us to define the inputs and outputs of a component.
However, we can't do anything more than define inputs and outputs using this technique. In order to model digital circuits in VHDL, we need to take a closer look at the syntax of the language.
There are two main classes of digital circuit we can model in VHDL – combinational and sequential .
Combinational logic is the simplest of the two, consisting primarily of basic logic gates , such as ANDs, ORs and NOTs. When the circuit input changes, the output changes almost immediately (there is a small delay as signals propagate through the circuit).
Sequential circuits use a clock and require storage elements such as flip flops . As a result, changes in the output are synchronised to the circuit clock and are not immediate. We talk more specifically about modelling combinational logic in this post, whilst sequential logic is discussed in the next post.

Combinational Logic
The simplest elements to model in VHDL are the basic logic gates – AND, OR, NOR, NAND, NOT and XOR.
Each of these type of gates has a corresponding operator which implements their functionality. Collectively, these are known as logical operators in VHDL.
To demonstrate this concept, let us consider a simple two input AND gate such as that shown below.
The VHDL code shown below uses one of the logical operators to implement this basic circuit.
Although this code is simple, there are a couple of important concepts to consider. The first of these is the VHDL assignment operator (<=) which must be used for all signals. This is roughly equivalent to the = operator in most other programming languages.
In addition to signals, we can also define variables which we use inside of processes. In this case, we would have to use a different assignment operator (:=).
It is not important to understand variables in any detail to model combinational logic but we talk about them in the post on the VHDL process block .
The type of signal used is another important consideration. We talked about the most basic and common VHDL data types in a previous post.
As they represent some quantity or number, types such as real, time or integer are known as scalar types. We can't use the VHDL logical operators with these types and we most commonly use them with std_logic or std_logic_vectors.
Despite these considerations, this code example demonstrates how simple it is to model basic logic gates.
We can change the functionality of this circuit by replacing the AND operator with one of the other VHDL logical operators.
As an example, the VHDL code below models a three input XOR gate.
The NOT operator is slightly different to the other VHDL logical operators as it only has one input. The code snippet below shows the basic syntax for a NOT gate.
- Mixing VHDL Logical Operators
Combinational logic circuits almost always feature more than one type of gate. As a result of this, VHDL allows us to mix logical operators in order to create models of more complex circuits.
To demonstrate this concept, let’s consider a circuit featuring an AND gate and an OR gate. The circuit diagram below shows this circuit.
The code below shows the implementation of this circuit using VHDL.
This code should be easy to understand as it makes use of the logical operators we have already talked about. However, it is important to use brackets when modelling circuits with multiple logic gates, as shown in the above example. Not only does this ensure that the design works as intended, it also makes the intention of the code easier to understand.
- Reduction Functions
We can also use the logical operators on vector types in order to reduce them to a single bit. This is a useful feature as we can determine when all the bits in a vector are either 1 or 0.
We commonly do this for counters where we may want to know when the count reaches its maximum or minimum value.
The logical reduction functions were only introduced in VHDL-2008. Therefore, we can not use the logical operators to reduce vector types to a single bit when working with earlier standards.
The code snippet below shows the most common use cases for the VHDL reduction functions.
Mulitplexors in VHDL
In addition to logic gates, we often use multiplexors (mux for short) in combinational digital circuits. In VHDL, there are two different concurrent statements which we can use to model a mux.
The VHDL with select statement, also commonly referred to as selected signal assignment, is one of these constructs.
The other method we can use to concurrently model a mux is the VHDL when else statement.
In addition to this, we can also use a case statement to model a mux in VHDL . However, we talk about this in more detail in a later post as this method also requires us to have an understanding of the VHDL process block .
Let's look at the VHDL concurrent statements we can use to model a mux in more detail.
VHDL With Select Statement
When we use the with select statement in a VHDL design, we can assign different values to a signal based on the value of some other signal in our design.
The with select statement is probably the most intuitive way of modelling a mux in VHDL.
The code snippet below shows the basic syntax for the with select statement in VHDL.
When we use the VHDL with select statement, the <mux_out> field is assigned data based on the value of the <address> field.
When the <address> field is equal to <address1> then the <mux_out> signal is assigned to <a>, for example.
We use the the others clause at the end of the statement to capture instance when the address is a value other than those explicitly listed.
We can exclude the others clause if we explicitly list all of the possible input combinations.
- With Select Mux Example
Let’s consider a simple four to one multiplexer to give a practical example of the with select statement. The output Q is set to one of the four inputs (A,B, C or D) depending on the value of the addr input signal.
The circuit diagram below shows this circuit.
This circuit is simple to implement using the VHDL with select statement, as shown in the code snippet below.
VHDL When Else Statements
We use the when statement in VHDL to assign different values to a signal based on boolean expressions .
In this case, we actually write a different expression for each of the values which could be assigned to a signal. When one of these conditions evaluates as true, the signal is assigned the value associated with this condition.
The code snippet below shows the basic syntax for the VHDL when else statement.
When we use the when else statement in VHDL, the boolean expression is written after the when keyword. If this condition evaluates as true, then the <mux_out> field is assigned to the value stated before the relevant when keyword.
For example, if the <address> field in the above example is equal to <address1> then the value of <a> is assigned to <mux_out>.
When this condition evaluates as false, the next condition in the sequence is evaluated.
We use the else keyword to separate the different conditions and assignments in our code.
The final else statement captures the instances when the address is a value other than those explicitly listed. We only use this if we haven't explicitly listed all possible combinations of the <address> field.
- When Else Mux Example
Let’s consider the simple four to one multiplexer again in order to give a practical example of the when else statement in VHDL. The output Q is set to one of the four inputs (A,B, C or D) based on the value of the addr signal. This is exactly the same as the previous example we used for the with select statement.
The VHDL code shown below implements this circuit using the when else statement.
- Comparison of Mux Modelling Techniques in VHDL
When we write VHDL code, the with select and when else statements perform the same function. In addition, we will get the same synthesis results from both statements in almost all cases.
In a purely technical sense, there is no major advantage to using one over the other. The choice of which one to use is often a purely stylistic choice.
When we use the with select statement, we can only use a single signal to determine which data will get assigned.
This is in contrast to the when else statements which can also include logical descriptors.
This means we can often write more succinct VHDL code by using the when else statement. This is especially true when we need to use a logic circuit to drive the address bits.
Let's consider the circuit shown below as an example.
To model this using a using a with select statement in VHDL, we would need to write code which specifically models the AND gate.
We must then include the output of this code in the with select statement which models the multiplexer.
The code snippet below shows this implementation.
Although this code would function as needed, using a when else statement would give us more succinct code. Whilst this will have no impact on the way the device works, it is good practice to write clear code. This help to make the design more maintainable for anyone who has to modify it in the future.
The VHDL code snippet below shows the same circuit implemented with a when else statement.
Instantiating Components in VHDL
Up until this point, we have shown how we can use the VHDL language to describe the behavior of circuits.
However, we can also connect a number of previously defined VHDL entity architecture pairs in order to build a more complex circuit.
This is similar to connecting electronic components in a physical circuit.
There are two methods we can use for this in VHDL – component instantiation and direct entity instantiation .
- VHDL Component Instantiation
When using component instantiation in VHDL, we must define a component before it is used.
We can either do this before the main code, in the same way we would declare a signal, or in a separate package.
VHDL packages are similar to headers or libraries in other programming languages and we discuss these in a later post.
When writing VHDL, we declare a component using the syntax shown below. The component name and the ports must match the names in the original entity.
After declaring our component, we can instantiate it within an architecture using the syntax shown below. The <instance_name> must be unique for every instantiation within an architecture.
In VHDL, we use a port map to connect the ports of our component to signals in our architecture.
The signals which we use in our VHDL port map, such as <signal_name1> in the example above, must be declared before they can be used.
As VHDL is a strongly typed language, the signals we use in the port map must also match the type of the port they connect to.
When we write VHDL code, we may also wish to leave some ports unconnected.
For example, we may have a component which models the behaviour of a JK flip flop . However, we only need to use the inverted output in our design meaning. Therefore, we do not want to connect the non-inverted output to a signal in our architecture.
We can use the open keyword to indicate that we don't make a connection to one of the ports.
However, we can only use the open VHDL keyword for outputs.
If we attempt to leave inputs to our components open, our VHDL compiler will raise an error.
- VHDL Direct Entity Instantiation
The second instantiation technique is known as direct entity instantiation.
Using this method we can directly connect the entity in a new design without declaring a component first.
The code snippet below shows how we use direct entity instantiation in VHDL.
As with the component instantiation technique, <instance_name> must be unique for each instantiation in an architecture.
There are two extra requirements for this type of instantiation. We must explicitly state the name of both the library and the architecture which we want to use. This is shown in the example above by the <library_name> and <architecture_name> labels.
Once the component is instantiated within a VHDL architecture, we use a port map to connect signals to the ports. We use the VHDL port map in the same way for both direct entity and component instantiation.
Which types can not be used with the VHDL logical operators?
Scalar types such as integer and real.
Write the code for a 4 input NAND gate
We can use two different types of statement to model multiplexors in VHDL, what are they?
The with select statement and the when else statement
Write the code for an 8 input multiplexor using both types of statement
Write the code to instantiate a two input AND component using both direct entity and component instantiation. Assume that the AND gate is compiled in the work library and the architecture is named rtl.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Table of Contents
Sign up free for exclusive content.
Don't Miss Out
We are about to launch exclusive video content. Sign up to hear about it first.
palomafg (Member) asked a question.
- Welcome And Join

eteam00 (Member)
palomafg (Member)

gszakacs (Member)

austin (Member)
Related Questions
Community Feedback?

IMAGES
VIDEO
COMMENTS
In this case your input is a and your output is a_out. If you want to make the delay longer, increase the size of a_store by resizing the signal declaration. If you want to access the intermediate signal for other reasons, you could do this: a_store <= a_store_registered(cycles_delayed-2 downto 0) & a; process(clk) begin. if rising_edge(clk) then.
constant dn: positive := 5; signal delay: std_ulogic_vector(0 to dn - 1); ... process (read_clk) begin if rising_edge(read_clk) then delay <= rd_en & delay(0 to dn - 2); end if; end process; rd_en_d5 <= delay(dn - 1); ... Delay a signal in vhdl. 1. testbench help to make one clock cycle delay. 0. Generate a clock delay by fixed cycles number ...
VHDL signal assignment statements prescribe an amount of time that must transpire before a signal assumes its new value. This prescribed delay can be in one of three forms: Transport: propagation delay only. Inertial: minimum input pulse width and propagation delay. Delta: the default if no delay time is explicitly specified.
This section explains how VHDL can be used to model time delays to obtain a timing simulation. We will discuss two models of delay that are used in VHDL. The first is called the inertial delay model. The inertial delay model is specified by adding an after clause to the signal assignment statement. For example, suppose that a change on the ...
In VHDL the designer has the possibility to perform a signal assignment after certain amount of time, implementing the delay in the assignment. VHDL Delay Type. In VHDL there are two different kind of delay. Transport delay; Inertial delay; VHDL Delay Model. The transport delay is used to model the delay introduced by wire connection or a PCB ...
Delay Types. Delay Types. All VHDL signal assignment statements prescribe an amount of time that must transpire before the signal assumes its new value. This prescribed delay can be in one of three forms: Transport -- prescribes propagation delay only. Inertial -- prescribes propagation delay and minimum input pulse width.
A Signal assignment statement can appear inside a process (sequential statement) or directly in an architecture (concurrent statement). The target signal can be either a name (simple, selected, indexed, or slice) or an aggregate. A signal assignment with no delay (or zero delay) will cause an event after delta delay, which means that the event ...
Yes. If a signal assignment with delay is made to a signal that is also used as an input in a separate concurrent signal assignment, then the delay will propagate through both assignments. B) No. Only the assignment in which the delay is used will experience the delay. Summary. VHDL operators are defined to work on specific data types.
In VHDL-93, any signal assignment statement may have an optional label: label: signal_name <= expression; A delayed signal assignment with inertial delay may be explicitly preceded by the keyword inertial. It may also have a reject time specified. This is the minimum "pulse width" to be propagated, if different from the inertial delay: ...
As we see later signal assignment with its associated delay and simple variable assignment use different symbols ( <= and =) ... Section 3 - The Delay Model. One way that a VHDL simulator can treat time delays is called the inertial delay model. The inertial delay model is specified by adding an after clause to the signal assignment statement.
The VHDL Simulation Cycle Initialization Phase 1. The effective value of each declared signal is computed, and the current value of the signal is set to this effective value. The value is assumed to have been the value of the signal for an infinite length of time prior to the start of simulation. 2. The value of each implicit signal of the form
Signal assignment statement 3. Variables are cheaper to implement in VHDL simulation since the evaluation of drivers is not needed. They require less memory. 4. Signals communicate among concurrent statements. Ports declared in the entity are signals. Subprogram arguments can be signals or variables. 5. A signal is used to indicate an ...
Conditional Signal Assignment or the "When/Else" Statement. The "when/else" statement is another way to describe the concurrent signal assignments similar to those in Examples 1 and 2. Since the syntax of this type of signal assignment is quite descriptive, let's first see the VHDL code of a one-bit 4-to-1 multiplexer using the ...
Signal A is assigned the result of the function my_function by the inertial delay model. In the first example this happens after 5ns; in the second and third example this happens after a time which is determined by the constant delay. In the third example the value 0 is transferred to A 2ns after the preceding assignment.
3.4 Design a VHDL model for a combinational logic circuit using selected signal assignments. Design a VHDL model for a combinational logic circuit that contains delay. 3.1 VHDL Operators There are a variety of pre-defined operators in the IEEE standard package. It is important to note that operators are defined to work on specific data types ...
Delta Delay. Default signal assignment propagation delay if no delay is explicitly prescribed VHDL signal assignments do not take place immediately Delta is an infinitesimal VHDL time unit so that all signal assignments can result in signals assuming their values at a future time E.g. Supports a model of concurrent VHDL process execution
The VHDL code shown below uses one of the logical operators to implement this basic circuit. and_out <= a and b; Although this code is simple, there are a couple of important concepts to consider. The first of these is the VHDL assignment operator (<=) which must be used for all signals.
simulation. This is equivalent to direct assignments. The fact that there is other. code in the process has no bearing on the behavior of these signals. This is not. a microprocessor that runs sequentially through a set of instructions. Unless. there is an event trigger, then everything in the process happens at once - i.e. no delay.-- Gabor
1. The sensitivity list does not contain all the signals that are used in the process, since the address is a signal (assigned in the same process, but that does not matter) but the signal is not in the sensitivity list of the process. So the process is not reexecuted by the simulator when the value of address changes.
1. Careful with your terminology. When you say a changed in the other "process", that has a specific meaning in VHDL (process is a keyword in VHDL), and your code does not have any processes. Synthesizers will treat your code as: a <= c and d; b <= (c and d) and c; Simulators will typically assign a in a first pass, then assign b on a second ...
The effect of executing any line with a signal assignment in it is, if the value of that signal is to change, to schedule that change for sometime in the future. If no delay is specified (which is the case here), then that future time is the next iteration of the simulation, which will occur once all the processes have finished executing (have ...